
Beginning C++ : Input and Output Basics
This is my guide for students just starting with beginning C++. Programming in general is not the easiest thing to do. With just a little effort it can be easy to pick up. I cover the essential basics here with lots of examples.
Beginning C++
This is a guide on learning C++ and how to program. The two are not the same. Learning to program is the fundamentals behind problem solving. You will eventually use a variety of techniques and algorithms. You will learn to think long and hard about how to describe the problem. Once you do that you are well on your way to figuring out what to do. That is the whole purpose to programming. We solve problems. Some are hard and others are easy. To accomplish this we have to use a computer language such as C++.
Reason Why C++
C++ is an old language. It is a very good one though and it is still very actively developed. It is sort of low level but not as much as it could be and it is extremely powerful. The trend these days is to gravitate towards newer and higher level languages. These languages abstract away a lot of the details that C++ provides. Sometimes this is acceptable but if you really want to learn programming then you need a language like this.
If you know how to program and you just have an easy task I can certainly understand using a high level language. I think it is a crutch, though. Using only high level languages will eventually stop your growth as a programmer. That is why C++ is often used as a first language for beginners. Learning C++ will teach you how a computer works. This is vital to tackling harder problems later on.
Starting Out
I am going to assume you have some basic computer skills. Knowing some basics will help you understand how a program is built. Prior programming will not be necessary though. You will need to have a compiler installed. I will leave this part up to you. There are many choices to choose from. Choose something simple that you can understand and operate. When you have done this continue on to the beginning C++ guide. I want to note that compilers come standard with any Linux or BSD distribution. They are designed for programmers and include a lot of great tools that are free and continually updated. I recommend going this route.
Parts of a Program
Essentially there are three parts of every simple program. I am dumbing this down as much as possible at first. Large complex problems are a bit different. For now, though, these simple programs we will start with have 3 parts to them.
- Input
- Processing
- Output
Input
Getting input from a user or other source is usually one of the first things you will do. Often you will first get some data so you can use it.
Processing
Once you have this data you will then want to do something with it. That is why it is called processing. You do such things as calculations and sorting of your data.
Output
Once you have processed your data you will need to display it somehow. This is outputting it somewhere. It could be a monitor, printer, or even another file. You will learn all of these eventually. If you have done a calculation then you can put it on screen to see the results.
Planning Your Program
Before you start just randomly writing code, you need to have a plan. Do whatever works for you. It can be a nice flowchart or the steps written down somewhere. Think of it like an outline for a research paper and since you would not write a serious paper without planning it out then you should not go without this step here either. You can use numbers and letters to organize your steps. It is just a guide written down for you to follow. A common first problem is to figure out the area of some space. Let's just call it a rectangle to make it simple and go from there. Here are some steps written down that would help me solve this problem.
Steps To Write This Program
- Calculate the area in square meters(goal)
- Get input which will be the dimensions(input)
- Use the dimensions to calculate the area(processing)
- Output the area to the screen so I can see what it is(output)
This is a rudimentary list but would serve for this simple program. I could add C++ syntax and tasks that were specific to the program as well. You can also see that my outline corresponds to the parts of a program that I listed previously. Each one of those tasks I can break down to sub tasks too to expand my outline and make it more detailed. In a larger program you would want to do that.
Basic C++ Framework
All C++ programs have the same basic structure to an extent. They have one or more header files, defining the namespace, and a main function. I will explain more about these as they come up and you need to know about them. Here is an example of what a basic program looks like.
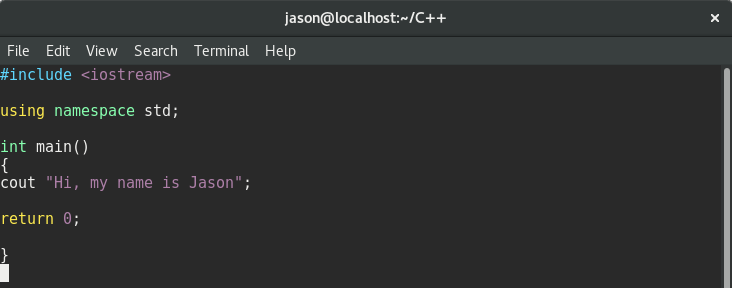
There it is. It is nice and simple. I decided I wanted to output a message onto the screen and that is how you do it. Programs start from here and just get more complicated as they do more.
Points To Remember Are
- Header files(<iostream>) go at the top.
- Put your namespace function next.
- Every program has a main function and your code goes into it.
- C++ is a case sensitive language.
- Make sure you have a closing brace for every opening brace.
- C++ statements end with a semi-colon.
- Put a return 0 at end of main function until you have a reason to do otherwise.
To me this is not really a complete program because it does not really do anything except print to the screen. As you can see there is no input or processing. However, in beginning C++ classes it is traditional to start with something like this. I will use this framework to expand to more useful code that does things.
Sample problem 1
Ok let's just dive right in. There are a lot of details but if i told you about them you would forget anyway. So let us work on a problem and use that association to remember what is necessary. For this first problem you have a bought a house. You want to put carpet in your bedroom. Saving money is a priority since you just bought a house. So doing it yourself is the plan. Then you have to figure out how much carpet to buy. The first step is to figure out my plan. We start with our outline. #1 in our outline is always state your goal.
Steps For Problem 1
- Calculate the amount of carpet you need.
- Get your input.
- Assign some variables.
- Use a prompt to ask for dimensions.
- Find length of room.
- Find width of room.
- Do your calculations.
- Well: area=length*width.
- Have some output.
- Printing to the screen is probably fine for now.
That list looks like it will get me started and be enough to finish it. It doesn't have to be perfect. It just has to be able to work. You can fine tune your code afterwards. So now you just open your code editor of choice and start writing C++ following the outline you made. I will do mine and then explain it.
