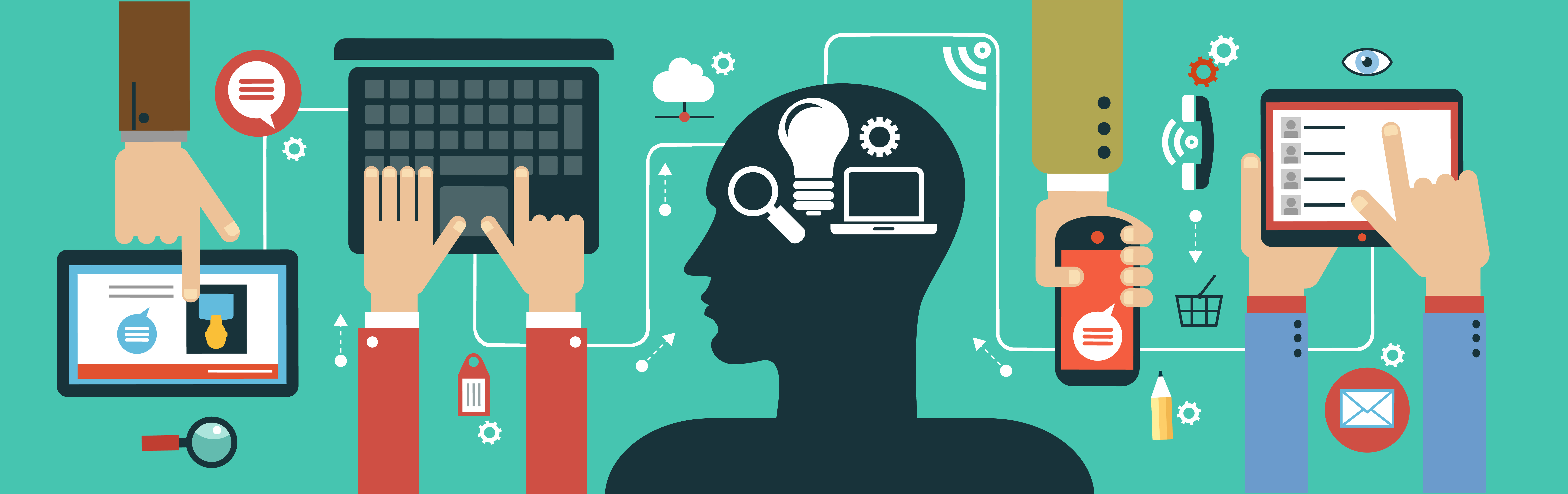
Using Functions in R Programming
In this guide today, I want to talk about some of the basics of R programming and keeping your code organized.
Introduction
If you want to follow along in this sectional load op your R editor or Rstudio. It will be fun. Start with checking your version and see if you need to update.
R.Version()
Vectorized Functions
You will use functions more than anything else. Almost everything you do will involve one. Let us start with vectorizing your functions. This type of function works on a whole set of values at once. A vectorized function takes things a step forward. You do not have to look at each element singly. A vectorized function will do this all at once for you. To make a vectorized function, use the “c()” function. It stands for combine and it combines stuff inside the parentheses.
I play a game called EverQuest so I will use some data from it. Specifically, my rogue does damage and the individual hits are going to be the arguments of the “c()” function.
damage_per_second = c(23453, 115200, 67441, 110688, 220665)
We have our data in a variable. Let us see what we can do. The total is the first property to come to mind.
sum(damage_per_second)
This gives us our total damage done to some deserving digital monster in our game. We can also work with string data. A good example would be the name of the monster. Let us call it a goblin berserker.
Monster_name = c(“Goblin”, “Berserker”)
So, we have created another vector with the first and last name of this kind of goblin. We can use the “paste()” function to copy it back in any order.
Paste (monster_name)
This function will look at each item and concatenate them together. It will do something else very cool. What if we did not want to type “goblin” every time because we are surveying a whole clan of goblins and we have a few hundred types? I won’t type all of those but I will do a few to show you how this works.
monster_first_name = c("goblin")
monster_last_name = c("berserker", "extremist", "digger")
paste (monster_first_name, monster_last_name)
Now hit enter after each statement and do you see what happens? We have the word “goblin” in front of each of the types. I think that is so cool. These are vectorized functions!
Function Arguments
We have just used a couple functions. Remember the data we put into the parentheses? Those are arguments for the function. The function is waiting for something to work on, whether it is numbers or names or whatever. There is more to arguments than this, but for now, let’s keep it simple. We will come back to function arguments later when we need to.
Command History
R keeps track of your commands. You can look at your past commands by using the up and down arrows in your IDE. So, you just hit up, for example, and then select “enter” when you see the command you want to execute. You can also use a function called “savehistory()” to save your commands to a file. If you do not give it any arguments between the parentheses, it saves the commands in a file called .Rhistory in your working directory. However, you can give arguments to the function to give it a different name.
savehistory(file = “functions.Rhistory”)
This will let you see specific commands when learning about functions!
Comments
I don’t think I have talked about comments in this section, yet. So, let’s do that. If you have written code before, you know the importance of comments in your code. Your code should be self documenting so you can easily see what the code does. However, you need comments to tell you why you did it that way. I also use comments in my code to divide it into sections. That way it is easier for me and others to see what and why I did something.
# This is a comment and it should explain something about your code.
Everything after a hash mark is ignored on a line.