
How To Read Data From A File With C++
These are my notes on how to read data from a file with C++.
Intro
C++ is a really cool language. Despite its age it is still being updated and is one of the top languages around. For hardware programming it is still king and nothing will take its place any time soon. You can do much more than hardware programming in it though. In this article I combine C++ with a basic physics experiment. I show how to read data from a file with C++. I use this data to calculate gravity on Earth. Hopefully I can get close. C++ is so flexible as you can see. That is why I choose to use it still and do tutorials on it. This lesson is over how to read data from a file. This is still information in a first semester course but I have been busy lately. I plan to write articles well past the first semester so keep checking back. Now on to this lesson.
Read Data From A File With C++
This lesson is about reading data from a file. This is done by using text files. C++ can read data directly from a text file and then do any processing that you need from there. The data can be either numeric or string. To me, numeric data is much more useful but other people probably have uses for string data. I am a lover of Physics and Astronomy and enjoyed doing experiments.
One of these experiments was measuring gravity on Earth. There are multiple ways of doing this but one of the oldest was using a pendulum. You attach a weight to one end of a string and you can measure the oscillation motion that it makes. This is done many, many times and you record the data each time.
There are several ways to handle the data but the one I was first taught was to take an average. After you have done as many as you want you apply a simple formula and you will get the gravity that this planet applies to every mass here.
Where C++ Comes In
This data from our experiment is recorded into a text file on your laptop or workstation. When I did this experiment I had a laptop with me and just recorded all test results as they happened. It would also be convenient if you had a workstation in the lab with you so. Either way works though. The advantage to this method is that you can do the experiment for days, weeks, or even months if you are so inclined.
After all, experiments are an on going progress. You will be able to run this program at any time in your experiments to see what your data looks like. Another advantage is that you can then do other things with the data. Applying statistical methods to the data is trivial at this point.
Now the great thing about this is that whatever you want to do can just be coded into your C++ program and then displayed however you would like. So I am going to show you how to read this numerical data from a text file, process it for you gravity calculation, and then output it to your screen or another file.
Start Up Your Compiler
Whether you use a compiler from your command line or an actual IDE it is now time to start it up. Soon I am going to do an article on how to compile and make programs as well. At first I assumed it was too basic of an article but I have got several emails on how to set things up in Windows and in Linux distributions. After I get that done I will link to that article here.
Starting Your Program
Now we want to start our program. You should be a little bit familiar with C++ at this point but if not you can try my articles Input and Output Basics and Making Decisions which will help get beginners started using C++.
Reading Character Data
There are multiple ways to read data from a file. I am just going to just talk about the basic ways at this point though. You can read a single character at a time. This is useful when you want the person at the computer to make a decision and type a certain character like in a menu system.
This can also be used when you want to pause the program and have the computer operator type a character to continue the program. It would be done like this.

As you can see I read a character into the program. Depending on what choice was made, I printed a different message to the screen. This was just user input but I could have read from a file too. Now we will get on to files.
Working With Files
As I mentioned earlier, having data in a text file has several advantages. It is just the easiest way to deal with some types of data. To do this we have to now work with files in C++. The first step in modifying your programs for this is to use the #include <fstream> directive. That stands for file stream obviously. It goes at the top of your program with your other directives.
After that we need to create a file stream object. This is best explained by saying you are creating a command to work in this file stream environment only. So data comes from the file, into the object, into variables inside your program, and then processed however you want. These commands are:
- ofstream - This is the output file stream. It is used to create files and write data to files.
- ifstream - This command is the input file stream. It does the opposite. It will open files and read the data in them.
Open Your Data File
The next step is to open your file so the contents can be read and manipulated. To do that you use the input file stream command with its object name. Do it like this.
- ifstream file;
- file.open("experiment.data");
This will now open the file [experiment.data] and allow us to work with it. The file [experiment.data] is the file I put my experiment data into. It actually has lots of data in it but at first we will only be using a little of it.
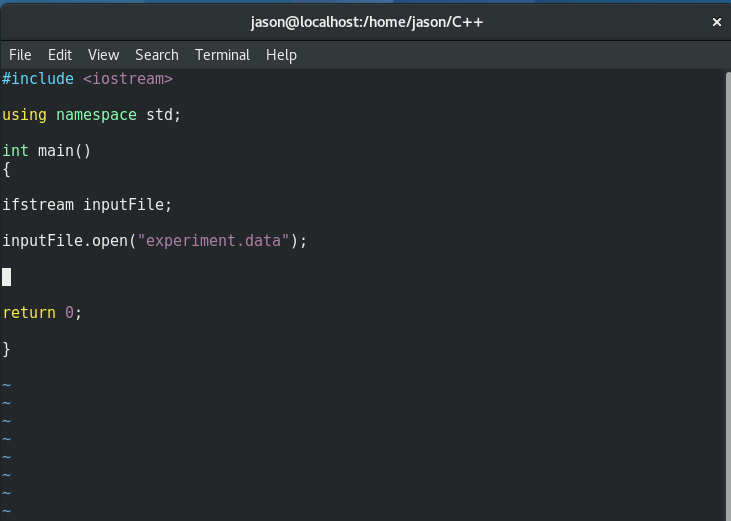
Now that it is open we can continue with our program. We will want to now read the data from the text file into memory so that we can work on it. If your text file is not in your current directory then just add the full path inside the quotes.
Reading Line Data
Now it is time to start working with this file. It contains numeric data. If it had only text or string data that would be fine too. You can read and store the information the same way. Of course the code will just be slightly different. This file is different because it has only the times listed on a separate line.
Now to read data from our file the next step is to pair the object [file] with the stream extraction operator [>>].
This command will read the first line of data in the file. After it reads the first line of the file, it needs to go somewhere. You just put it in a variable.
I made a variable and put it in my program like this.

Now after the stream extraction operator pulls the data from the file it is now stored in the variable [data1]. The data type [float] before it will allow us to represent decimals. It is up to us to decide what we will do with the information that is now in our variable. Obviously we many options.
You could just print it to screen, do more processing with it, or send it back out to another file that you specify. For this program, however, we will take more data and then process it.
Expanding Our Program
This program was designed to help me calculate gravity from an experiment that I did. I am sure many of you know of the famous pendulum experiment. It can be effectively used to calculate gravity with nice accuracy. If my data is good then I should be right at it. To expand this program I am going to add more variables.
Each variable will be assigned to a line of data. Then other variables I will have to create will help me calculate gravity from a known formula.
Variable Section Of Program
To do this experiment I had to build a pendulum apparatus from stuff around home. That was interesting let me tell you! I was taking apart kids toys and modifying some of my own things to make this work.
