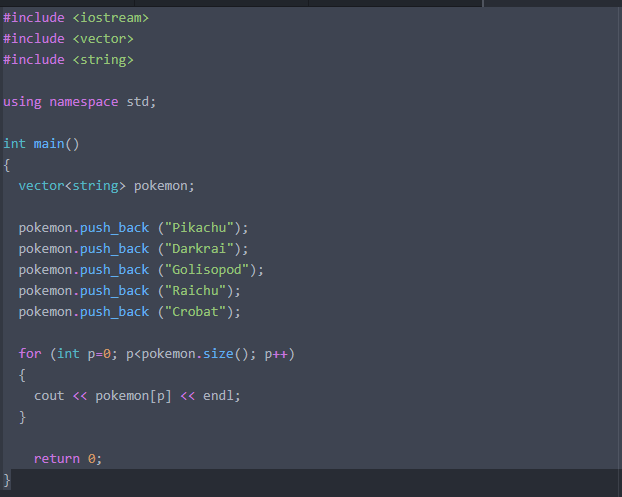
Using Vectors In C++
The Vector container in C++ is very useful. It will be the better option when you want to store data in an array. Vector has several advantages over a traditional array structure. This makes it more flexible.
Introduction
The Vector container originates from the STL(standard template library). It is one of the C++ essentials you will need to learn well. The STL has many such data structures and algorithms built in. A Vector array can hold a sequence of data. This data is stored in memory next to each other. You can access its elements like you would in a traditional array.
A Vector container has advantages over the traditional, built in array. You can easily find out the current size of your array with member functions. A Vector will grow as needed. It can be full of data but will add an extra element at the end if it is needed. Vectors do not have to be a certain size when created.
You need to use a Vector container if your data changes regularly. It will save you lots of headaches. It is a good way to handle input and output. A Vector will also make your program more efficient. We should all strive for this.
In C++ we have the <string> class which makes text much easier to work with. It is a vast improvement over the C language use of char’s.
Creating and using a Vector is easy to do. You must include its header file.
#include <vector>
Defining a Vector container is slightly different than a regular array. It looks like this:
vector<string> PokemonSets;
vector<int> number;
There is no space between the data type and the word “vector”. You will not need to declare a size because it expands as needed. However, you can include a starting size. This is good because it makes this container really flexible. You will need to specify the data type like <int> and a name for your Vector like <number>.
vector<double> LargeNumber(5, 5);
In this example, “LargeNumber” is created with 5 elements and all of them are initialized to 5.
You can use all the typical data types with a Vector. Float, int, char, string and double are all valid. Let us now start with some basic examples so you can see how this works. I will start with the <push_back> c++ function to add elements. The <push_back> function is an example of a modifier. Use it to modify the contents of your Vector.
Adding Elements
This first example used the <push_back> member function to add values to the Vector. This function adds a value to the end of a Vector or it makes it the first value. If it is full, then it creates empty space at the end.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main()
{
vector<string> pokemon;
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
return 0;
}
I defined my vector as a <string> and then named it. I then usesd the <push_back> function for each element that I wanted to add to the array. Then I constructed a <for loop> to run through each element and print it to the screen.
Getting the Size
We can determine the size of a Vector easily. If the vector contains hundreds of elements, it is much easier to use the <size()> function. Let us modify our first example to give us more information.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main()
{
vector<string> pokemon;
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
int num = pokemon.size();
cout << endl;
cout << "The size of this Vector is : " << num;
return 0;
}
Here I use the <size()> function to let me know how large the Vector is. You add the <size()> function to the end of yourn Vector name. This associates the function with the Vector you want to work on. Set up a variable like <num> so you can print the value to the screen.