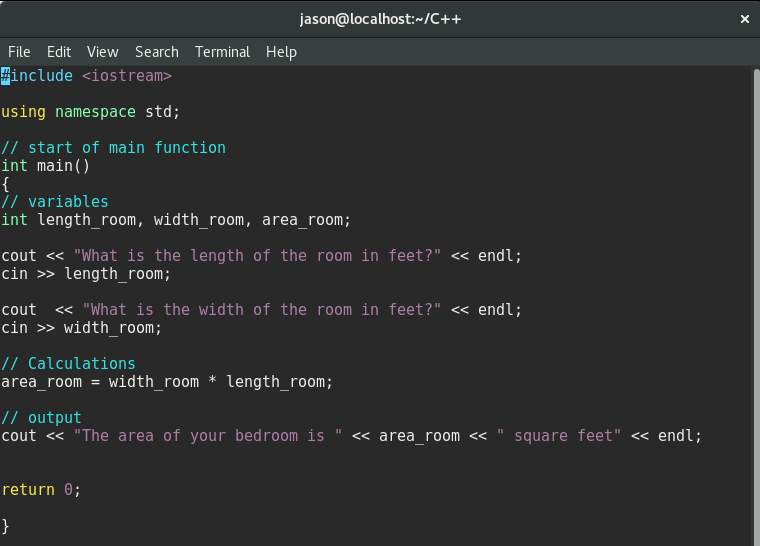
Here is out short little program to calculate the area of our bedroom. There is a lot more we can do with it but we will worry about that later. Let me explain this for those of you who have never done this before.
Key Points
- #include <iostream> --this is our header file. A header file has a bunch of code in it that lets us use commands like [cin] and [cout].
- //--every line that starts with these slashes are a comment. They are not compiled and are your notes in the program.
- int- this is the type of variable I am using. It stands for integer and it is a whole number.
- variable--these are the locations in memory I have named to hold my dimensions.
- cout--this is the function that displays text as output.
- cin--this is the function that gets input from the user or another source.
- // calculations--this is my formula and is the rule as to how the computer uses my variables.
If you have sat down and typed this out also or your own version of it then you can see how all of this interacts. It will make more sense like that too. In later sections I will go over more details about how variables and data types work.
#include <iostream>
This will usually be your first header file. It is the basic header file in all beginning C++ programs. It includes all of the most basic commands and functions that you will use. Functions like [cout] and [cin] are included in this. Any header file, like this one, is made up of a bunch of functions coded so that you just have to include the header file like we did above in order to use those functions. If we did not then we would have to write the definition of every function that we use.
That is how custom functions that we will eventually write get into our programs. You can also write your own header files too once you have a collection of functions that you like to use that you have written yourself.
//-Comments
Any line starting with [//] is a comment. They can also be inserted in the middle of statements or lines. Comments are exactly what they say they are. They are basically notes to yourself and any other programmer that might look at your code. It is a good idea to use them any time you can explain what is happening in your program. Use them liberally. When you come back to a program you will probably not remember the logic of it at first. That is what comments are for. They are used to explain what you were trying to do.
Int
[Int] stands for integer. It is a data type used in beginning C++ code and a number without decimals. Only use it when you do not want decimals or there is no possibility of decimals being in your calculation. There are other data types like string, float, and double as well. They each have their own characteristics and uses. I will explain more as we use them because I think that is the best way to teach them.
Variable
A variable is a named location in memory. It is a holder or container of information. It will hold the dimensions I put in there. In this case I use them for my dimensions. I then refer to them when doing my calculations. There are many rules regarding them but the main things to remember is have them make sense for their use. I could have used [a] as the variable name but that would not make sense. If someone looked at my code, like your professor, then they would not know what it would stand for. You could then therefore get marked off on your grade.
You see how I used my variables. I made them clear as to what their purpose was. I made them 2 words to help me explain them clearly. You could use 1 word but that is my preference. The point is that they should make sense without being too long to type.
Cout
In beginning C++ programs this is often the preferred way to display data on the screen. You can think of it as C++(for c) and output(for out). It is your output. Whether that means to the screen, a printer, or a file is up to you. You just type [cout] then [<<](stream insertion operator I think) and then ["your message"] in quotes as I have done there.
You can do more than one [<<] per line and you can mix them into regular sentences too. [Cout] is often used as a prompt which means to ask for information. Here I use it to ask for the dimensions. That is what appears on screen as a question. That is what I mean as a prompt. I then display my answers at the end of the program with [cout] too. It is as very versatile function.
Cin
[Cin] is how you get your input. After I ask a question on the screen, using [cout], I want to put the answer into a variable. This is done when asking for the dimensions. I ask for them and whatever is typed after that, if the correct data type, goes into the variable. Then as I explained above, I just use the variables in my calculations. Notice the [>>] after [Cin]. They are in opposite directions of the [cout] function. That is because data is coming in.
Calculations
This is the last main section of my small program. It could also be called the processing section. Up until this point I have asked for data. Then I put the data into variables. Now in this section I do something with the data. I could do lots of different things here. It depends on the program. We are doing a calculation here so I will define a formula and use the variables in it. Then I just display my results. I could run this program or anyone else could too.
After Program Thoughts
Since we are now finished with our first program I just wanted to mention a few things:
- We could have written that many ways. C++ is very flexible and there are complicated ways of doing the same thing.
- The structure of our program could be very different. You can do things like prompt differently, ask for all your dimensions at once, and formatted it.
- You can also do a whole lot more with it when you develop your skills and imagination. Those things will all come later.
More Input Techniques
When you want to capture something to put in a variable that you do not want to do calculations with, you need different types of data types. Input such as names, sentences, or even zip codes use a different type. Lets do another program that will not do anything significant but will hopefully showcase more things we can do.
Char Data Type
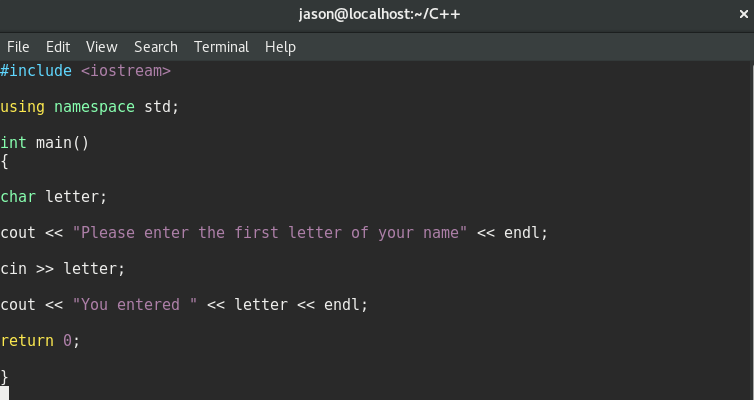
This uses the [char] data type. It is useful for many things. This is just a basic example but it shows you how to use it. For example, if you want a yes or no answer it would be useful to use this to get it. Just give the choices as y or n and you can continue with your program.
Floating-Point Data Type
This data type is used for calculations or anything where a whole number will not do. Lets try calculating the area of a circle and see an example of the floating-point data type in action.

