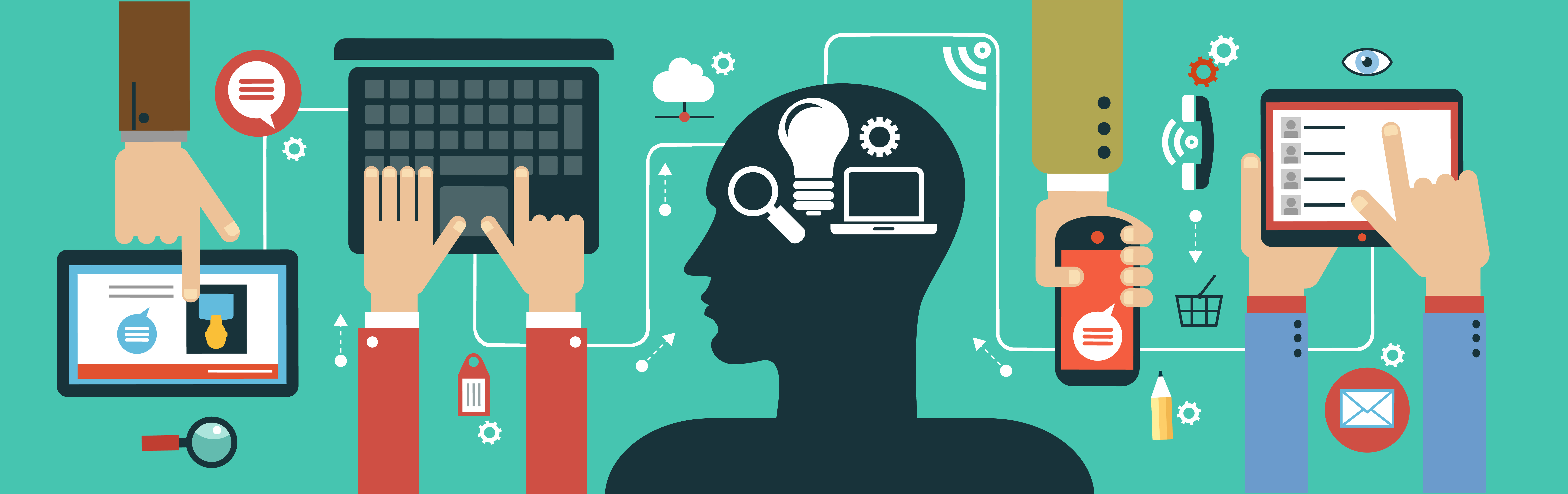
C++ Basics
This is my guide for people who want to know how C++ works. With a little effort it can be easy to pick up. I cover the essential basics here with examples.
This is my favorite C++ book on Amazon, if you are interested in learning C++ I highly recommend it
Table of Contents
Getting Started With C++
C++ is very popular and has been around a long time. It has had several different versions. Every 3-4 years or so a new version gets released.
It is very fast. It is fast because it is a lower level language. Often it is the backbone of other program languages and major applications. Operating systems, games, and compilers use C++ as their foundation.
Use A Text Editor
A text editor is the best way to get started in learning C++. The reason why is that it is simple to set up and use. You can use an IDE, which stands for integrated development environment, but that just complicates things. So use an editor that you like and are comfortable with.
Popular editors include Vim, Nano, Emacs, Atom, Sublime Text, and VsCode. There are many others but you can’t go wrong with any of those. It will also depend on what operating system you use. I use both Linux and Windows. When I am on Linux I like Vim but when I am on Windows I prefer Atom.
Installing Compiler
You only need to do this if you are using Windows. If that is the case you want to install MinGW-64 and add it to your path. When installing, leave all the defaults. After it is installed, it is time to add it to your path variable in Windows. Browse your installation folder until you get to the "bin" folder. That whole path is what you want to add.
Here is a video I found that shows you, in case you get confused.
If you use Linux, it already comes with a compiler. You do not have to do anything. If you use Linux, I am automaticaly assuming you know how to use a terminal and run things. Just run "g++" and you can use the help system if you need to know options.
Compiling Your Program
Whichever operating system you are on, it is helpful to know what a compiler does. A compiler translates your c++ code into an executable. Indeed, every time you edit your code from your text editor, you have to re-compile your code.
After you write your code, you have to name the file with a ".cpp" extension. This indicates, to the compiler, that it is a c++ program.
First Program
Lets make the typical first program, so we can start this ball rolling! Type this in your editor:
#include <iostream>
int main()
{
std::cout << “I love pokemon!!” << '\n';
return 0;
}
Name this something simple, like "pokemon.cpp". Then, in your terminal, navigate to the directory where you saved it at. You can list the files in this directories, you should see your file there. Then, from the command line, you can run:
g++ -o pokemon pokemon.cpp
This is how you compile your code.
Then type “pokemon” to run your program.
This will give your the console output of “I love pokemon”.
On linux, you compile the same way but you need to run the program differently. Type:
./pokemon
This will then run your code and give you the same output.
Structure Of A Program
C++ programs aren't complicated at first. However, there are a few parts to them that you need to know about. Every program is made up of:
- header file(s)
- main function
A header file is a library. It contains multiple namespaces and other code that allow the c++ programmer to use. You will encounter other functions that need a certain header file in order to work. When that happens, you use it in an "#include" statement.
The namespace is a section of our <iostream> header file. It contains certain functions and code. The one we are using is the "std" namespace. It stands for "standard". You shouod manually designate the namespace for a command.
For instance, if you dont want to include a header file, you can just define the namespace for any command that needs it.
Next is the main function. Every program has one. A program can have many functions but it has to have the main one at the very least. A function is a block of code that does something. It can output a line of text to the console or call a series of functions.
The "int" in front of main means integer. This defines the main function. It says the main function will return an integer. Indeed, the last line of the function says "return 0". So, it returned an integer and all is good. A lot of functions return values. The kind of value it returns is determined by the programmer.
Every C++ program has to have a "main" function. Everything inside of the "main" function is executed in sequential order.
Now, if we have this line:
cout << "I love pokemon" << '\n';
You will notice the semicolon at the end. This has to be done at the end of every c++ statement. It tells the compiler that this is the end of a statement.
Let us go over some more code now to see all this in action again. Specifically, I want to show you how to deal with lines.
#include <iostream>
int main()
{
std::cout << "darkrai and vileplume are my favorite pokemon" << '\n';
std::cout << " charizard is pretty cool too" << '\n';
return 0;
}
In this small snippet, you can see I wrote two lines. If I want them to appear on two separate lines in the output, I have to use "\n" or "std::endl". This means to end the line and go to the next, in the output. You put a stream insertion operator "<<" in front of it. The "std::endl" flushes the buffer and is slower than using "\n".
You can also use the escape character sequence to go to the next line. Escape sequences do others things too, depending on which you use. You will see more in the future. For now though, just know that you can use "\n" to skip a line too.
There are a few minor differences between "\n" and "std::endl" but at this stage they do the same thing. You use "\n" like this:
#include <iostream>
int main()
{
std::cout << "I listen to music, too \n";
std::cout << "In fact, I like the groups: Big Bang, SuperJunior, and 2pm " << '\n';
return 0;
}
The next thing I want to mention is comments. Comments are statements or notes to someone that are included in your code. This is often done by developers who want to explain what they are doing in sections of their code. Let me give an example.
// for std::cout and std::cin
#include <iostream>
int main()
{
// sending text to console
std::cout << "I miss playing the pokemon TCG" << '\n';
return 0;
}
The highlighted section above is a comment. It starts with "//". There are various ways to do this and I will go over other ways later. So, anything on the line with "//" is ignored by the compiler. It is just a note and a good idea to use throughout your program. I often use a series of comments at the top of my code as pseudocode. This helps me with organizing my program.
Syntax Errors
Every language has rules. C++ is no exception. Lines in C++ end with a semi-colon. If something is wrong, the compiler will issue a warning. Most compilers will tell you where in the program the error is coming from.
Comments
The code you write should say what you are doing but your comments tell you why.
This is important to remember. Programs should have comments to explain unclear portions. You can insert comments anywhere in your program. The compiler ignores all comments in a program.
