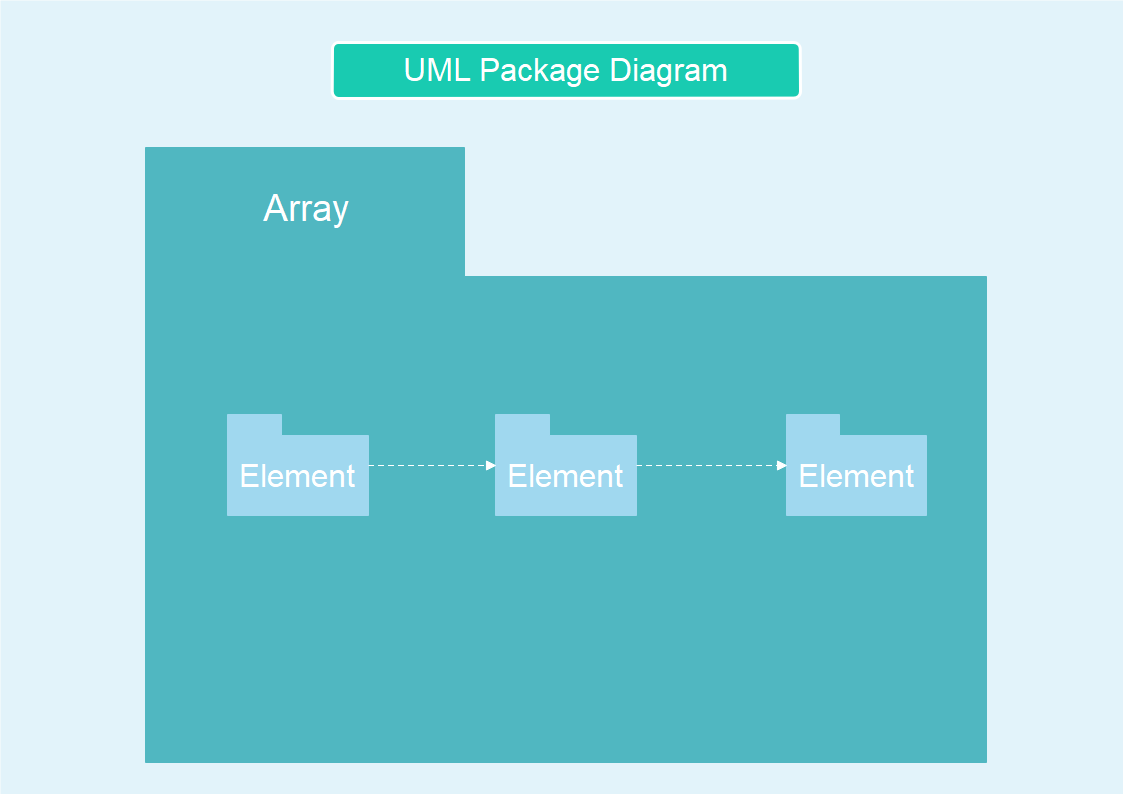
How To Start With Arrays In C++
This article is over arrays in c++ and how to get started with them. I will show you how to initialize them and then examples of some basic tasks.
Introduction
An array is a variable of sorts. It is a construct that can be used like a variable but hold many values instead of just one. These values must all be the same data type. Data types are short, long, double, float, bool, and strings. Those are just the main examples, I did not list every data type. So, in short, an array lets you hold and work with multiple values of the same data type.
An array looks like this:
int list[10];
Arrays must be of the same data type. Any of the legal data types in C++ will work. In my first example, [int] is an integer, [list] is the array name, and [10] means there are ten elements in the array. The size of the array must be a positive integer, no decimals allowed.
Memory
The memory used by an array depends on the data type and the number of elements. The more of each, the greater the memory required. So you will have to make a decision and decide how large you want your array to be.
Accessing Elements
Remember, an array is just a special type of variable. Don’t let it confuse you. This special variable is divided up into many other variables. That is best way to think of it. The discrete elements will each have its own subscript. You will use the subscripts to access the elements of the array.
A key point in arrays and their subscripts is how to use the subscripts. A [10] element array will be numbered from 0-9. A [25] element array will be numbered 0-24. Hopefully that is clear.
Accessing the elements to use them is easy enough. It is done like this:
list[5] = 75;
In this example, element [5] has been assigned the value of 75. It is important to know that you can’t use an element if it does not have a value. So you have to give elements values in order to use them as variables.
Giving and Getting Information From Arrays
Since they are like variables, lets try some examples and see how to use them. Here is a small program that creates an array and sums its contents.