This program does 1 thing. It calculates the area of a circle. I use another header file called [cmath] that is required for the [pow] function. you can see how the float data type works. It will give a decimal value if there is one from your formula. The [double] data type is used the same way. It can just hold larger values.
Bool Data Type
The [bool] data type is an interesting one. Variables with this type can be either true or false. A false boolean has a value of 0. True is then represented by 1. These are the standard on and off values associated with a computer.
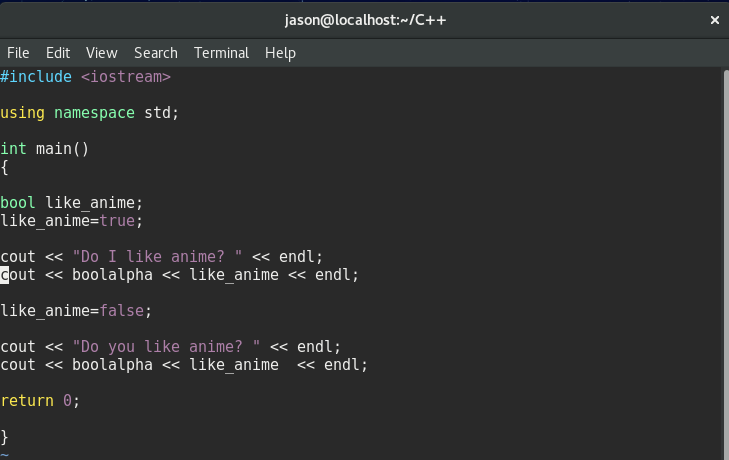
This is simple but shows you the versatility of [bool] and [boolalpha]. I started this program like usual. Using the [bool] data type in this beginning c++ tutorial, I show you how it can be set to 0 or 1. 0 is false and 1 is true. I then use the [boolalpha] flag right before I [cout] my variable. By doing this, I make it return true or false, depending on its value. This is a great data type. It uses very little memory. Later on when you want to make your program faster and quicker remember the [bool]. It is very good to use.
Scope Of Variables
The scope is an important concept when designing your program. This means what has access to your variable. You need to remember to define your variable before you can use it. That is what this concept is all about and what you need to remember at first. You can create a global variable outside of your main function and everything has access to it. If you define the same variable inside your function then only that function has access to it. Just something to remember.
Formatting Your Output
You already know that [cout] can be used to output the results of a program or even a prompt onto the screen. It should be apparent that you can also adjust what [cout] does to fit your needs. Aligning numbers into columns is a very simple task. There is a way to control how many digits appear after a decimal. Controlling the width of your statement is also very easy. To do this we will want to use the [iomanip] header file. It will be included at the top with the other header files.
Here is a short piece of code to demonstrate.
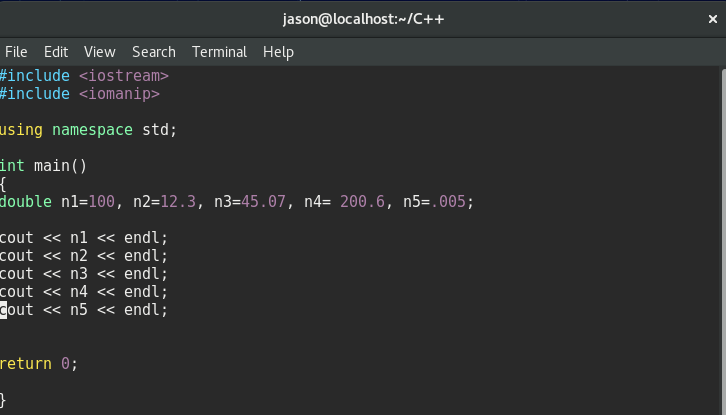
While the code itself appears completely ordinary, I wanted to draw attention to the default output. Here is the output of this code.

