
How To Construct Loops in C++
Today's guide will be over loops in C++. I have been wanting to get to my latest C++ article for a while. So lets break out our favorite text editor and compiler and have some real fun today!
Table of Contents
Loops in C++
Loops in c++ will generally require some specific operators. These are simple and easy to memorize. They also use variables which can use inputs and outputs. The loops are used for mathematical expressions, testing, and reading from files. Creative uses abound, however, and you should indulge yourself whenever you think of a new use. So once you get the hang of things try and experiment some. Your usage and skills will grow the more you do that.
Types of Loops
There are a few different kinds of loops. Looping in C++ will require you to be proficient in all of them. Examples include while loops, the do-while loops and for loops. These loops are used in different scenarios. The one you choose will depend on your situation. When you get more experience it will be easier to recognize when to use each type of loop. For example you can even use them when you make decisions.
Incrementing and Decrementing Values
When you are looping in c++ you will have to modify variables and their values often. Increasing a value is called incrementing. Decreasing a value is called decrementing.
The increment symbol is [ ++ ]. The decrement symbol is [ -- ]. They should not be too hard to memorize.
Incrementing Example
This is a short example of incrementing a variable.

This is an example of a post-increment variable. That means the variable was read first and then incremented. You can also increment the variable first and then read the variable in to memory. If you want to do that, put ++ before the variable name. Nice and simple!
The While Loop
This is the standard first loop that you usually see. It is simple and has a lot of uses. A loop is a construct that helps you repeat actions. For example, if you have a text file that has 5,000 computer names in it, all on a separate line, you can use a loop to look at each line. Now it might not make sense why you would want to do that but you could sort these items, see how many started with a certain letter, or look for certain names within each line. The point is, it is much quicker to use a loop to do this than have a person look at each line individually.
A while loop is made of two main parts. They are the expression that is tested and the statement that is carried out if the test is successful. The expression must be evaluated to be true or false. This is called a boolean. Now the statement, which is executed, is any c++ statement that can be carried out.
What happens is the expression is tested. If it is true the loop continues to the statement block. It is then executed until the expression is false. This is what makes it a pre-test loop. That means its condition is tested before the loop takes place. A loop is always constructed so that it becomes false at some point. It is bad form to make loops that never become false by the way, so don't do this. Your program also will not work right if a loop never becomes false. This is a standard way to construct loops in c++.
Example While Loops
Lets go to an example now.
https://gist.github.com/jahlelin/be697086e0c1a725b4c1418625dd5f3c
This is just basic while loop. I asked the loop to print to screen the numbers from 1 to 20. The (num < 21) ensures that the loop does not go past 20. As you can see in the loop, I also asked the variable (num) to increment by +1 each time the loop executed. There isn't much more to it than that. Here is what the output looks like.
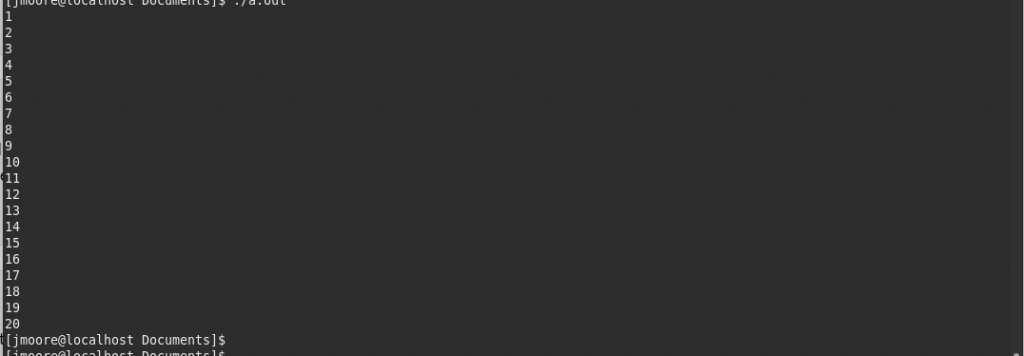
Lets do another example where we decrement a variable after each loop execution.
https://gist.github.com/jahlelin/5260d78249a25a8d5e3b36123994e4a8
In this short example I just decrement the variable (num) from 25 to 15. During the loop I decreased the value of the variable by 1. That is called decrementing. Let me show the output below so you can see.
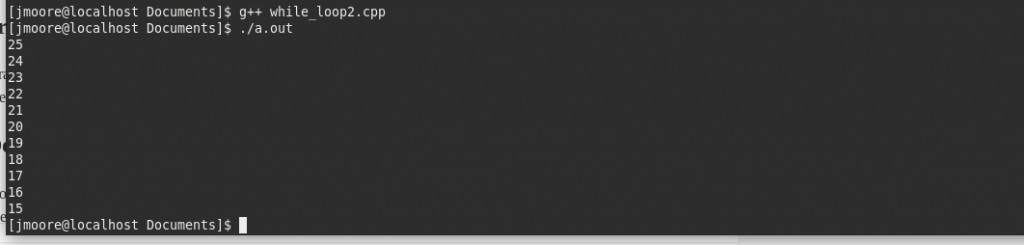
There, that is nice and easy.
Variable Counters
Counters go hand in hand with loops. The reason is that sometimes we want to know how many iterations a loop has gone through. Another scenario is that sometimes we want a loop to only run a certain number of times. That is why counters are important. They are just variables that are incremented or decremented when a loop executes. You will see I use them to control the loops I use.
Do-While Loop
A do-while loop is the opposite of a while loop. What I mean by this is that its statement is done before its condition is tested. This makes it a post-test loop. This loop will always make one iteration because of this. So when you are writing a function or whole program, if you need your loop to execute at least once then use a do-while loop. A unique part of this loop is that it always ends with a semicolon, which makes it different than a straight while loop. That makes it confusing to people first learning too. Lets look at an example.
https://gist.github.com/jahlelin/5880f5f628d6c23861b9af9a9339b741
Everything looks mostly the same except the format of the loop. That would be correct too. This is how a do_while loop works and it is also a simple way to construct loops in c++. In this loop you can see we take an action first and then evaluate whether our condition is true. I then increment the variable in the loop. The loop then repeats as long as our condition is true. Here is the output for this very simple loop if anyone is curious.

The For Loop
When you know the exact number of times a loop should execute then a for loop is an even better choice. A for loop will initialize a counter to its starting value. It will test the counter to its max value. Finally it will update the counter after each iteration of the loop. It is a pre-test loop which means it does its comparisons before going through the rest of the loop. You should use a for loop whenever you need a variable initialized or know how many times you want the loop to run.
There are several tricks you can do with for loops. You can update the counter directly to better control your loop. Going forward or backward is no problem of course. Defining and initializing your main variable in the loop is often a good idea. So lets try one of these out.
https://gist.github.com/jahlelin/6597f8efac0361556450f4f068401ebf
So this one is slightly different. The initialization, condition, and updtate of the variable is done on all one line. You then just have whatever valid c++ statement down below. Here is the resulting output when I compiled and ran it.
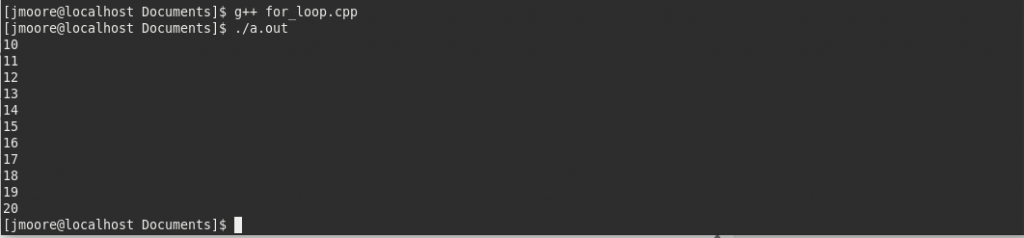
So nice and simple.
Working With Accumulators
An accumulator is the sum of numbers every time a loop iterates. This is very common in loops and is important when constructing loops in c++. This could be sales totals, number of laps in a race, or how many cards you have discarded in a game of pokemon. Its important. In each iteration of the loop the accumulator will contain the total from the numbers read by the loop.
Conclusion
Loops in c++ have many uses. They are used constantly but at a more complicated level of course. You can evaluate thousands or millions of numbers or letters in an instant. Much can be done when you can look at that much data quickly. Constructing loops in c++ is easy, you just have to figure out which loop you want to work with. Some people use all of them but others will try and use one loop as much as possible. Either can work but ultimately you want to do what will make your program work the best.
I hope you enjoyed this article. If you did please share it on your social media because I will be writing more. Have a great day!