#include
using namespace std;
int main()
{
// data is open tickets by employee
const int employees = 5; // employees
int tickets[employees]; // elements of array
int total = 0; // total tickets
int count; // loop counter
// get tickets outstanding
for (count =0; count < employees; count++)
{
cout << "Enter the current tickets by each employee " << (count + 1) << ": ";
cin >> tickets[count];
total += tickets[count];
}
cout << "The number of tickets we have is " << total;
return 0;
}
This is a typical program except for the array part. At the beginning of the main function I create the array and use a constant integer variable so it can’t be changed by the program later. The other variables are self-explanatory. I have one for the total amount of tickets and a loop counter. Both of these are necessary.
In the next section I have a [for] loop that takes input from the user and stops when I have hit the last employee. As the loop runs I am making a running total of the tickets we have. Then lastly I print to the screen our total.
Averaging the Values of an Array
We have done most of the work already to do this. So it will be just another couple steps. In our current program we already have a total to work with. So all we need to do is some division. After we have done the calculations, we will just display our results.
#include
using namespace std;
int main()
{
// data is open tickets by employee
const int employees = 5; // employees
int tickets[employees]; // elements of array
int total = 0; // total tickets
int count; // loop counter
double average; // average of our tickets per employee
// get tickets outstanding
for (count =0; count < employees; count++)
{
cout << "Enter the current tickets by each employee " << (count + 1) << ": ";
cin >> tickets[count];
total += tickets[count];
}
average = total / employees;
cout << "The number of tickets we have is " << total << endl;
cout << "The average number of tickets we have per employee is " << average;
return 0;
}
In line 12 I added a variable to hold our average value. I output its value on line 25 since I already had the other data I needed. That is really all there is to it. It was nice and simple to add that addition.
Reading Data From a File
This is the same topic I covered a long time ago in an earlier article. The difference is that I am putting the data into an array after reading the file. Here is the code, I will go over it.
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
// variables
const int number = 5;
int array[number];
int count;
ifstream inputfile;
// opening file to read from
inputfile.open("numberfile.txt");
// reading from file into the array
for (count = 0; count < number; count++)
{
inputfile >> array[count];
}
// closing our file
inputfile.close();
// printing the elements of the array one line at a time
for (count = 0; count < number; count++)
{
cout << array[count];
cout << endl;
}
return 0;
}
So in this program I start it out just like most others. I organize my program into its sections to keep things neat. I create my constant and initialize my array. I use a text file I have with a few property numbers to read from. It's a good example. I then start a [for] loop to read through the file and assign values to my elements in the array. After I am done with the file I close it so I don't get any weird errors with it being open. Then I use another [for] loop to print the values that have been stored in the array. All goes according to plan and we have the numbers printed to the screen. So this example file only had 5 numbers. You might ask why would you do this for 5 numbers ands the answer is that you would not. However, what if you had 500, 5000, or 50000 numbers to read and do some calculations. That is where the real power of C++ comes into play. It is powerful and fast. It will make short work of intensive calculations such as the example I just mentioned.
Finding The Highest Number In An Array
If you have to compare values from a lot of data this can be very useful. If I have 1000 values I can input them into an array and compare quickly to see what is the highest. Let me show you how this works.
#include
#include
using namespace std;
int main()
{
// variables
const int element = 100;
int array[element];
int count = 0;
int highest = 0;
ifstream inputfile;
// opening file to read from
inputfile.open("numberfile.txt");
// reading from file into the array
for (count = 0; count < element; count++)
{
inputfile >> array[count];
}
// closing our file
inputfile.close();
// printing array values
for (count = 0; count < element; count++)
{
cout << array[count] << endl;
}
cout << endl;
// finding highest value in the array
highest = array[0];
for (count = 1; count < element; count++)
{
if (array[count] > highest)
highest = array[count];
}
cout <<"The largest of these values is " << highest << endl;
return 0;
}
In this example, I had a 100 values in a text file. I wanted to read each of them into an array and then do a comparison to see which was the largest. After they were in the array, I went ahead and printed them onto the screen. I then added a section to analyze each of them. It was interesting to me to do it like this because I did not want to read over each line. This was only 100 values. What if I had 20000 to look at? I would have some headache after all that!
Conclusion
I am going to end here, but I have more to do with arrays in my next C++ article. They can be used with functions which will be very helpful. I want to also start sorting them so we can do more processing, yay! Hope someone enjoyed this article. I put a lot of work into it. Every day we are bombarded with data from a variety of sources. I find it handy to be able to process large amounts at once when it is presented to me. Thanks for reading for those that got to the end.