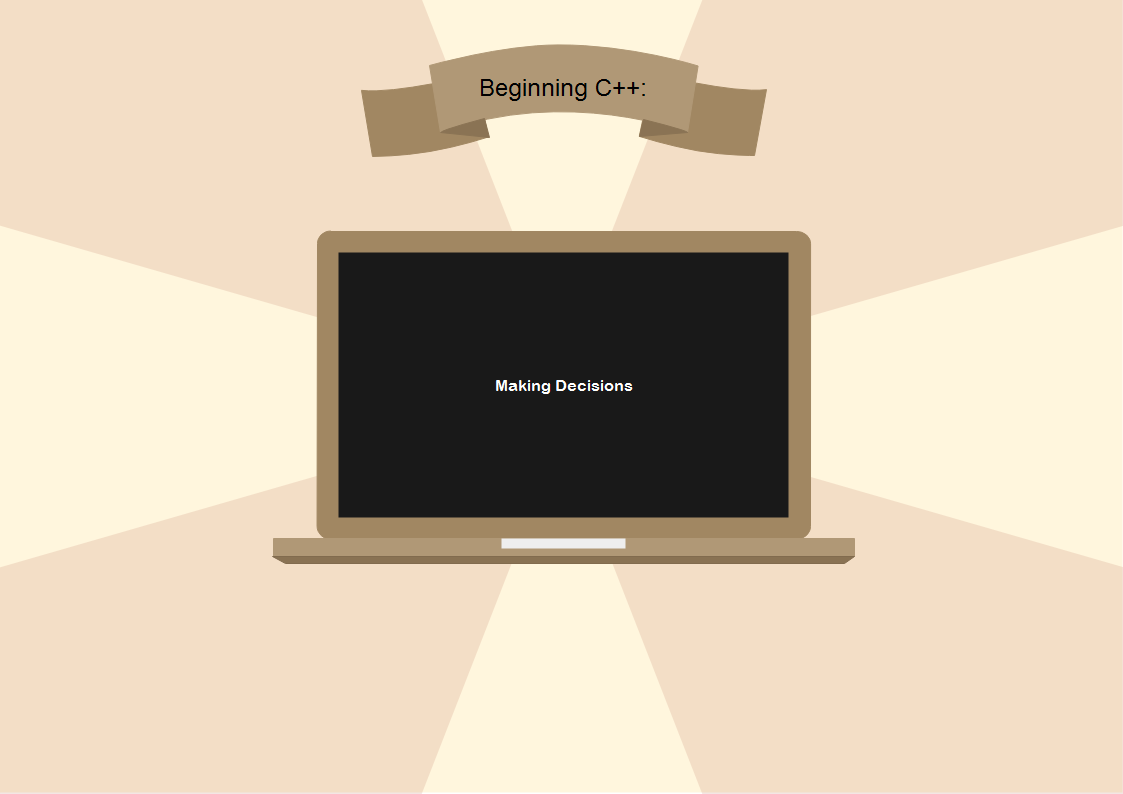
Beginning C++: Making Decisions
Making decisions is one of the fundamental activities in any program. Data has to be compared and conditions will have to be evaluated. This is usually the second stage in learning how to program. C++ is just our tool. Regardless, this is one of those concepts that you need to know really well. It will be applied everywhere.
Making Decisions
If you missed my first article please read it first : http://aindien.com/beginning-c-input-output-basics/
Wanting articles about IT then try this: http://aindien.com/how-to-make-the-jump-to-system-administrator/
This is one of the core topics in programming and something you will use a lot. This should be ideal for the beginner who is just learning about programming. If you missed my first article on C++ and how to get started then try it here.
Making decisions specifically means that you will be comparing data. This will be done using either numeric or character operators. Numeric data is compared using these operators:
- < less than
- > greater than
- <= less than or equal to
- >= greater than or equal to
- == equal to
- != not equal to
Use these to compare numbers or expressions. They work left to right. Now we can ask for input and make a decision.
[If] Statement: Your First Decision
Using [if] lets you make decisions in your statements. They will only execute [if] a condition is met. That is how [if] works.
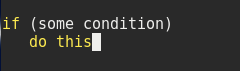
This is easy so don't worry about it. If the condition is met then the line after will execute. If the condition is not met then the program will just skip right by it and move to the rest of your program. It's that easy. Let's do a quick and easy example.
Example 1: Making Decisions With An If Statement

This is pretty straight forward. It is our same basic framework as before. I ask a question. Then I add 2 different conditions. I ask how many movies you own. I then make a condition for more than a 100 and less than or equal to a 100. Look and see how the [if] statement was used there. Give it a condition and then do something. It is simple. I then wanted to do a second condition so you could see what happens if the condition was not met the first time. I did not want my program to end and just do nothing so that is why I added the second condition. Plus its another example so should help seeing it again. Here is some of the output from that.