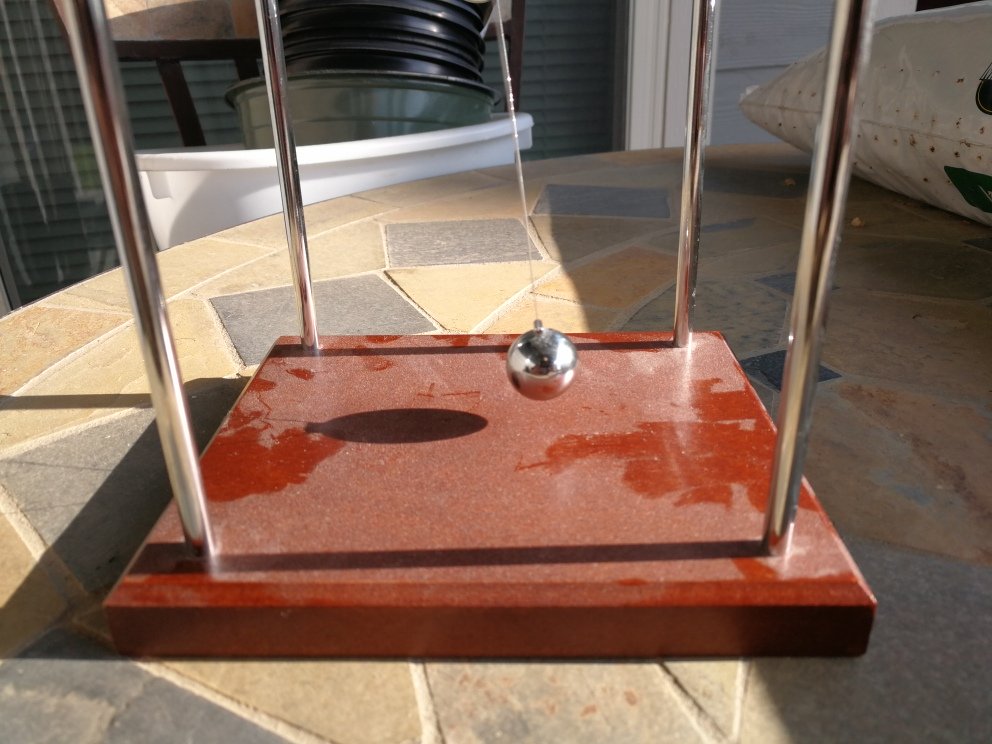
With this makeshift pendulum set up I could now time how long it took to do 30 oscillations. An oscillation is the complete back and forth motion once you pull it back and let it go. The time for all of the oscillations was the data that went into my text file.
I just used a timer on my desktop to time the oscillations. With those time in there it was now time to do some calculations. First, though, I had to set up a few more variables. Here is what I had:
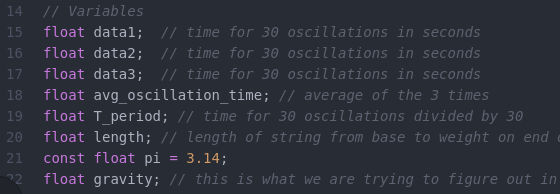
I tried to make my variables self-explanatory from my comments.
Reading My Data
While I did my experiment around, I think, a 100 times, we are not going to process that all at once. The main reason is that it is not practical without using loops in C++ and I have not gone over loops yet in this series.
I am now ready to read the rest of my data. I created a couple more [data] variables and was ready to go. Here is how you set up the data extraction section.

As I talked about before, line 25 is where I created my file stream object. That object is then used to open a file that had my data in it. Then I used the same object [file] to extract the data from the text file into my variables. It's nice and easy.
Calculations
To do some of these calculations it became apparent that I needed a math library that is available to C++. That was <cmath>. It goes into the header section at the very top of the program along with all of the other headers.
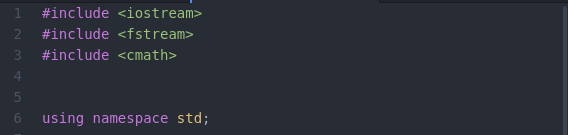
Now we can use a cool function called [pow] in order to do squares of numbers and variables easier.
Gravity is well known. The formula to calculate it in words is pi squared * length of string *4 /period squared. That sounds ugly I know. So other than that I just need to figure out my average oscillation time from my data and then the period. Easy enough though. It is all in my processing section which looks like this now:
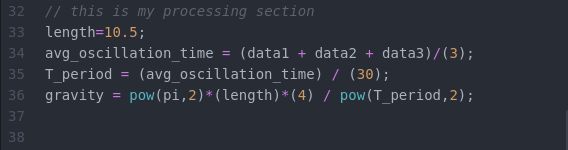
I want to point out the [pow] function here. It's form is [pow( number, exponent)]. This means that if number was 3 and the exponent value was 2 then you would end up with 9. That is how it works. Instead of having a number though you could use a variable like I did. You can see I used [pi] which I defined in the variable section above. It works the same way though.
That function will square the value of [pi]. I think my average oscillation time and period calculations are easy enough to understand so I won't go over them specifically.
Displaying My Results
This is the last section of most programs. You have done your research, your coding, and your calculations. Now it is time to see the results. There is nothing unusual in my section here. I did not even format the results because it was not needed. I am not running a report for a company here.
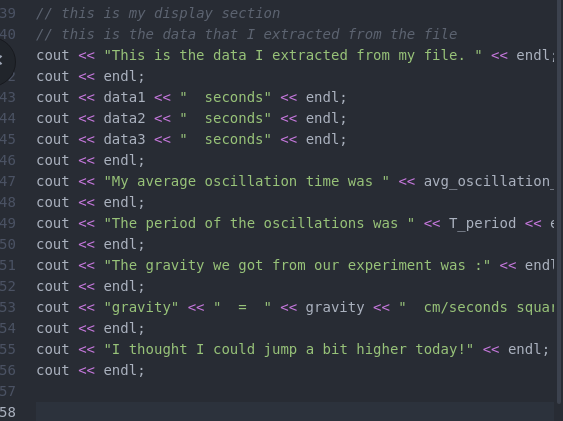
Most of my work here with all of these output statements was getting my results to looks nice. I like things nice and neat. Yes I even made a joke at the end. When you see the results you should understand my joke, I hope !
I will run this program now and show you the results I got.

For this I wanted to show specifically the data I extracted from my data file. I will also post a picture of the data file too or part of it at least.
Then as you can see I did my calculations. All elementary I know with basic algebra. Since I did them I might as well show what I got I thought.
Last, there is my gravity that I came up with. I left it in centimeters instead of meters but I am sure that won't bother anyone. Can you see the meaning of my joke yet? Yes my data was not very good and my gravity was lower than it should be. It should have been around 980.00 centimeters/second squared. The probable culprit was my reaction time in doing the experiment.
My Data File
Here is part of the data file that I keep adding to. I keep doing this experiment and can watch as the time times fluctuate slightly. I just keep adding to my file and then just process the whole thing at once. Here are the first 10 lines of my data so i can keep the picture size down.
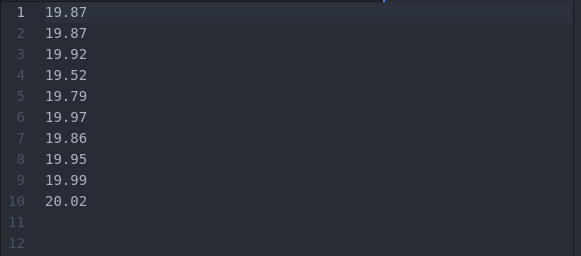
If you try to emulate this experiment you should get similar results. measure the length of your string using centimeters or millimeters. Put that in your formula. Release your weight at the end of the string from the same position every time. Use a digital time on desktop or something handheld if you want. Then have fun and see what happens!
The Whole C++ Program
I almost forgot to to screen shot all the code in my program. It is simple but it works. Later on I want to show you how to further improve it. There are many ways but learning programming is done a step at a time and then applying to a project. I firmly believe that.
https://gist.github.com/anonymous/f1e69f55f94fbb3d3997c3c1f9b3f63b
This is all of my code. It does the job of what I asked in a way without loops using standard C++. Hope it makes sense to all and is commented sufficiently for everyone who needs it.
Conclusion
I hope you all enjoyed this little experiment and then letting C++ do some of the work on it. Learning how to read data from a file with C++ is a useful technique to learn. I admit it was very fun for me also. C++ has a lot of power and can be adapted for many things. Sometimes it takes a bit of creativity but really not very much. C++ has a lot more math you can do with it which will make other tasks easier.
Soon I will write about looping in C++ and may come back to this to show how all of the data could be processed in the blink of an eye. I encourage you all to take a second look at C++ if you are not familiar with it. Today only scratched the surface of its capabilities.