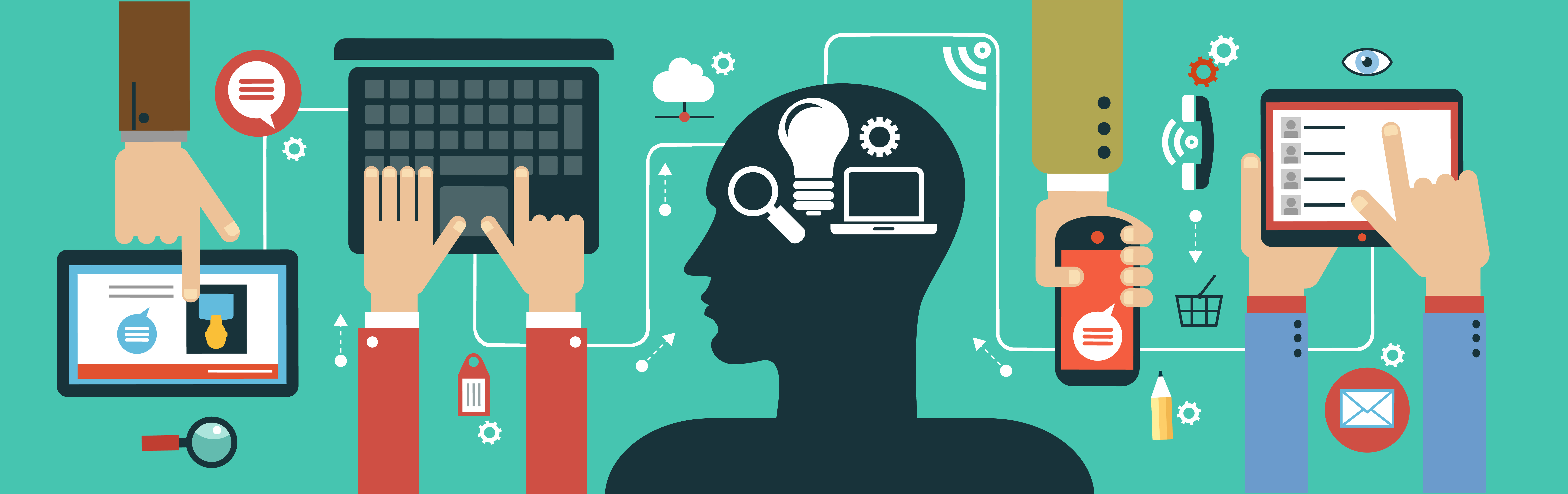
Web Development Explained
Almost everyone uses the internet. We use it for fun, to do work, and so on. In order for us to do all of these things, we rely on web pages. There are many things that work together to show us a web page.
Table of Contents
Web Applications
Web applications depend on clients and servers. Clients are your phones,laptops, desktops, and tablets. They use a web browser, like Chrome or Firefox, to access the web pages on a web server. It is called a web server because the files are on a server, which is another computer, that gives access to those websites it wants to share.
A static web page is one that does not change unless its developer changes it. It shows content that is static. It comes directly from the web server, as is, to your browser. When you click a link to go to a website, your browser builds a request for that page. This is called an HTTP request and uses the rules of the HTTP protocol.
When the web server receives this HTTP request, it will show you the file that resides on its data drive. This file will contain some form of web instructions, usually HTML. The web server will send the file back to the client’s browser as an HTTP response. When the browser receives the HTTP response, it translates the file into the web page you are looking for. Static web page files have an .html or.htm extension.
Web pages can also be dynamic. This means they change or are built every time they are requested. This allows for a lot of customization. A dynamic web page is built by a program or script. When your browser contacts the web server, the program or script starts and does what it is programmed to do. A cool feature of a dynamic web page is that it can use the data entered by the user to build the web page and show it to the user.
Dynamic web pages let you create interactive applications. This is what makes them so powerful.
When developing websites, it is important to test on many browsers. The reason for this is that they all do things differently.
Javascript can also be used for web page processing. This is done on the client side, though. This is one of the features that make Javascript useful to learn at a later date. It helps applications run quicker because its code does not have to travel to the web server and back.
HTML
To start developing web pages, you use HTMl to create the page and CSS to format everything.
The HTMl language is what you use to create a web page. It starts with a Doctype declaration and specific tags to define its structure. Tags are opened and then closed. For example,
<html> content </html>
All tags follow this basic structure. HTML documents have a head and a body. The head section tells you about the document itself. The body section contains everything that is displayed in the web browser.
A basic HTMl document looks like this:
<!Doctype html>
<html lang=”en”>
<head>
<meta charset=”utf-8”>
<title> Starting your HTML Journey </title>
</head>
<body>
<h1> Learning to Code </h1>
<img src=”mainpage.png” alt=”Coding Course”>
<p> Learning to code is fun. </p>
</body>
</html>
That is basic but should be a functional site. Put that into your text editor and give it an .html extension. Open with your browser of choice or whatever your default is.
CSS
CSS, or custom style sheets, is used to format html documents. Style sheets are just files. They exist alongside your html files. However, the css files modify the html files. They provide the formatting. You provide an html link to the css file you created. You do it by using the title and path in the html file so it knows where to look. This link goes in the head of the html document.
CSS extends the capabilities of html. That is why it is used. So, now we can link to a css file like this:
<!Doctype html>
<html lang=”en”>
<head>
<meta charset=”utf-8”>
<title> Starting your HTML Journey </title>
<link rel=”stylesheet” href=”coding.css”>
</head>
<body>
<h1> Learning to Code </h1>
<img src=”mainpage.png” alt=”Coding Course”>
<p> Learning to code is fun. </p>
</body>
</html>
Now, our html document will look at the coding.css file to see what it says to do.
When working with html and css files,. You can use a text editor or a web IDE. Every operating system has popular ones.
Once you have web hosting, you should use a FTP program to upload your files to your web server. Again, this is operating system dependent.
Viewing Source Code
For most websites, you can see its source code. You can do this through your browser menu pretty easily. It is some combination of view-page-source. While every browser is a little different, it should be something similar.
You can also right click on a page and select source. Then, in some browser menus, you can select the css files to view. The code is often opened up in another window.
Development Issues
There are a few issues to keep in mind when writing HTML. These are cross-browser compatibility, accessibility, and search engine optimization.
For compatibility, you want to make sure as many browsers as possible can use your web pages. Testing is important. Make sure to test older versions of browsers as much as possible. Try to use the core HTML and CSS features as much as possible. Doing this will let your code run on as many browsers as possible.
Accessibility is also very important. It is important to make a site accessible to everyone. The idea is to provide alternate versions of your website information so that impaired people can understand what is happening.
Finally, there is search engine optimization. The goal is to make your website appear higher in search rankings. The higher it is ranked, the more people that will see it. This is very important if it is an e-commerce site, for example. There are specific ways to code that will improve your search engine optimization. These techniques will be noted when we get to them.