You can see from the code that my condition was 100. Now look at the output above. I made sure to have an outcome for less than, equal to, and greater than 100. That is an important point to remember. When you have conditions you need to cover all the possibilities.
The [if] statement can also work more complicated. It is not just limited to 1 statement if its condition is met. It can do a series of statements and even other things. We can modify our program to do a little more. Notice how the [if] statement changes.
Example 2: Making Decisions With Multiple If Statements

Take note of the braces after each [if] statement. There is an opening and closing brace for each decision. They both do different things depending on whether the condition was met. What they can do is only limited by your imagination. Here is the output for both decisions.
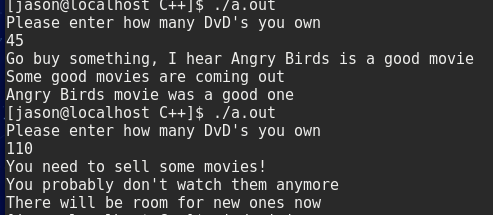
You will notice that for each decision that is made, a different block of statements was executed.
If/Else Statement
There is also a separate [if] structure for each block of statements. There is no need to do that except when first learning about [if]. With this structure you can use [if] then if the condition is met do one thing. If the condition is not met then it will do the other block of statements. It looks like this:
Example 3: Making Decisions With If/Else Statement
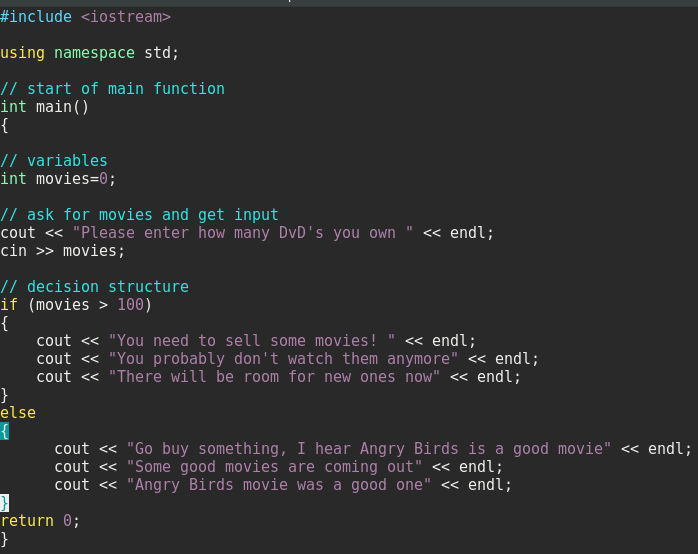
There is only 1 [if] structure in this version. Notice the [if] with a condition, then braces enclosing your condition, then the [else] statement, then braces enclosing another block of statements that execute if the condition was not met. There are semi-colons at the end of each statement in the statement blocks. Also see the opening and closing braces for each statement block. No need for output as it is easy to see what it will look like.
Nested If Statements
Now if 1 decision depends on another you can use nested statements. Lets modify our current program some to do this.
Example 4: Making Decisions With Nested Structures

Look at how this program is different. I have a nested [if] structure right there in the middle. I asked for y or n answers too. That meant I had to declare [char] variables which take the input of any key press.
Then you will notice I asked my question. Then gave the conditions. Otherwise they might type out yes or no. They can still do that but we will account for that later. Afterwards I got my input and put it in a variable. Nice and simple.
The decision structure looks different too as it is now nested. This means I have a condition within a condition. In logic terms, this means both have to be right to be true over all. This is important to remember.
Last thing I want to mention is to indent your structures to set them apart from other things. It makes it easier for you and your professor to read. Plus it is just good coding style to do it.
If/Else/If Structures
This is another type of [if] statement. Frankly its kind of weird but it has its uses at times. It is best used when you can have a series of conditions. It will terminate as soon as the first condition is met. This is unlike my last example where it went through all the conditions anyway. Let me think of a good example to show this. Well i couldn't think of a good idea, but this idea should suffice. My conditions will be based on the length of this article. I will show you how it works.
Example 5: Making Decisions From A Series Of Conditions

Yeah this idea was cheesy I know. It was all I could think of. Anyway, as you can see, there can be as many conditions that you want in a structure like this. That is its advantage. If you have a huge series of things to ask then it is best to use this type of decision structure.
Notice how all the statements are aligned. This makes it easier to read and debug when you make typos and stuff. Look at how the end of the decision terminated. You use [else if] on everything but the first and last of the structure. Make sure your logic is right too by testing.
Conclusion
Well that will conclude this topic. There is a lot more you can do with decisions and operators. It of usually quirky and very situational. I did not want to include it for that reason. The idea for this article was to be clear and show how to do the main topics in my own way. Then, I did the types of decisions that I thought were the most useful to beginners to help them learn. I hope this will be fun or at least helpful for anyone who reads this.