You can see here that nothing is aligned. Decimals are everywhere. It makes it hard to read in fact. Let me show you some things to do about that.
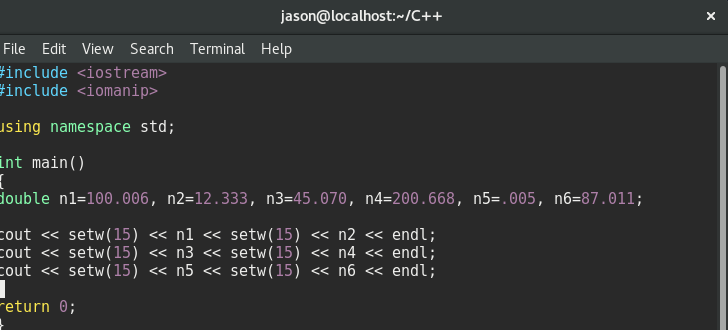
This looks a little better.

With using [setw] we can right justify any way we would like. Sometimes this may be exactly what we want. If, however, we want to control how many digits appear after the decimal we can use [setprecision] and [fixed].

Notice the extra [cout] statement with both [setprecision(2)] and [fixed] in it. [setprecision] controls how many digits after the decimal point are shown. I chose 2 here. [Fixed] always shows a decimal point so that is useful as well. Here is the output.

That is much better now. You can be very creative with how you want things to look. In beginning c++ formatting there is much you can do and still much to learn. Lastly, you can also control whether your output is left or right justified. By default it is right justified but you can easily change that to left and then right again if you wanted to.

Here I just added [left] to the other formatting options.

There you have everything left justified. Easy to do. If you want everything right justified again just put [right] where you have [left] in the code.
Conclusion
C++ has a lot of flexibility. While learning to program it is best to concentrate on as few ways of doing things as possible. Get comfortable with one way for doing a certain task first so you will understand. After that you can branch out and experiment. That way you get the concepts down first and not get bogged down in the particulars of whatever language you are using. Beginning C++ has a myriad of ways to do every task. You will notice I only showed one way to do any particular task. I thought those were the most basic. You will learn more on your own which is fine. Just remember to get these basics down.
Once you do, other things will be easier to pick up down the road. Hope you all enjoyed this guide. It took a while to put together but if it helped someone get started or kindle a passion for programming then it was all worth it.