Single line comments tell the compiler to ignore that one line.
// This is a single line comment
It is usefull to make notes to yourself. I often use these to indicate a section of code.
You can do multiple lines too. This is useful when you have a lot to write and want it to look organized.
/* This is a multiple line comment
* I like to use these to explain the program to myself
* It is a useful place to include your pseudocode for the program
* or any constraints that were given with the program task.
*/
I prefer to keep everything left-aligned when possibe. I like neat code but this is just personal preference. You can do it the way you want, C++ will not complain. Don't try to next comments, C++ will not allow that.
Use comments to say what things are and why they are doing that task. You will forget why you did things a certain way so always use comments in your program. This goes for every language, not just C++.
Comment your coder as much as it makes sense. Don't overdo it, however. It may take a while but go for a balance if possible.
Literals In C++
A literal is any value that is put directly in your code. It is not contained in a variable. These are literals:
"Hello World";
int num{10};
double pi{3.14};
These statements can have any of several different types.
Operators in C++
These are the symbols that tell us what to do with operands. Operands are the numbers, variables, or expressions that we work with. Operators are therefore +, -, *, and / symbols. There are several others too. You see them in statements and entire expressions.
2 + 3 /7 -5 *2
There is an order of precedence to all of these symbols. They are listed below in descending order:
- Parenthesis
- Exponents
- Multiplication
- Division
- Addition
- Subtraction
Expressions in C++
Expressions are combinations of everything you can put into code. Examples are literals, operators, variables, and functions. Expressions are evaluated to get their values.
2 * 3 + (1 + 2) -1 = ?
This is an expression and it uses multiple operators. There are many other examples and you will see them all eventually. The important thing to remember is that expressions can take many shapes and, for now, they will give only one value.
Variables
Variables are very im portant in learning c++. They are used to hold values. You can think of them as containers that hold a certain type of value. That type of value is determined when you declare the variable. So, it is up to you, the programmer.
Sometimes they can stay the same throughout a program or they can change. It depends on you. To use a variable in c++, you have to declare it with a data type.
There are several different data types. They can include:
- char
- int
- string
- double
- float
- bool
There are also others but they are special cases or variations of the ones I just listed. These types go in front of a variable. That is declaring the variable. Here are some examples.
char s;
int {7};
string "name";
double {5.5};
float {5.5};
bool yes;
A "char" is just a single ascii character. It is not a numeric character, meaning you cant do calculations on it easily. It is anything in single quotes. "Int" stands for integer and does not contain a decimal. It can be negative. Do all the math you want on these.
"String" is multiple characters, like a sentence. It is anything surrounded by double quotations. You can't do numeric calculation on it easily. "Double" is another numeric type. "Float" has a decimal and implies precision of a number. "Bool" is a logical operator and used for true or false operations.
Let's get to some example now, so this is clearer. I will start with the usual "int" type.
#include <iostream>
int main()
{
//variables
int x {10};
int y {15};
//output
std::cout << x + y << '\n';
return 0;
}
#include <iostream>
int main()
{
//variables
int number {10};
//output
std::cout << number * number << '\n';
return 0;
}
In these examples I used variables to do calculations. The highlighted section is where I declared an integer variable. i added the variables "x and y". I declared these as integers so that mean I could perform calculations on them.
In the second example, I did a slightly different calculation. I declared my one variable. Then I printed to the console the result of multiplying the variable by itself.
It is time to try a "string" example now. To use this function, you must include the "string" header file. It won't work right otherwise.
#include <iostream>
#include <string>
int main()
{
// declare variables
string name = "darkrai is a dark pokemon";
//output
std::cout << name << '\n';
return 0;
}
In this example, we are simple printing to the console again. It is important to see how a "string" works, because it is different than an integer.
I want to mention that you need to declare a variable with the same type of data that you defined it with. For example, you can't declare it with an integer value but give it a string value in double quotes. This will cause problems.
Variable Declarations
To use a variable, you first have to create it. This is done through a declaration.
double pi = 3.14;
The container type is "double" which tells us how much data this variable can hold. The variable name is "pi". We then use the assignment operator to copy 3.14 into the memory location designed as "pi".
You can define multiple variable on the same line. However, it is best not to. Do them one line at a time so they can be initialized and you can add comments to each as needed.
Initializing a variable is giving it a value when you declare it. You should always do this if given the option. If you do not give it a value, it will have garbage at that location. this can cause problems and bugs later. So, it is best to just initialize at the time of declaration. If you do not have a starting value to give it, then just set it to 0.
double weight{};
The variable "weight" is declared and set to 0. You will notice I used braces to initialize my variable. This is the modern way of doing this task. Using the assignment operator comes from the "c" language and we want to avoid that if possible.
Using brace initialization also helps to prevent bugs. If you initialize with an diffeerent value than the type of the variable, the compiler will throw an error. This is good because that will be a hard bug to track down. The older "c" style method of copy initialization allows it and that will introduce bugs. So you should avoid copy initialization. For initialization, use braces at all time if given the choice.
int num{};
So, to recap, always initialize your variables when you declare them. Use brace initialization when you can. This will be most of the time.
Constants
Constants are another container type. They are used when you do not want the value to change. Think of a value like "pi", it doesn't ever change. You denote a constant with the prefix "const". It looks like this.
const float pi = 3.14;
That value will not change during the course of a program and I doubt you would want it to.
It is a good idea to use constants anytime they make sense. If a value will not change, make it a constant. It makes your program easier to read and it is more efficient. When declaring your constant, you must initialize it.
Character Output
Sending output to the console is one of the most basic tasks you can do in C++. When you are doing that, you are using the output library that is built into the standard library. This is what the "iostream" library is for. In your program it looks like this:
#include <iostream>
The most commonly used function is:
std::cout
We used this earlier to print a message to the console. It stands for character output.
std::cout << "We love Pokemon" << '\n';
We can also print numbers or literals, variables, or whole expressions. Use the insertion operator "<<" more than once to print many different combinations of things. You can piece together text or include whitespace in a message.
Character Input
Getting user input is very important. It is important because many types of programs are based off the input that a user gives you. For example, a calculator type program is like this. It depends on the input that a user gives you.
There are various ways to get user input. Let's start with the "std::cin" object. It stands for character input and it is the most basic way to get input. It is used with the stream extraction character, ">>", that is used directly after using "std::cin". It is used with a space inbetween, just like the "std::cout" object does.
#include <iostream>
int main()
{
//variables
int num1{};
int num2{};
int num_out{};
//directions to user
std::cout << "Enter two numbers you need to multiply together" << '\n';
std::cout << "Please hit enter after each number" << '\n';
// get user input
std::cin >> num1;
std::cin >> num2;
//calculation
num_out = num1 * num2;
//output
std::cout << "The answer is :" << num_out << '\n';
return 0;
}
In the above simple program, you can see how the "cin" object can be used. This is a sequential program, the program flows from top to bottom. You can see the order of the program.
Basically, pretend the person to sitting in front of you and you ask them what they want. Tasks have to be done in a certain order so they make sense. Programming is no different.
We have to ask for input before we can out the answer. This may seem obvious, but many beginners have trouble with program logic. Hopefully, this way of thinking will help.
Just like "std::cout", you can get input more than one value in a single line.
std::cin >> a >> b >> c;
Be careful not to mix up the insertion "<<" and extraction ">>" operators. Doing so will cause major problems in your program. Your compiler will complain though, so it will be easy to find the problem.
String Input
If you need to get input like a name, then you use a string. This is useful when you need one word that is character based. Here is a quick example.
std::string name;
std::cin >> name;
std::cout << name;
This is super simple and not practical, but shows how you initialize, request input, and print it to the console. Remember, it only takes character input. You can't do calculations with string data.
Getline
Now, the problem is when you want to capture multiple words. Something like a sentence is what I am thinking of. For that, we need to go back to "cin" and use one of its member functions. This member function is "getline". It will capture a line of data.
getline(cin,name);
This will grab a line of input and put it into the variable that you designate. Let me do an example so it is clear.
#include <iostream>
#include <string>
int main()
{
// variables
std::string name;
// get input
std::cout << "Please enter your full name" << '\n';
std::getline(std::cin, name);
// output
std::cout << "You entered :"<< name << '\n';
return 0;
}
Again, this is simple but it shows how "getline" works. It is very useful. The more you learn, the more combinations we can use. Many programs will use sequences like this to gather user input and do something with that data afterwards.
Variables In C++
In C++, we work with information. Sometimes, that information changes. Therefore, we need variables. Variables let us change the information that we work with. They allow you to store and work with information. Variables let us identify the information that we are working on. Once identified, we can change or manipulate it as we need to.
#include <iostream>
int main()
{
int var {1000};
std::cout << var << '\n';
return 0;
}
When we create a variable, we store it in memory. So, you can also say that it stored our information in memory. This is because a variable is a type of container that stores things. This is one of your tasks as a programmer, to determine how many and what size variables you need in a program.
Data Types
Variables can store different information. We call these data types in C++. The differences in data types are mainly about size and type of information. The size distinguishes the different types.
Here are some common data types:
int
char
string
float
double
bool
The 'int' data type, for example, can be signed or unsigned. A signed data type means it can be positive or negative. Each numerical data type will have a maximum value that it can hold. The maximum value is directly related to how much information that it can hold.
An unsigned data type, like an integer, can hold a number at twice the value of a signed integer. This is because, if there are no negative numbers, then the variable can be all positive and therefore, twice the value.
Data types can be changed too. You can use short, unsigned, or long to optimize a variable. For example, if you need to store a value of 3 billion, then use an unsigned integer as your data type. You would do this because it uses less memory than a regular integer.
Modifying Data Types
Saving memory and being efficient becomes important later on. It is something you want to keep in mind. That is why modifiers like short, unsigned, and long exist. They are there to help you make the most of the memory you have to work with.
The size of data types are dependent on the compiler you use. There are slight differences between compilers, so it is important to know about the compiler that you are using.
We can find out the size of our data types with the “sizeof” command. You should do this every time you use a new compiler or machine, so you can plan your memory usage accordingly. We will look at this function at the end of this article.
Use unsigned data types when you know your program won’t have negative values. This lets you have larger values in your variable without having to use a float or a double, which uses more memory.
Declaring Variables
When you declare a variable, it is called a variable declaration. This declaration lists the variable’s name to the compiler and says what data it will hold. There must be a definition for every variable in your program. These definitions are a statement and must have a semicolon at the end of the statement.
// This is a variable definition and assignment
int num {15};
When you give a variable a value, it is an assignment. The equal sign copies the value on the right into the variable on the left. When information is stored in a variable, the entire operation is an assignment statement.
When a value is copied into a variable, the ‘=’ is used to do this. We call this the assignment operator. Operators work on our information. The information that it works on is called the operands.
When copying values to variables, the variable has to be on the left side of the operator. You can also assign value when you declare the variable. We call this initializing the variable.
You can declare many variables at once. So, instead of using a separate line for each variable, you can declare several at once if they are the same data type. It’s also less typing, which can be important too.
// Declaring multiple variables with one statement
int age, weight;
double mass, distance;
You can put them anywhere in your program as long as it is before you try to use them. However, I think it’s best to be organized and have a variable section for each program. It makes your code easier to read.
However, it is better to always initialize variables when you create them. So, the above method is not the best. Just wanted to mention it is possible. Also, when you initialize variables, do so with values that match their type. Initialize an integer with an integer value. The same goes for float and double.
int {1};
float {5.5};
double {.345}
char 's';
string "name";
Naming Variables
Naming variables is an important task. It does not take much effort, but it should be done with some thought. You want it to be short but meaningful to a stranger who has never seen your code before. That is what you are trying to do.
When you name variables, it can’t be a C++ keyword. The first character must be a letter or an underscore. After the first character, you do also use numbers as well as letters and an underscore. Variables are case sensitive so you will need to pay attention to that.
Integers
Integer data types only hold whole numbers. When selecting a data type for your variable, you have a few things to think about. You want to consider the largest and smallest value that this variable is supposed to hold. Know how much memory the variable will use.
// Assigning an integer value
int book_amount {1700};
int number_shelves {6};
Think about whether the variable can be unsigned or should it remain signed. Last, you need to know how much precision the variable must account for.
// Using an unsigned integer value
unsigned int age {22};
// Age will never be negative so this is a good use for an unsigned integer
Here are some examples of numeric modifiers:
short = 2 bytes
unsigned short = 2 bytes
int = 4 bytes
unsigned int = 4 bytes
long = 4 bytes
unsigned long = 4 bytes
#include <iostream>
int main()
{
float length {20.3};
float width {23.8};
float area = length*width;
std::cout << area << '\n';
return 0;
}
The long modifier is a special case. On some older systems, long is needed in tandem with the integer data type. I think this would be a pretty old system but I didn’t want to discount it because other parts of the world may use that type of equipment.
Char Data Type
This is the character data type, and it uses 1 byte of memory. We mostly used it for storing characters, however, that can really be anything. This is because characters are really just numbers in the background.
// Declaring a char data type variable
char letter = 'A';
Most characters these days are encoded using ASCII. There are older formats also but they are not really in use too much these days. This means that ‘A’ has a certain ASCII number and so does ‘a’. Case matters and different cases of the same letter have different numbers.
So, when a character is assigned to a variable, it is the number that represents the character that is really stored.
When assigning char values, you use single quotes around the value. An example would be ‘A’ or ‘b’.
#include <iostream>
int main()
{
char letter;
letter = 75;
std::cout << letter << '\n';
letter = 76;
std::cout << letter << '\n';
return 0;
}
Char variables can’t hold a string but there are ways around that. Typically, you use a char array. So, it is possible. However, it’s probably just better to use an actual string. I will show you those soon.
Floating-Point Data Type
Floating-points are a data type that are used to hold real numbers. Real numbers, if you remember from set theory, can have a decimal. Decimals are an important part of many programs and operations.
// Declaring a floating-point variable
const float pi {3.14};
We can further divide this data type up and each has their uses.
floats = 4 bytes
double = 8 bytes
long double = 8 bytes
#include <iostream>
int main()
{
float height{};
float width{};
float wall{};
std::cout << "Please enter dimensions of your wall in meters " << '\n';
std::cout << "Enter your height in first and then your width" << '\n';
std::cin >> height;
std::cin >> width;
wall = height*width;
std::cout << "Your wall takes up this much space: " << '\n';
std::cout << wall << " meters squared " << '\n';
return 0;
}
The actual size of these data types on your computer will need to be determined. Usually, though, float is the smallest and long double will hold the largest values. There are also no unsigned floating-point data types. That means they can all be positive or negative.
Bool Data Type
The bool data type is either false or true. To the computer, that means 0 or 1. We call these boolean expressions. They allow you to create small variables that hold true or false values.
// Declaring a boolean variable
bool tf;
Boolean operations are great for logic problems. You can get very creative with them and that is where they show their flexibility.
#include <iostream>
int main()
{
bool answer;
answer = false;
std::cout << answer << '\n';
answer = true;
std::cout << answer << '\n';
return 0;
}
Data Type Size
You should determine the size of a particular data type on your machine. This is because machines and compilers all vary slightly and this is something you want to know for sure.
We accomplished this by using the ‘sizeof’ function. It will report the number of bytes used by a data type or variable.
#include <iostream>
int main()
{
int wall{};
float area{};
double pi{};
std::cout << "The size of data types will be given in bytes" << '\n';
std::cout << '\n';
std::cout << "An integer is " << sizeof(int) << '\n';
std::cout << "A float is " << sizeof(float) << '\n';
std::cout << "A double is " << sizeof(double) << '\n';
return 0;
}
Variables are a simple part to learn in C++ but they are essential to get right. In this article we talked about what they are used for, declaring them, modifying them, and the different data types we can use. Finally, I showed you how to find the memory each data type takes up on your machine.
Decisions In C++
This is one of the core topics in programming and something you will use a lot. Every language I can think of has methods to make decisions. These are conditional statements and enable branching behavior.
Making decisions specifically means that you will be comparing data or C++ statements. We can do this with numeric or character operators. Logic operators work left to right. Now we can ask for input and make a decision.
[If] Statement
Using [if] lets you make decisions in your statements. They will only execute [if] we meet a condition. That is how [if] works. For example, if one condition is true, your code can jump to a certain part and execute something. If the condition is false, it will go in another direction.
The syntax of an "if" statement looks like this:
if (condition)
{
Do something based on condition
}
The purpose of an "if" statement is to evaluate a condition. You can test if something is true or if it is false. An example is asking if the temperature is over 100 or less than 0. Then, in your block between the braces, the program does something based off the condition.
Detriments Of Conditionals
We store all the conditionals that you write in memory. This means our program will have to access different portions of memory. This can slow a program down. I advise not to use a lot of conditionals. It's ok to use them in small numbers, just watch your performance.
Making Decisions With An If Statement
#include <iostream>
int main()
{
// variables
float gpa;
// get input
std::cout << "Please enter your gpa" << std::endl;
std::cin >> gpa;
// conditional
if (gpa>=3.0)
{
std::cout << "You have good grades, nice going!" << std::endl;
}
return 0;
}
This is our first example. Remember, the lines starting with "//" are for comments. They just document the code.
So, in this program, I wanted to ask someone's gpa. Then I wanted to congratulate them if it was good.
- created variable
- got input from user
- made a conditional
- output a result
Look and see how the "if" statement was used there. Give it a condition and then do something.
You can see from the code that my condition was ">=3.0". When I ran this code, I inputted 3.2. I have high hopes for myself, as you can see! Anyway, because the condition was greater than or equal to 3.0, it output the congratulatory message.
What happens if my gpa is below 3.0? What will my code do? Since there is not a path for the program to take if the condition is false, it will do something useless. We need to add a condition that accounts for a lower than 3.0 gpa. Then, we need to tell the program what it should do at that point.
#include <iostream>
int main()
{
// variables
float gpa;
// get input
std::cout << "Please enter your gpa" << std::endl;
std::cin >> gpa;
// if conditional is true
if (gpa>=3.0)
{
std::cout << "You have good grades, nice going!" << std::endl;
}
// if conditional is false
else
std::cout << "You need to work harder" << std::endl;
return 0;
}
In this snipet, I added an "else" condition. This tells the program what to do if the original conditional is false. So, if our gpa is below 3.0, then the program tells us to work harder. I also color-coded the changes I made so it would be easy to see.
With "if" statements, you can use them anywhere in your program. There can be multiple conditions and do multiple things. We can also test conditions as strings or other variables. Here is another example.
#include <iostream>
int main()
{
// variables
std::string fav_pokemon;
// get input
std::cout << " Try to guess my favorite Pokemon " << std::endl;
std::cin >> fav_pokemon;
// conditional
if (fav_pokemon == "darkrai")
std::cout << " You guessed right! " << std::endl;
else
std::cout << " Sorry, that is incorrect " << std::endl;
return 0;
}
In this program, I am asking you to guess my favorite pokemon. Easy enough, right! As you can see, I am using strings this time to get string input. This is all very simple, but it shows you how you can use "if"statements.
Also know that we can test for multiple conditions. If I want to make it a bit easier to guess my favorite pokemon, we can add that to the test.
#include <iostream>
int main()
{
// variables
std::string fav_pokemon;
// get input
std::cout << " Try to guess my favorite Pokemon " << std::endl;
std::cin >> fav_pokemon;
// conditional
if (fav_pokemon == "darkrai" || fav_pokemon == "vileplume")
std::cout << " You guessed right! " << std::endl;
else
std::cout << " Sorry, that is incorrect " << std::endl;
return 0;
}
As you can see above, I tested for either darkrai or vileplume to be true. So, if a person guessed either, then they got my true message.
Complex Branching
Previously, we have just used a simple form of flow control. One"if" and one "else" statement. We have more options, however. There can be multiple branches. We call these nested statements.
Indent each level of nesting. This makes your code easier to read and prevents silly mistakes. Try to keep all the levels the same. You can use the "tab" key or whatever you want.
Be careful designing your "if else" statements. It is easy to get your program where it will compile, but you get the wrong output. Make sure and use braces to control the flow of your program. You don't want the compiler to give a wrong value through a logic error. These can be hard to track down because there are no warnings.
The compiler pairs an "else" with the nearest "if" that is not already paired. This is the purpose of braces, so you can make sure the program goes in the direction it is supposed to.
#include <iostream>
int main()
{
// variables
float gpa;
// get input
std::cout << "Please enter your gpa" << std::endl;
std::cin >> gpa;
// conditional
if (gpa >= 3.8)
std::cout << " Those are exceptional grades " << std::endl;
else if (gpa >= 3.0)
std::cout << "You have good grades, nice going!" << std::endl;
else
std::cout << "You need to work harder" << std::endl;
return 0;
}
In the new section, I added another condition, by using an "else if" statement. This technique can give the beginner precise control of the flow of their program. It is testing for a different positive condition.
Now, if the gpa is between 3.0 and 3.8, it triggers a different message that is more accurate. If none of the conditions hold true, then our back statement at the end will display.
In this next example, I do the same with my pokemon guessing program.
#include <iostream>
int main()
{
// variables
std::string fav_pokemon;
// get input
std::cout << " Try to guess my favorite Pokemon " << std::endl;
std::cin >> fav_pokemon;
// conditional
if (fav_pokemon == "darkrai")
std::cout << " You guessed right! " << std::endl;
else if (fav_pokemon == "vileplume")
std::cout << " I like that pokemon too ";
else
std::cout << " Sorry, that is incorrect " << std::endl;
return 0;
}
Instead of using an "or" or "and" logic in my conditional statement, I break it up and use an "else if". This is just another way of doing it. Remember, we check conditions from the top. As soon as a condition is true, the rest of the block is skipped.
Keep in mind that any statement outside of the "if" block will always run. This is because it is not part of the conditions, so it is not checked and it is a regular C++ statement.
C++ is a really cool language. Despite its age, it is still being updated and is one of the top languages around. For hardware programming it is still king and nothing will take its place soon. You can do much more than hardware programming in it, though.
Looping In C++
We often need to run a piece of code many times to get results that we need. Looping is how this is done. In this section, I will talk about the different types of loops and show some simple examples.
Incrementing and Decrementing
Variables are increased or decreased as needed. You will often have a counter variable for this purpose. These counter variables are useful in loops. They keep track of how many times the loop has executed. The “++” and “--” operators are used to increment and decrement variables.
#include <iostream>
int main()
{
int counter = 1;
std::cout << “Our variable is currently equal to “ << counter << std::endl;
counter++;
std::cout << “We incremented our variable, and it is now “ << counter << std::endl;
counter—;
std::cout << “Now we decremented our variable and it is again “ << counter << std::endl;
return 0;
}
Looping
For the beginner, looping is where a piece of code repeats. It will repeat until it meets a condition to stop. You, as the programmer, will give it this condition. If you do not give it a condition to stop, it will run forever. This is bad. It is an infinite loop. You normally do not want this.
Therefore, a loop is a code structure than repeats a group of statements. C++ has the “while” loop, “do-while” loop, and the “for” loop.
Loops are where we use counter variables and they are important in these structures.
While Loop
A while loop executes and keeps running as long as its test condition remains true. It comprises two parts. One part is where it tests to see if its condition is true. The other part is the code that gets repeated.
The condition to be tested has to be a true expression. Again, it only runs if it is true.
#include <iostream>
int main()
{
int var = 10;
while (var <=20)
{
std::cout << var << std::endl;
var++;
}
return 0;
}
At the beginning of our program, we defined and initialized our variable. We then set our condition to be tested and put this in our while loop. It tests the variable to see if it is less than or equal to 20. When it is, variable value prints on the screen.
The loop increments the variable each time the loop runs. When the variable reaches the value of 21, the loop stops because the condition I set is no longer true. Each time the loop runs, it is an iteration.
If you have noticed, the while loop tests its condition before it runs. This means the loop will never run if the condition is false from the start. It is an important consideration if you need the loop to run at least once.
Let us now do some decrementing in a loop. It works the same way. We test the condition to see if is true. If it is, then the loop runs an iteration. This continues until the condition is false.
#include <iostream>
int main()
{
int var = 50;
while (var >=10)
{
std::cout << var << std::endl;
var —;
}
return 0;
}
While loops are handy for working with numbers and you can do a lot with them. Here is another common example, where I square several numbers. The condition in the while structure tells it when to stop.
#include <iostream>
int main()
{
int var = 5;
std::cout << “variable\tsquared” << std::endl;
while (var <=25)
{
std::cout << var << “\t\t” << (var*var) << std::endl;
var++;
}
return 0;
}
Do-While Loop
This type of loop is the opposite of the while loop. It is because it tests its condition after the loop runs once. This loop structure is useful in the right situation. It is useful when you need the loop to run at least once. This is important to remember.
#include <iostream>
int main()
{
int counter = 0;
do
{
std::cout << counter << std::endl;
counter++;
while (counter <=20)
}
return 0;
}
This program takes the opposite method of counting numbers. It is just as good as the regular while loop, it just depends on your situation. There will be times when it is best for one or the other. Be flexible in your coding and don’t get stuck doing things only one way.
The For Loop
This is another type of loop and it is an excellent choice when you know how many times a loop must iterate. The two previous loop structures only run as long as they test true. It could be zero or infinite many times. The For Loop will execute a certain number of times and that is it. You dictate how many times that is.
The For Loop is unique because it has a few requirements. It has to initialize a counter variable, it must do a test, and it has to update the counter variable. Let us do an example to see how it works.
#include <iostream>
int main()
{
for (int counter = 0; counter <=10; counter++)
{
std::cout << counter << std::endl;
}
return 0;
}
The top line of the For Loop includes the initialization, the test, and an update. This processes work the same asin the other loops. They are just in one line now. Notice the lack of semi-colon after the counter update expression. This often trips people up at the beginning.
The counter variable is used to control how many times the loop runs. This happens until the test condition is false. Then the loop ends.
The For Loop is versatile. You should use it when it makes sense to do so. For example, use it when you need to initialize a variable or when you need to update a counter. It is ideal for these situations.
Keep Running Total
Keeping a running total is very useful. You can keep track of sales, your dps in your favorite mmo, or even your stock gains. The uses are endless and only reliant on your imagination.
A type of variable that is used for this purpose is an accumulator. It is used to keep a running total. It reads each number and adds it to the total. Most of the time the accumulator is initialized to zero, but this could change depending on your needs.
#include <iostream>
int main()
{
int total = 0;
int days;
int coffees;
std::cout << “How many days did you have coffee this week? “ << std::endl;
std::cin >> days;
for (int counter=1; counter <= days; counter++)
{
std::cout << “How many coffees did you have today “ << std::endl;
std::cin >> coffees;
total = total+coffees;
}
std::cout << “You have had “ << total << “ coffees this week” << std::endl;
return 0;
}
Sentinel Values
A sentinel value is an entry that marks the end of a list of entries. It says to stop doing what you're doing. We use this in loops when you do not know how many items there are to work with. In a For Loop, for example, you tell the loop how many times to run and then do something. If you don’t know how many times the loop is supposed to run, you use a sentinel value.
This gives your loop and program a lot of flexibility. We just don’t always know how many times our loop is supposed to iterate. So, we just ask the user to enter a sentinel value when their task is done.
#include <iostream>
int main()
{
int hour = 1;
int people;
int total=0;
std::cout << “Enter number of patrons in coffee shop by the hour:” << std::endl;
std::cout << “Enter -1 to stop” << std::endl;
std::cout << “Enter number of people:” << std::endl;
std::cin >> people;
while (people != -1)
{
total=total+people;
hour++;
std::cout << “Enter number of people:” << std::endl;
std::cin >> people;
}
std::cout << “The total is “ << total << “ people” << std::endl;
return 0;
}
In this program, I do not know how many hours I plan to stay in the coffee shop. Coffee is too good to just leave! Anyway, I just set up a sentinel value so I can tally my figures anytime I want. When I enter the sentinel value, the loop stops.
Indenting Loops
There is nothing technically wrong if you do not indent your loop code. However, it is a good idea if your program is more than a few lines. I’ll admit I am not the most consistent. In longer pieces of code, I will indent. It makes everything more readable. There are time I do not. If something is extremely short and won’t be used anywhere, I often don’t. If in doubt, just indent.
Pretest and Posttest Loops
This can be pretty important to understand. In a pretest loop, it will not run until your condition returns true. It is the opposite in a posttest loop. In a posttest loop, the loop will run once and then test for a true condition.
In some loops, you want your loop to run once, no matter what. This means you need to use a posttest loop so it will run once and then test for its condition.
Conditional Statements
Conditional statements are run if they meet the correct condition. We set this condition up in the loop definition. You set the definition of the loop. So, if the condition is met, the statements inside the loop will run.
Difference Between Loops
The While Loop is a pretest loop and its statements will only run if its condition is true. We evaluate the condition before the loop runs once. In a Do-While Loop, the loop code is run once and then the condition is tested for the first time. This makes it a posttest loop. It is useful when you need code to run once, no matter what.
In this section we have briefly talked about variables inside loops. These variables can be increased or decreased as needed. This lets us control the loops and set condition for stopping the loop.
We also discussed the different types of loops and their different benefits. Since there are both pretest and posttest loops, each has advantages. Those are While Loops and Do-While Loops. then we talked about For Loops. These are great when you want a loop to run an exact number of time before it terminates.
Then we talked about keeping a running total. This technique is great for certain tasks, like counting how many times a loop runs. Sometimes we don’t know how many times we need it to run, but we want to know the results afterwards.
Functions In C++
Calling Your Function
After you write a function that does some task, you have to call it when you want to use it. This will be in your [main()] function.
#include <iostream>
void some_function()
{
std::cout << "Hi";
}
// Main Function
int main()
{
some_function();
return 0;
}
#include <iostream>
// Function Definition
void way()
{
std::cout <<"This is the way. \n";
}
// Main Function
int main()
{
way();
return 0;
}
This is another example of a function.
Void pokemon()
{
Std::cout << “charmander, pikachu, crobat, darkrai, eternatus \n”;
}
This is the function definition and it has to be defined before the main function. There are not many other rules, it just has to be legal c++ code in the function. You call the function in your program like this:
pokemon();
This function call will run the code that was defined. Here is a short example program using the function we just created.
#include <iostream>
void pokemon()
{
std::cout << "charmander, pikachu, crobat, darkrai, eternatus \n";
}
int main()
{
pokemon();
return 0;
}
You can have many functions in a program. You can define them at the beginning and then use them as needed. When you call a function, the program branches to that function and runs what is inside. Then, it returns to the main function.
#include <iostream>
#include <cmath>
double user_input_number()
{
std::cout << "Please enter a number : \n";
double num{};
std::cin >> num;
return num;
}
int main()
{
double value{user_input_number()};
std::cout << "The square root of " << value << " is: " << sqrt(value);
return 0;
}
Return Statement
Returning True/False Values
So these are functions. I have gone over how they are created and slightly how they are used. There are infinite combinations. It may not seem very useful but as you get more proficient in using functions in C++, you will want to use them.
Returning Values
A function does not have to return any kind of value. We looked at those kind of functions above. They just printed a message to the console. That behavior is useful in some situations.
There are times, however, when we need a function to return a value. A common practice is to get input from a user. If this is part of a function, we need to send that input to the main program so it can be used. Let me show you a sequential program that is not modular and then one that is, so you can see the difference it makes.
#include <iostream>
int main()
{
double num{};
double num_squared{};
std::cout << "Enter a number you want to square : " << '\n';
std::cin >> num;
num_squared = num * num;
std::cout << "Your number squared is : " << num_squared << '\n';
return 0;
}
As you can see, we have multiple variables and other statements. In practice, we always want to make our program modular if possible. So, we take individual parts and turn them into functions. Once they are functions, you can store them in a header file or something else. That way, they can be reused when needed.
#include <iostream>
double get_num()
{
std::cout << "Enter a number you want to square : " << '\n';
double num{};
std::cin >> num;
return num;
}
int main()
{
double num{get_num()};
double num_squared{};
num_squared = num * num;
std::cout << "Your number squared is : " << num_squared << '\n';
return 0;
}
I made a function called "get_num()" and this contains the code to get user input. The definition is outside of "main" now. See the code that remains in "main"? Anytime we need to get user input we can use something similar.
Notice how the variable "num" is initialized with the function. The function returns a value of type double. So the variable "num" is initialized with the return value.
Functions have some rules to follow. Any function that should return a value must do so or your compiler will complain. Your "main" function does not have to have a return statement. However, it is good practice to do so. A function that returns a value can only return one value at a time. The values can also be variables, expressions, or another function that gives a value. When you create a function, you can decide what kind of value it returns or if it returns one at all.
Void Functions
Void functions do not return a value. "Void" is the return type, which means the function returns nothing.
void print_message()
{
std::cout << "Hi" << '\n';
}
In the above function, you can see there is no return statement. Void functions do not need a return statement. You use this as you do any other function. You call it when you need to.
#include <iostream>
void print_message()
{
std::cout << "Hi" << '\n';
}
int main()
{
print_message();
return 0;
}
Remember the program starts at the top of the "main" function. It goes sequentially from top to bottom. When it encounters the function call, it looks for the function definition and executes it. Then the program continues to the end of the "main" function. Functions that are part of an expression must return a value. So, that means we cannot use a "void" function in an expression. We must use it by itself.
Function Parameters
void output_num(double num)
{
std::cout << num << '\n';
}
This function outputs a number and it is designed as a double. The parameter "num" will come from outside the function. Notice, since we do not return any value here, the function type is "void".
double multiply(double num1, double num2)
{
return num1 * num2;
}
This function goes a step further and returns a value. The function type is double along with the parameters. When this function is called, the two parameters will be multiplied together and that value will be returned.
#include <iostream>
double multiply(double num1, double num2)
{
return num1 * num2;
}
int main()
{
std::cout << multiply(4.4,5.5);
return 0;
}
In this program, we pass arguments manually to the "multiply()" function. We output this result. This is not very useful, but it does show how parameters and arguments work together.
Now, let us do something a bit more fun.
#include <iostream>
#include <cmath>
double user_input_number()
{
std::cout << "Please enter a number : \n";
double num{};
std::cin >> num;
return num;
}
int main()
{
double value{user_input_number()};
std::cout << "The square root of " << value << " is: " << sqrt(value);
return 0;
}
Here, we showcase the combination of functions working together. Ignore the "cmath" header for now, it is just to use the "sqrt()" function. What we are doing is getting a number from the user. Then in "main" we declare a variable named "value" and initialize it with the value returned from our function. This is the number the user provided. then, we just take the square root of this value using that function.
Function Prototypes
#include <iostream>
pokemon();
int main()
{
pokemon();
return 0;
}
// function definition
I will say its a matter of personal opinion whether you use a prototype or not. At this point it doesn't make any difference. I prefer not to use prototypes myself but again it doesn't matter.
Local Scope Variables In C++
Variables and parameters of a function are called local variables. The function can be “main” or or one that you created. If you define a variable in “main”, that variable can only be used in “main”. A variable created and defined in a function you create cannot be used in “main”.
Local Variables
Look at the following piece of code. The variable is local to the “main” function because it was created and defined there.
#include <iostream>
int main()
{
int num{}; // the variable “num” can only be used in the “main” function
return 0;
}
If we create a function and define a variable inside, that variable will be local and in scope to that function. It will not be visible to the “main” function.
#include <iostream>
int multiply( int a, int b)
{
return a * b;
}
int main()
{
std::cout << multiply(5,3);
return 0;
}
This program will output 15 as expected. The variables “a” and “b” are local to the “multiply” function. This means they are in scope to “multiply”. However, they are not accessible to the “main” function and are out of scope to “main”.
Variable Lifetime
The reason variables are out of scope between functions is they do not exist after their function runs. When a variable is defined in a function, it is destroyed at the end of that function. It can be the “main” function or “multiply”, it does not matter. To be exact, variables are destroyed at run time, not at compile time. It happens at the ending curly brace of the function it was defined in.
int multiply( int a, int b)
{
return a * b;
} // both variables “a” and “b” are destroyed here when the program gets to this ending curly brace
Local Scope
The scope of a variable determines whether it can be accessed. If it is in scope then it can be accessed. However, if it is out of scope, then it cannot be accessed. Scope is determined at compile time. A variable’s life begins at the point of creation and ends at the end of the function in which it is created.
Conclusion
In this section, we talked about local scope in C++. Local variables are local and in scope to the function they were created in. Variable lifetimes start when they are created and end at the last curly brace of the function they were created in. We then talked about the differences between being in scope and being out of scope.
Naming Collisions
A naming collision happens when there is an identifier that is not unique. Why might this happen? You could use the same name in two different parts of your program. There could be multiple files that need compiling which have the same name. The linker would give an error in this case. Also, future versions of C++ might have a new name for an identifier that breaks an existing program.
If this happens, the compiler will give an error. If your project contains thousands of lines, good luck figuring out where everything is.
This problem happens with variables and functions. With variables, you have global and local scopes. You can prevent a lot of issues by using local scope variables. However, we do not get this luxury with functions. Either of these can cause a naming collision.
This leads us to namespaces. Namespaces were created so that we can use the same name identifier, or at least so they would not break a project. There is the standard namespace and we can also create our own namespaces. The advantage to a namespace is that anything inside one will not cause errors with one outside of it. It is a good idea to create your own namespace and include your functions in it. When you then refer to that code inside that particular namespace, it will not cause any naming collisions or conflicts.
The global namespace contains anything that is not already part of another function, class, or another namespace. A global variable would be an example of this. This is an important reason to avoid global variables if you can. For example, any variable outside of the main function is a global variable.
#include <iostream>
int num{};
double x{};
int main()
{
return 0;
}
The variables declared above the main function are global variables. If you have another file that has the same name that is in the global namespace, you will have an error and your project will not compile.
The standard namespace was created to address this issue. All the original functions and identifiers were in the global namespace. However, this caused issues as it was easy to have naming collisions from other files or libraries. So, they were all moved to the standard namespace. So, when we want to use a function from that namespace, we have to designate it.
std::cin
That is using the cin function inside the standard namespace. This is the proper way to do it.
#include <iostream>
int main()
{
std::cout << “I live in the standard namespace”;
return 0;
}
Try not to use using-directives as there is no good purpose for most people.
The Array Data Structure In C++
An array is a variable of sorts. It is a construct that can be used like a variable but hold many values instead of just one. These values must all be the same data type. Data types are short, long, double, float, bool, and strings.
Those are just the main examples, I did not list every data type. So, in short, an array lets you hold and work with multiple values of the same data type.
An array looks like this:
int list[10];
Arrays must be of the same data type. Any of the legal data types in C++ will work. In my first example, [int] is an integer, [list] is the array name, and [10] means there are ten elements in the array.
The size of the array must be a positive integer, no decimals allowed.
Memory
The memory used by an array depends on the data type and the number of elements. The more of each, the greater the memory required. So you will have to make a decision and decide how large you want your array to be.
Accessing Elements
Remember, an array is just a special type of variable. Don’t let it confuse you. This special variable is divided up into many other variables. That is best way to think of it.
The discrete elements will each have its own subscript. You will use the subscripts to access the elements of the array.
A key point in arrays and their subscripts is how to use the subscripts. A [10] element array will be numbered from 0-9. A [25] element array will be numbered 0-24. Hopefully that is clear.
Accessing the elements to use them is easy enough. It is done like this:
list[5] = 75;
In this example, element [5] has been assigned the value of 75. It is important to know that you can’t use an element if it does not have a value. So you have to give elements values in order to use them as variables.
Giving and Getting Information From Arrays
Since they are like variables, lets try some examples and see how to use them. Here is a small program that creates an array and sums its contents.
#include <iostream>
int main()
{
// data is open tickets by employee
const int employees = 5; // employees
int tickets[employees]; // elements of array
int total = 0; // total tickets
int count; // loop counter
// get tickets outstanding
for (count =0; count < employees; count++)
{
std::cout << "Enter the current tickets by each employee " << (count + 1) << ": ";
std::cin >> tickets[count];
total += tickets[count];
}
std::cout << "The number of tickets we have is " << total;
return 0;
}
This is a typical program except for the array part. At the beginning of the main function I create the array and use a constant integer variable so it can’t be changed by the program later.
The other variables are self-explanatory. I have one for the total amount of tickets and a loop counter. Both of these are necessary.
In the next section I have a [for] loop that takes input from the user and stops when I have hit the last employee. As the loop runs I am making a running total of the tickets we have. Then lastly I print to the screen our total.
Averaging the Values of an Array
We have done most of the work already to do this. So it will be just another couple steps. In our current program we already have a total to work with. So all we need to do is some division. After we have done the calculations, we will just display our results.
#include <iostream>
int main()
{
// data is open tickets by employee
const int employees = 5; // employees
int tickets[employees]; // elements of array
int total = 0; // total tickets
int count; // loop counter
double average; // average of our tickets per employee
// get tickets outstanding
for (count =0; count < employees; count++)
{
std::cout << "Enter the current tickets by each employee " << (count + 1) << ": ";
std::cin >> tickets[count];
total += tickets[count];
}
average = total / employees;
std::cout << "The number of tickets we have is " << total << std::endl;
std::cout << "The average number of tickets we have per employee is " << average;
return 0;
}
In line 12 I added a variable to hold our average value. I output its value on line 25 since I already had the other data I needed. That is really all there is to it. It was nice and simple to add that addition.
Reading Data From a File
This is the same topic I covered a long time ago in an earlier article. The difference is that I am putting the data into an array after reading the file. Here is the code, I will go over it.
#include <iostream>
#include <fstream>
int main()
{
// variables
const int number = 5;
int array[number];
int count;
std::ifstream inputfile;
// opening file to read from
inputfile.open("numberfile.txt");
// reading from file into the array
for (count = 0; count < number; count++)
{
inputfile >> array[count];
}
// closing our file
inputfile.close();
// printing the elements of the array one line at a time
for (count = 0; count < number; count++)
{
std::cout << array[count];
std::cout << std::endl;
}
return 0;
}
So in this program I start it out just like most others. I organize my program into its sections to keep things neat. I create my constant and initialize my array. I use a text file I have with a few property numbers to read from. It's a good example.
I then start a [for] loop to read through the file and assign values to my elements in the array. After I am done with the file I close it so I don't get any weird errors with it being open.
Then I use another [for] loop to print the values that have been stored in the array. All goes according to plan and we have the numbers printed to the screen. So this example file only had 5 numbers.
You might ask why would you do this for 5 numbers ands the answer is that you would not. However, what if you had 500, 5000, or 50000 numbers to read and do some calculations.
That is where the real power of C++ comes into play. It is powerful and fast. It will make short work of intensive calculations such as the example I just mentioned.
Finding The Highest Number In An Array
If you have to compare values from a lot of data this can be very useful. If I have 1000 values I can input them into an array and compare quickly to see what is the highest. Let me show you how this works.
#include <iostream>
#include <fstream>
int main()
{
// variables
const int element = 100;
int array[element];
int count = 0;
int highest = 0;
std::ifstream inputfile;
// opening file to read from
inputfile.open("numberfile.txt");
// reading from file into the array
for (count = 0; count < element; count++)
{
inputfile >> array[count];
}
// closing our file
inputfile.close();
// printing array values
for (count = 0; count < element; count++)
{
std::cout << array[count] << std::endl;
}
std::cout << std::endl;
// finding highest value in the array
highest = array[0];
for (count = 1; count < element; count++)
{
if (array[count] > highest)
highest = array[count];
}
std::cout <<"The largest of these values is " << highest << std::endl;
return 0;
}
In this example, I had a 100 values in a text file. I wanted to read each of them into an array and then do a comparison to see which was the largest. After they were in the array, I went ahead and printed them onto the screen.
I then added a section to analyze each of them. It was interesting to me to do it like this because I did not want to read over each line. This was only 100 values. What if I had 20000 to look at? I would have some headache after all that!
I am going to end here, but I have more to do with arrays in my next C++ article. They can be used with functions which will be very helpful. I want to also start sorting them so we can do more processing, yay!
Hope someone enjoyed this article. I put a lot of work into it. Every day we are bombarded with data from a variety of sources. I find it handy to be able to process large amounts at once when it is presented to me.
Vector Containers in C++
The Vector container in C++ is very useful. It will be the better option when you want to store data in an array. Vector has several advantages over a traditional array structure. This makes it more flexible.
The Vector container originates from the STL(standard template library). It is one of the C++ essentials you will need to learn well. The STL has many such data structures and algorithms built in.
A Vector array can hold a sequence of data. This data is stored in memory next to each other. You can access its elements like you would in a traditional array.
A Vector container has advantages over the traditional, built in array. You can easily find out the current size of your array with member functions. A Vector will grow as needed.
It can be full of data but will add an extra element at the end if it is needed. Vectors do not have to be a certain size when created.
You need to use a Vector container if your data changes regularly. It will save you lots of headaches. It is a good way to handle input and output. A Vector will also make your program more efficient. We should all strive for this.
In C++ we have the <string> class which makes text much easier to work with. It is a vast improvement over the C language use of char’s.
Creating and using a Vector is easy to do. You must include its header file.
#include <vector>
Defining a Vector container is slightly different than a regular array. It looks like this:
vector<string> PokemonSets;
vector<int> number;
There is no space between the data type and the word “vector”. You will not need to declare a size because it expands as needed. However, you can include a starting size.
This is good because it makes this container really flexible. You will need to specify the data type like <int> and a name for your Vector like <number>.
vector<double> LargeNumber(5, 5);
In this example, “LargeNumber” is created with 5 elements and all of them are initialized to 5.
You can use all the typical data types with a Vector. Float, int, char, string and double are all valid. Let us now start with some basic examples so you can see how this works.
I will start with the <push_back> c++ function to add elements. The <push_back> function is an example of a modifier. Use it to modify the contents of your Vector.
Adding Elements
This first example used the <push_back> member function to add values to the Vector. This function adds a value to the end of a Vector or it makes it the first value. If it is full, then it creates empty space at the end.
#include <iostream>
#include <vector>
#include <string>
int main()
{
vector<string> pokemon;
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
return 0;
}
I defined my vector as a <string> and then named it. I then usesd the <push_back> function for each element that I wanted to add to the array. Then I constructed a <for loop> to run through each element and print it to the screen.
Getting the Size
We can determine the size of a Vector easily. If the vector contains hundreds of elements, it is much easier to use the <size()> function. Let us modify our first example to give us more information.
#include <iostream>
#include <vector>
#include <string>
int main()
{
vector<string> pokemon;
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
int num = pokemon.size();
std::cout << std::endl;
std::cout << "The size of this Vector is : " << num;
return 0;
}
Here I use the <size()> function to let me know how large the Vector is. You add the <size()> function to the end of yourn Vector name.
This associates the function with the Vector you want to work on. Set up a variable like <num> so you can print the value to the screen.
Removing Elements
Sometimes you will want to reduce the size of your Vector. You will want to do this if you have old data, for example. To do this, you use the <pop_back> function.
#include <iostream>
#include <vector>
#include <string>
// Main function
int main()
{
vector<string> pokemon;
// Adding elements to the Vector
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
std::cout <<"Printing the original list of pokemon" << std::endl;
std::cout << std::endl;
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
int num = pokemon.size();
std::cout << std::endl;
std::cout << "The size of this Vector is : " << num << " pokemon" << std::endl;
std::cout << std::endl;
// removing the last value from the vector
pokemon.pop_back();
std::cout << std::endl;
std::cout <<"Printing the list for the 2nd time" << std::endl;
std::cout << std::endl;
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
int num2 = pokemon.size();
std::cout << std::endl;
std::cout << "The size of this Vector is now : " << num2 << " pokemon" << std::endl;
std::cout << std::endl;
// Adding the element again to the end
pokemon.push_back ("Crobat");
std::cout <<"Printing list for the 3rd time " << std::endl;
std::cout << std::endl;
// Checking and displaying size again
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
std::cout << std::endl;
int num3 = pokemon.size();
std::cout << "The size of this Vector is again : " << num3 << " pokemon" << std::endl;
std::cout << std::endl;
return 0;
}
This example is a continuation of the first two programs. I create my Vector and name it appropriately. Again, I add my values as elements and print to the screen. Now I use the <pop_back> function to remove the last element of the array.
I loop my way through the elements again so I have an accurate count. Then I print the new total of pokemon I have in my Vector.
The next step is adding my lost <Crobat> back to the array again. Can't let the poor fellow be all himself. After I use the <push_back> function again he is reunited with his friends.
I repeat the same steps as before. I loop my way through the elements again to get the correct total. I print to the screen again.
Emptying A Vector
There could be times when you want to completely clear a vector. It is done with the <clear()> function.
#include <iostream>
#include <vector>
#include <string>
// Main function
int main()
{
vector<string> pokemon;
// Adding elements to the Vector
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
std::cout <<"Printing the original list of pokemon" << std::endl;
std::cout << std::endl;
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
int num = pokemon.size();
std::cout << std::endl;
std::cout << "The size of this Vector is : " << num << " pokemon" << std::endl;
std::cout << std::endl;
// Clearing the vector
pokemon.clear();
std::cout << std::endl;
std::cout <<"Printing the list for the 2nd time" << std::endl;
std::cout << std::endl;
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
int num2 = pokemon.size();
std::cout << std::endl;
std::cout << "The size of this Vector is now : " << num2 << " pokemon" << std::endl;
std::cout << std::endl;
// Repopulating the elements
pokemon.push_back ("Pikachu");
pokemon.push_back ("Darkrai");
pokemon.push_back ("Golisopod");
pokemon.push_back ("Raichu");
pokemon.push_back ("Crobat");
std::cout <<"Printing list for the 3rd time " << std::endl;
std::cout << std::endl;
// Checking and displaying size again
for (int p=0; p<pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
std::cout << std::endl;
int num3 = pokemon.size();
std::cout << "The size of this Vector is again : " << num3 << " pokemon" << std::endl;
std::cout << std::endl;
return 0;
}
I create my Vector and name it. I add my elements and print them to the screen. Afterwards I use the <clear()> function to empty the contents of the array. When I print again it is just zero in my list.
There is nothign there. So I have to add them all back in again. After I do that I loop through the elements and once again print the entire list to the screen.
Reading From A File Into A Vector
Now lets do a more interesting example. I want to read data from a file. This is a task that you will do quite often as it is more useful. Since there is only data, there are no decisions to make.
To keep with the theme of pokemon, I created a text file in the same directory I am working out of with my editor. In this text file are ten names of pokemon, and each is on a separate line.
This program will read the file line by line and print what it finds to the screen. Remember to be in the same directory or you have to enter the full path to your file.
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
int main()
{
// variables
vector<string> pokemon;
std::ifstream PokemonFile;
std::string str;
// opening file to read from
PokemonFile.open("PokemonFile.txt");
// reading from file into the array
while (PokemonFile >> str)
pokemon.push_back(str);
// closing our file
PokemonFile.close();
// printing array values
for (int p = 0; p < pokemon.size(); p++)
{
std::cout << pokemon[p] << std::endl;
}
std::cout << endl;
return 0;
}
I created my Vector and named it accordingly. To read from a file, though, you have to do a little more set up. You need to create a variable to work with the <fstream> objects. The next task is to open up your file. I named mine <PokemonFile.txt>.
That is so I won't get confused, which happens easily I admit. All the more reason to name variables in meaningful ways. It really does help.
Next I create a loop to read each line of the file. As it reads each line, it will add them to the elements of the Vector array. Close the file and then just print your Vector and its elements.
We got the expected output, which is nice. You can keep adding to the file and saving it. Each time you run this program, it will read the current contents of the file.
In these examples I used all strings. Strings are harder to deal with but it is easy to find examples dealing with numbers. That is why I did it like this.
However, if you have numbers in your file, you can add them to the Vector array and do all kinds of math on them. That is very useful as well.
In this section we learned about the Vector container. Creating a Vector and getting data into are pretty easy. Just don’t get confused by the different syntax from a regular array.
We covered adding elements, removing elements, getting its size, emptying it out, and reading data from a file.
Basic Math With C++
C++ has several options for doing math. To do calculations, choose the operator you need. An operator is the symbol that tells the compiler what action to take. Let me show some examples. I am not going to spend a lot of time here, it's just basic math.
Math Operators
Here are the main mathematical operators:
- +
- -
- *
- /
- %
Now, here are the operators in action. I did it all in one example but that should be understandable for anyone.
#include <iostream>
int main()
{
// variables
int a {5};
int b {10};
int c,d,e,f,g=0;
// calculations
c = a + b;
d = a - b;
e = a * b;
f = b / a;
g = a % b;
// output
std::cout << "c = " << c << '\n';
std::cout << "d = " << d << '\n';
std::cout << "e = " << e << '\n';
std::cout << "f = " << f << '\n';
std::cout << "g = " << g << '\n';
return 0;
}
You can copy that into your editor and compile. Play with it if you need and if you don't understand something.
Min and Max Functions
These are useful functions later on. They are especially useful when you work on arrays, for example. These functions can analyze a series of numbers, like in an array, and output the largest and smallest of them. Below is a quick example.
#include <iostream>
int main()
{
double a {10.0};
double b {15.0};
double x {0.0};
double y {0.0};
x = max(a,b);
y = min(a,b);
std::cout << "The highest value is " << x << '\n';
std::cout << "The smallest value is " << y << '\n';
return 0;
}
As you can see, I hardcoded two numbers into variables. I then had the <min> and <max> functions compare the numbers and tell me which was the largest and smallest.
Pow and Sqrt Functions
More nice math can be accomplished with these functions. The <pow> function reads a number with its exponent and evaluates it. The <sqrt> function does the opposite, it takes the square root of a number. Let's look at another example.
#include <iostream>
#include <cmath>
int main()
{
double A {10.0};
double B {2.0};
double C = pow(A,B);
double D = sqrt(C);
std::cout << "A to the B power is " << C << '\n';
std::cout << "The square root of C is " << D << '\n';
return 0;
}
Please notice that we had to use a new header for the pow function. It is the <cmath> header and is required. I first declared a couple variables with some values. Then I created variables to hold the <pow> and <sqrt> functions. I then displayed them to the console screen.
Intro To Codeblocks With C++
Codeblocks is an IDE and in this section I want to show you how to get started using it with C++.
Introduction
Codeblocks is a popular IDE. It works very well on Linux or Windows. It supports projects, syntax highlighting, and debugging your code.
Installing Codeblocks
I recommend using your package manager. This will make it easy and usually a simple command to get it done. If you need a binary, you can go to their website. If you go to the Codeblocks site, you should just get the binary that includes the MinGW compiler. Both are installed at once.
Compiler
You will need a compiler to use with Codeblocks. If you are on Linux, you already have one installed. If you are on Windows, you can get one on the Codeblocks website as a complete package. When you open Codeblocks for the first time, you can select the compiler that you have installed. Click the button to the right to make it the default.
Using Single Files
You should create a folder to hold your single files. This will allow you to be more organized and be able to find them quickly. If you want to do a quick test, you can go to your menu and hit create.
- Select File
- Select New
- Select Empty File
This just creates a blank file for you to put some code in to see if you have everything setup correctly. Then you will go to your menu again to build and run.
- Select Build
- Select Build & Run
These options will build your files into object code and then run your code. You will see a small console screen with your output if everything went correctly.
Creating Projects
Again, I would create a folder to store your projects in. With this method, you will know where everything is at all times. To create a project, go to your menu and hit create project.
- Select File
- Select New
- Select Project
- Select Console Application
- Select C++
Now, you name your project and select the project folder you created earlier. Once this is done, you can add files to your project that you are working on. If this does not make sense yet, do not worry, you will know when you need to do this. So at first, you will just be working with single files to learn.
Conclusion
In this short guide, we talked about how to install Codeblocks and a compiler with it. Afterwards, I showed you how to create single files with some code in them. Then, we created a project, which will help us with larger tasks sometime in the future.