Removing Elements
Sometimes you will want to reduce the size of your Vector. You will want to do this if you have old data, for example. To do this, you use the <pop_back> function.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Main function
int main()
{
vector<string> pokemon;
// Adding elements to the Vector
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
cout <<"Printing the original list of pokemon" << endl;
cout << endl;
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
int num = pokemon.size();
cout << endl;
cout << "The size of this Vector is : " << num << " pokemon" << endl;
cout << endl;
// removing the last value from the vector
pokemon.pop_back();
cout << endl;
cout <<"Printing the list for the 2nd time" << endl;
cout << endl;
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
int num2 = pokemon.size();
cout << endl;
cout << "The size of this Vector is now : " << num2 << " pokemon" << endl;
cout << endl;
// Adding the element again to the end
pokemon.push_back ("Crobat");
cout <<"Printing list for the 3rd time " << endl;
cout << endl;
// Checking and displaying size again
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
cout << endl;
int num3 = pokemon.size();
cout << "The size of this Vector is again : " << num3 << " pokemon" << endl;
cout << endl;
return 0;
}
This example is a continuation of the first two programs. I create my Vector and name it appropriately. Again, I add my values as elements and print to the screen. Now I use the <pop_back> function to remove the last element of the array. I loop my way through the elements again so I have an accurate count. Then I print the new total of pokemon I have in my Vector.
The next step is adding my lost <Crobat> back to the array again. Can't let the poor fellow be all himself. After I use the <push_back> function again he is reunited with his friends. I repeat the same steps as before. I loop my way through the elements again to get the correct total. I print to the screen again.
Emptying A Vector
There could be times when you want to completely clear a vector. It is done with the <clear()> function.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Main function
int main()
{
vector<string> pokemon;
// Adding elements to the Vector
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
cout <<"Printing the original list of pokemon" << endl;
cout << endl;
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
int num = pokemon.size();
cout << endl;
cout << "The size of this Vector is : " << num << " pokemon" << endl;
cout << endl;
// Clearing the vector
pokemon.clear();
cout << endl;
cout <<"Printing the list for the 2nd time" << endl;
cout << endl;
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
int num2 = pokemon.size();
cout << endl;
cout << "The size of this Vector is now : " << num2 << " pokemon" << endl;
cout << endl;
// Repopulating the elements
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
cout <<"Printing list for the 3rd time " << endl;
cout << endl;
// Checking and displaying size again
for (int p=0; p<pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
cout << endl;
int num3 = pokemon.size();
cout << "The size of this Vector is again : " << num3 << " pokemon" << endl;
cout << endl;
return 0;
}
I create my Vector and name it. I add my elements and print them to the screen. Afterwards I use the <clear()> function to empty the contents of the array. When I print again it is just zero in my list. There is nothign there. So I have to add them all back in again. After I do that I loop through the elements and once again print the entire list to the screen.
Reading From A File Into A Vector
Now lets do a more interesting example. I want to read data from a file. This is a task that you will do quite often as it is more useful. Since there is only data, there are no decisions to make. To keep with the theme of pokemon, I created a text file in the same directory I am working out of with my editor. In this text file are ten names of pokemon, and each is on a separate line. This program will read the file line by line and print what it finds to the screen. Remember to be in the same directory or you have to enter the full path to your file.
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
int main()
{
// variables
vector<string> pokemon;
ifstream PokemonFile;
string str;
// opening file to read from
PokemonFile.open("PokemonFile.txt");
// reading from file into the array
while (PokemonFile >> str)
pokemon.push_back(str);
// closing our file
PokemonFile.close();
// printing array values
for (int p = 0; p < pokemon.size(); p++)
{
cout << pokemon[p] << endl;
}
cout << endl;
return 0;
}
I created my Vector and named it accordingly. To read from a file, though, you have to do a little more set up. You need to create a variable to work with the <fstream> objects. The next task is to open up your file. I named mine <PokemonFile.txt>. That is so I won't get confused, which happens easily I admit. All the more reason to name variables in meaningful ways. It really does help.
Next I create a loop to read each line of the file. As it reads each line, it will add them to the elements of the Vector array. Close the file and then just print your Vector and its elements.
We got the expected output, which is nice. You can keep adding to the file and saving it. Each time you run this program, it will read the current contents of the file.
In these examples I used all strings. Strings are harder to deal with but it is easy to find examples dealing with numbers. That is why I did it like this. However, if you have numbers in your file, you can add them to the Vector array and do all kinds of math on them. That is very useful as well.
Conclusion
In this section we learned about the Vector container. Creating a Vector and getting data into are pretty easy. Just don’t get confused by the different syntax from a regular array. We covered adding elements, removing elements, getting its size, emptying it out, and reading data from a file.