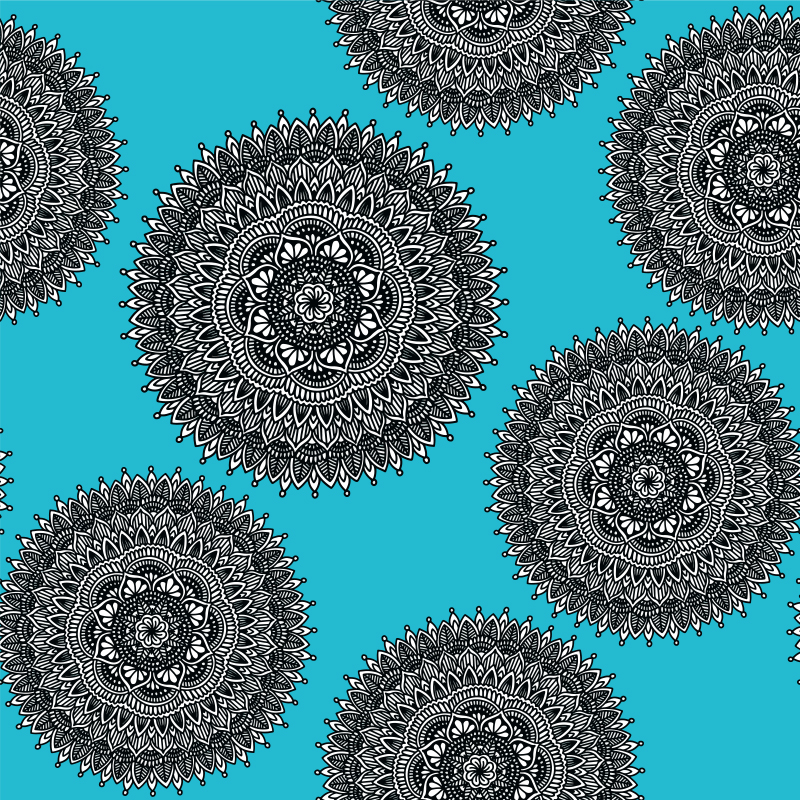
Using Variables in C++
Variables are storage containers in your computer’s memory and they hold the information that you work with.
Introduction
In C++, we work with information. Sometimes, that information changes. Therefore, we need variables. Variables let us change the information that we work with. They allow you to store and work with information. Variables let us identify the information that we are working on. Once identified, we can change or manipulate it as we need to.
#include <iostream>
int main()
{
int var = 1000000000;
std::cout << var << std::endl;
std::cin.get();
return 0;
}
When we create a variable, we store it in memory. So, you can also say that it stored our information in memory. This is because a variable is a type of container that stores things. This is one of your tasks as a programmer, to determine how many and what size variables you need in a program.
Data Types
Variables can store different information. We call these data types in C++. The differences in data types are mainly about size. The size distinguishes the different types.
Here are some common data types:
int
char
string
float
double
bool
The 'int' data type, for example, can be signed or unsigned. A signed data type means it can be positive or negative. Each numerical data type will have a maximum value that it can hold. The maximum value is directly related to how much information that it can hold.
An unsigned data type, like an integer, can hold a number at twice the value of a signed integer. This is because, if there are no negative numbers, then the variable can be all positive and therefore, twice the value.
Data types can be changed too. You can use short, unsigned, or long to optimize a variable. For example, if you need to store a value of 3 billion, then use an unsigned integer as your data type. You would do this because it uses less memory than a regular integer.
Modifying Data types
Saving memory and being efficient becomes important later on. It is something you want to keep in mind. That is why modifiers like short, unsigned, and long exist. They are there to help you make the most of the memory you have to work with.
The size of data types are dependent on the compiler you use. There are slight differences between compilers, so it is important to know about the compiler that you are using.
We can find out the size of our data types with the “sizeof” command. You should do this every time you use a new compiler or machine, so you can plan your memory usage accordingly. We will look at this function at the end of this article.
Use unsigned data types when you know your program won’t have negative values. This lets you have larger values in your variable without having to use a float or a double, which uses more memory.
Declaring Variables
When you declare a variable, it is called a variable definition. This definition lists the variable’s name to the compiler and says what data it will hold. There must be a definition for every variable in your program. These definitions are a statement and must have a semicolon at the end of the statement.
// This is a variable definition and assignment
int num = 15;
When you give a variable a value, it is an assignment. The equal sign copies the value on the right into the variable on the left. When information is stored in a variable, the entire operation is an assignment statement.
When a value is copied into a variable, the ‘=’ is used to do this. We call this the assignment operator. Operators work on our information. The information that it works on is called the operands.
When copying values to variables, the variable has to be on the left side of the operator. You can also assign value when you declare the variable. We call this initializing the variable.
You can declare many variables at once. So, instead of using a separate line for each variable, you can declare several at once if they are the same data type. It’s also less typing, which can be important too.
// Declaring multiple variables with one statement
int age, weight;
double mass, distance;
You can put them anywhere in your program as long as it is before you try to use them. However, I think it’s best to be organized and have a variable section for each program. It makes your code easier to read.
Naming Variables
Naming variables is an important task. It does not take much effort, but it should be done with some thought. You want it to be short but meaningful to a stranger who has never seen your code before. That is what you are trying to do.
When you name variables, it can’t be a C++ keyword. The first character must be a letter or an underscore. After the first character, you do also use numbers as well as letters and an underscore. Variables are case sensitive so you will need to pay attention to that.
Integers
Integer data types only hold whole numbers. When selecting a data type for your variable, you have a few things to think about. You want to consider the largest and smallest value that this variable is supposed to hold. Know how much memory the variable will use.
// Assigning an integer value
int book_amount = 1700;
int number_shelves = 6;
Think about whether the variable can be unsigned or should it remain signed. Last, you need to know how much precision the variable must account for.
// Using an unsigned integer value
unsigned int age = 22;
// Age will never be negative so this is a good use for an unsigned integer
Here are some examples of numeric modifiers:
short = 2 bytes
unsigned short = 2 bytes
int = 4 bytes
unsigned int = 4 bytes
long = 4 bytes
unsigned long = 4 bytes
#include <iostream>
int main()
{
float length = 20.3;
float width = 23.8;
float area = length*width;
std::cout << area << std::endl;
return 0;
}
The long modifier is a special case. On some older systems, long is needed in tandem with the integer data type. I think this would be a pretty old system but I didn’t want to discount it because other parts of the world may use that type of equipment.
Char Data Type
This is the character data type, and it uses 1 byte of memory. We mostly used it for storing characters, however, that can really be anything. This is because characters are really just numbers in the background.
// Declaring a char data type variable
char letter = 'A';
Most characters these days are encoded using ASCII. There are older formats also but they are not really in use too much these days. This means that ‘A’ has a certain ASCII number and so does ‘a’. Case matters and different cases of the same letter have different numbers.
So, when a character is assigned to a variable, it is the number that represents the character that is really stored.
When assigning char values, you use single quotes around the value. An example would be ‘A’ or ‘b’.
#include <iostream>
int main()
{
char letter;
letter = 75;
std::cout << letter << std::endl;
letter = 76;
std::cout << letter << std::endl;
return 0;
}
Char variables can’t hold a string but there are ways around that. Typically, you use a char array. So, it is possible. However, it’s probably just better to use an actual string. I will show you those soon.
Floating-Point Data Type
Floating-points are a data type that are used to hold real numbers. Real numbers, if you remember from set theory, can have a decimal. Decimals are an important part of many programs and operations.
// Declaring a floating-point variable
const float pi = 3.14
We can further divide this data type up and each has their uses.
floats = 4 bytes
double = 8 bytes
long double = 8 bytes
#include <iostream>
int main()
{
float height, width, wall;
std::cout << "Please enter dimensions of your wall in meters " << std::endl;
std::cout << "Enter your height in first and then your width" << std::endl;
std::cin >> height;
std::cin >> width;
wall = height*width;
std::cout << "Your wall takes up this much space: " << std::endl;
std::cout << wall << " meters squared ";
return 0;
}
The actual size of these data types on your computer will need to be determined. Usually, though, float is the smallest and long double will hold the largest values. There are also no unsigned floating-point data types. That means they can all be positive or negative.
Bool Data Type
The bool data type is either false or true. To the computer, that means 0 or 1. We call these boolean expressions. They allow you to create small variables that hold true or false values.
// Declaring a boolean variable
bool tf;
Boolean operations are great for logic problems. You can get very creative with them and that is where they show their flexibility.
#include <iostream>
int main()
{
bool answer;
answer = false;
std::cout << answer << std::endl;
answer = true;
std::cout << answer << std::endl;
return 0;
}
Data Type Size
You should determine the size of a particular data type on your machine. This is because machines and compilers all vary slightly and this is something you want to know for sure.
We accomplished this by using the ‘sizeof’ function. It will report the number of bytes used by a data type or variable.
#include <iostream>
int main()
{
int wall;
float area;
double pi;
std::cout << "The size of data types will be given in bytes" << std::endl;
std::cout << std::endl;
std::cout << "An integer is " << sizeof(int) << std::endl;
std::cout << "A float is " << sizeof(float) << std::endl;
std::cout << "A double is " << sizeof(double) << std::endl;
return 0;
}
Conclusion
Variables are a simple part to learn in C++ but they are essential to get right. In this article we talked about what they are used for, declaring them, modifying them, and the different data types we can use. Finally, I showed you how to find the memory each data type takes up on your machine.
Thanks For Reading
Thank you for reading this, I really appreciate it.
If you would like to join my newsletter, you can do so here.
If you would like to read other articles I write then try here: