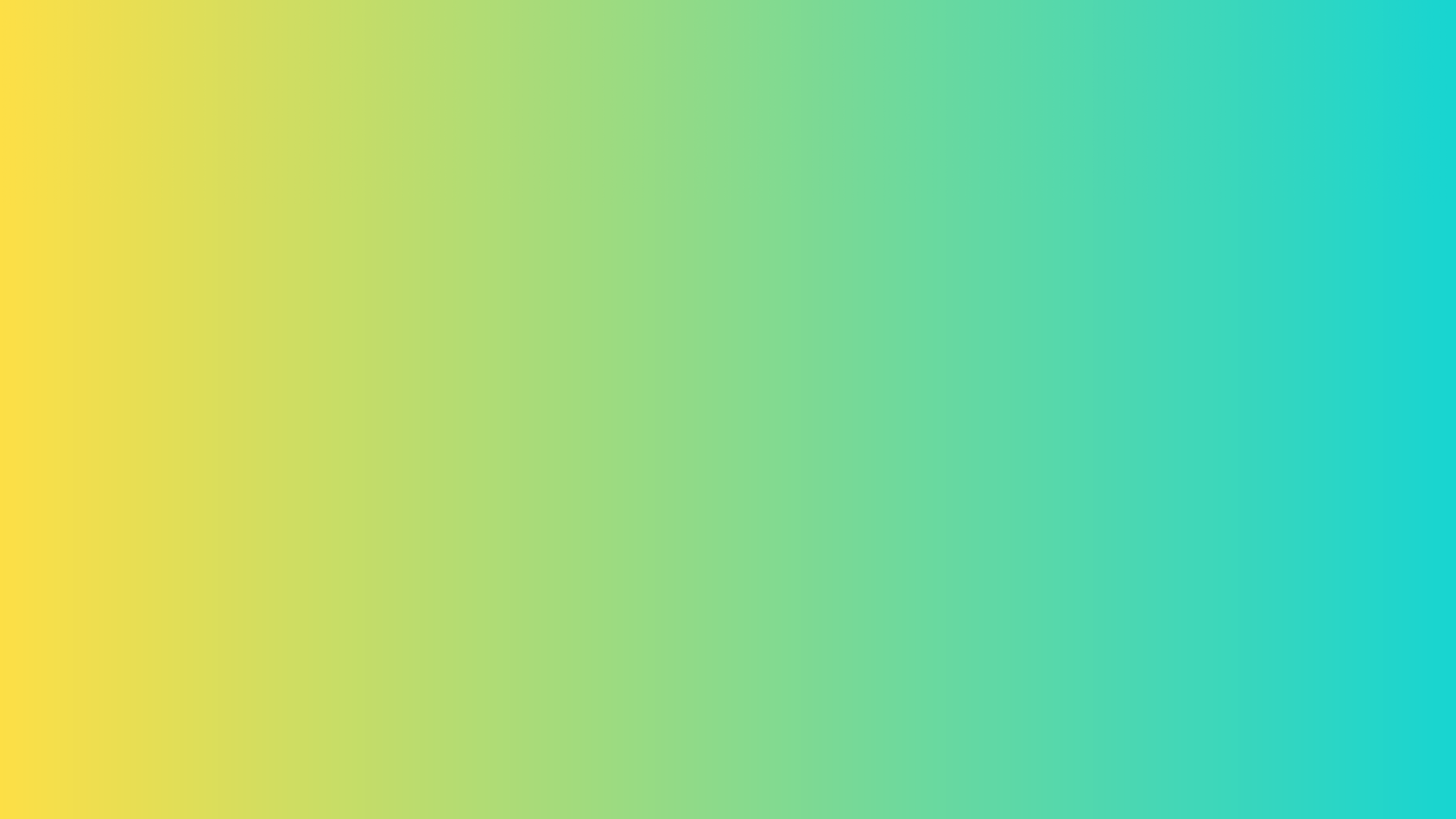
Making Loops In C++
Making loops in c++ is a fundamental skill just like it is in other languages. C++ has several options that I will go over.
Introduction
We often need to run a piece of code many times to get results that we need. Looping is how this is done. In this section, I will talk about the different types of loops and show some simple examples.
Incrementing and Decrementing
Variables are increased or decreased as needed. You will often have a counter variable for this purpose. These counter variables are useful in loops. They keep track of how many times the loop has executed. The “++” and “--” operators are used to increment and decrement variables.
#include <iostream>
int main()
{
int counter = 1;
std::cout << “Our variable is currently equal to “ << counter << std::endl;
counter++;
std::cout << “We incremented our variable, and it is now “ << counter << std::endl;
counter—;
std::cout << “Now we decremented our variable and it is again “ << counter << std::endl;
return 0;
}
Looping
For the beginner, looping is where a piece of code repeats. It will repeat until it meets a condition to stop. You, as the programmer, will give it this condition. If you do not give it a condition to stop, it will run forever. This is bad. It is an infinite loop. You normally do not want this.
Therefore, a loop is a code structure than repeats a group of statements. C++ has the “while” loop, “do-while” loop, and the “for” loop.
Loops are where we use counter variables and they are important in these structures.
While Loop
A while loop executes and keeps running as long as its test condition remains true. It comprises two parts. One part is where it tests to see if its condition is true. The other part is the code that gets repeated.
The condition to be tested has to be a true expression. Again, it only runs if it is true.
#include <iostream>
int main()
{
int var = 10;
while (var <=20)
{
std::cout << var << std::endl;
var++;
}
return 0;
}
At the beginning of our program, we defined and initialized our variable. We then set our condition to be tested and put this in our while loop. It tests the variable to see if it is less than or equal to 20. When it is, variable value prints on the screen.
The loop increments the variable each time the loop runs. When the variable reaches the value of 21, the loop stops because the condition I set is no longer true. Each time the loop runs, it is an iteration.
If you have noticed, the while loop tests its condition before it runs. This means the loop will never run if the condition is false from the start. It is an important consideration if you need the loop to run at least once.
Let us now do some decrementing in a loop. It works the same way. We test the condition to see if is true. If it is, then the loop runs an iteration. This continues until the condition is false.
#include <iostream>
int main()
{
int var = 50;
while (var >=10)
{
std::cout << var << std::endl;
var —;
}
return 0;
}
While loops are handy for working with numbers and you can do a lot with them. Here is another common example, where I square several numbers. The condition in the while structure tells it when to stop.
#include <iostream>
int main()
{
int var = 5;
std::cout << “variable\tsquared” << std::endl;
while (var <=25)
{
std::cout << var << “\t\t” << (var*var) << std::endl;
var++;
}
return 0;
}
Do-While Loop
This type of loop is the opposite of the while loop. It is because it tests its condition after the loop runs once. This loop structure is useful in the right situation. It is useful when you need the loop to run at least once. This is important to remember.
#include <iostream>
int main()
{
int counter = 0;
do
{
std::cout << counter << std::endl;
counter++;
while (counter <=20)
}
return 0;
}
This program takes the opposite method of counting numbers. It is just as good as the regular while loop, it just depends on your situation. There will be times when it is best for one or the other. Be flexible in your coding and don’t get stuck doing things only one way.
The For Loop
This is another type of loop and it is an excellent choice when you know how many times a loop must iterate. The two previous loop structures only run as long as they test true. It could be zero or infinite many times. The For Loop will execute a certain number of times and that is it. You dictate how many times that is.
The For Loop is unique because it has a few requirements. It has to initialize a counter variable, it must do a test, and it has to update the counter variable. Let us do an example to see how it works.
#include <iostream>
int main()
{
for (int counter = 0; counter <=10; counter++)
{
std::cout << counter << std::endl;
}
return 0;
}
The top line of the For Loop includes the initialization, the test, and an update. This processes work the same asin the other loops. They are just in one line now. Notice the lack of semi-colon after the counter update expression. This often trips people up at the beginning.
The counter variable is used to control how many times the loop runs. This happens until the test condition is false. Then the loop ends.
The For Loop is versatile. You should use it when it makes sense to do so. For example, use it when you need to initialize a variable or when you need to update a counter. It is ideal for these situations.
Keep Running Total
Keeping a running total is very useful. You can keep track of sales, your dps in your favorite mmo, or even your stock gains. The uses are endless and only reliant on your imagination.
A type of variable that is used for this purpose is an accumulator. It is used to keep a running total. It reads each number and adds it to the total. Most of the time the accumulator is initialized to zero, but this could change depending on your needs.
#include <iostream>
int main()
{
int total = 0;
int days;
int coffees;
std::cout << “How many days did you have coffee this week? “ << std::endl;
std::cin >> days;
for (int counter=1; counter <= days; counter++)
{
std::cout << “How many coffees did you have today “ << std::endl;
std::cin >> coffees;
total = total+coffees;
}
std::cout << “You have had “ << total << “ coffees this week” << std::endl;
return 0;
}
Sentinel Values
A sentinel value is an entry that marks the end of a list of entries. It says to stop doing what you're doing. We use this in loops when you do not know how many items there are to work with. In a For Loop, for example, you tell the loop how many times to run and then do something. If you don’t know how many times the loop is supposed to run, you use a sentinel value.
This gives your loop and program a lot of flexibility. We just don’t always know how many times our loop is supposed to iterate. So, we just ask the user to enter a sentinel value when their task is done.
#include <iostream>
int main()
{
int hour = 1;
int people;
int total=0;
std::cout << “Enter number of patrons in coffee shop by the hour:” << std::endl;
std::cout << “Enter -1 to stop” << std::endl;
std::cout << “Enter number of people:” << std::endl;
std::cin >> people;
while (people != -1)
{
total=total+people;
hour++;
std::cout << “Enter number of people:” << std::endl;
std::cin >> people;
}
std::cout << “The total is “ << total << “ people” << std::endl;
return 0;
}
In this program, I do not know how many hours I plan to stay in the coffee shop. Coffee is too good to just leave! Anyway, I just set up a sentinel value so I can tally my figures anytime I want. When I enter the sentinel value, the loop stops.
Indenting Loops
There is nothing technically wrong if you do not indent your loop code. However, it is a good idea if your program is more than a few lines. I’ll admit I am not the most consistent. In longer pieces of code, I will indent. It makes everything more readable. There are time I do not. If something is extremely short and won’t be used anywhere, I often don’t. If in doubt, just indent.
Pretest and Posttest Loops
This can be pretty important to understand. In a pretest loop, it will not run until your condition returns true. It is the opposite in a posttest loop. In a posttest loop, the loop will run once and then test for a true condition.
In some loops, you want your loop to run once, no matter what. This means you need to use a posttest loop so it will run once and then test for its condition.
Conditional Statements
Conditional statements are run if they meet the correct condition. We set this condition up in the loop definition. You set the definition of the loop. So, if the condition is met, the statements inside the loop will run.
Difference Between Loops
The While Loop is a pretest loop and its statements will only run if its condition is true. We evaluate the condition before the loop runs once. In a Do-While Loop, the loop code is run once and then the condition is tested for the first time. This makes it a posttest loop. It is useful when you need code to run once, no matter what.
Conclusion
In this section we have briefly talked about variables inside loops. These variables can be increased or decreased as needed. This lets us control the loops and set condition for stopping the loop.
We also discussed the different types of loops and their different benefits. Since there are both pretest and posttest loops, each has advantages. Those are While Loops and Do-While Loops. then we talked about For Loops. These are great when you want a loop to run an exact number of time before it terminates.
Then we talked about keeping a running total. This technique is great for certain tasks, like counting how many times a loop runs. Sometimes we don’t know how many times we need it to run, but we want to know the results afterwards.
Thanks For Reading
Thank you for reading this, I really appreciate it.
If you would like to join my newsletter, you can do so here:
If you need a suggestion on what to read next, then try these articles: