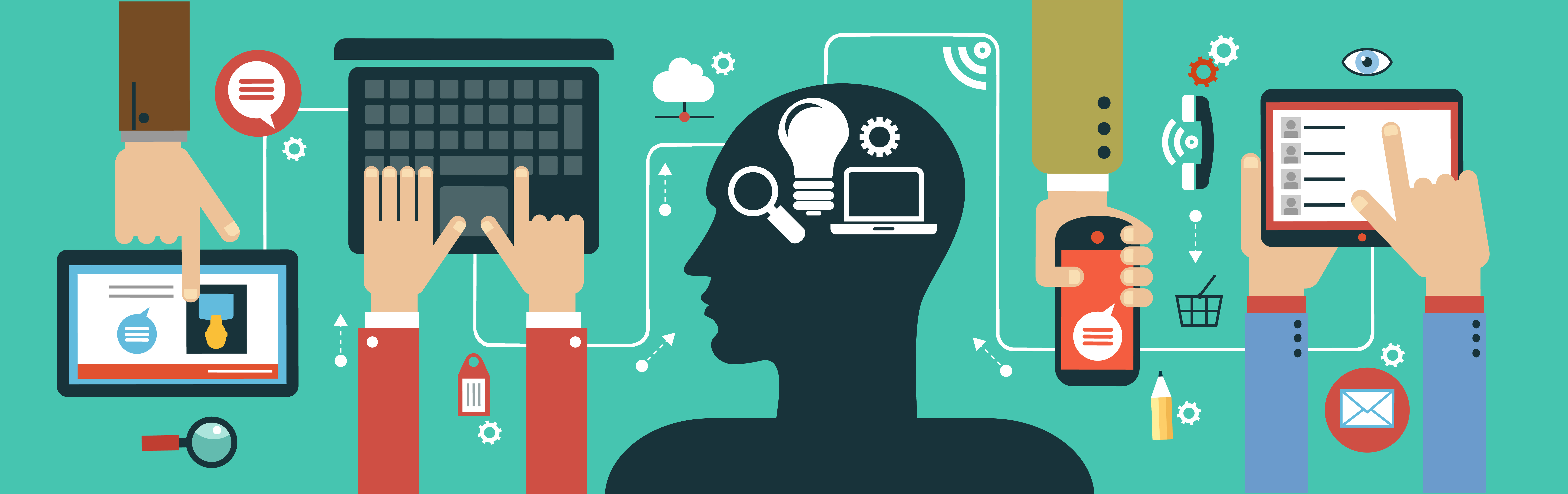
Vectorizing Your Functions In R
These are my notes and thoughts on vectorizing your functions in R.
Vectorized functions are very useful in R programming. They are a way to analyze data quickly and easily. I briefly mentioned them in the section before this but today I want to go more in depth.
Vectorizing Your Functions
Your first step in using vectorized functions is to create a vector of values. Let us make a vector using the high temperatures of Cincinnati, OH for the next few days. I don’t live there, but the temperatures are so nice, I wish I did. We need to make a vector name and then use the c() function. It looks like this:
Temps = c()
Now I will use my weather program to find the highs for the next few days and input those into the function. Use commas to separate values.
Temps = c(77,79,79,82,68,72)
With these values in hand, we can always call the vector name to see them again. The values could change, that is why it could be useful. Do it like this:
Temps
We could get the sum of the temperatures if we wanted, though with temperatures that is not exactly useful. However, you should know how to use the sum function for other kinds of numerical data.
sum(temps)
That will give you a sum of values.
We can also use functions that deal with string data or names, then work on that function with another function.
Let me use some Magic the Gathering cards I have for examples.
What I will do here is list the first part of the card name for a few different cards. Then I will do the same for the last part of the card name. Lastly, I will paste the second part to the first part.
first=c(“Annointed”, “Shivan”, “Llanowar”)
second=c(“Peacekeeper”, “Reef”, “Wastes”)
Now we can use the paste() function on our vector.
paste(first, second)
Those are the actual names of the cards I am looking at. The point is that you can use functions to work on other functions.
Arguments
Most functions will allow you to use arguments. They let you tell the function exactly how to behave. This is called passing a value to a function. If you know other programming languages, this is not a foreign concept. Most functions across languages work like this. You can have arguments with default values and some without default values.
Command History
R keeps track of all the commands you use in a particular session. The purpose of this functionality is to let you see what you have done and let you easily repeat something. You can look at the history that you have typed by using the up arrow within your console. You can hit enter when you see something you want to repeat. This runs the same command again.
You can save the history of your commands with the “savehistory()” function. This will automatically save them in a file called .Rhistory, but if you want to specify a different file you can do this:
savehistory(file=”modernmagic.Rhistory”)
You can look at the file with any text editor. You can also load a previous history file.
loadhistory(“legacymagic.Rhistory”)
Adding Comments
Comments are good to use, they help with readability. You can use them to indicate who wrote a piece of code and what they were intending. It is also good to explain why something is there in the first place. This is done with the pound symbol.
# this line is a comment and does nothing else
You can place comments at the beginning of a line or any place in the line itself. To do multiple lines of comments, every line will need a pound symbol.
Packages
Anyone can write functions in R and share them with others. These are called packages. There are a few different website repositories that contain collections of these packages. The most important is: https://cran.r-project.org
You install packages by using the command:
install.packages()
The name of the package is the argument of the function.
install.packages(“magic”)
Once the package is installed, you have to load it in order to use it. You do it like this:
library(“magic”)
After this step, you can officially use the commands of this package. The library is the directory where your packages are installed.