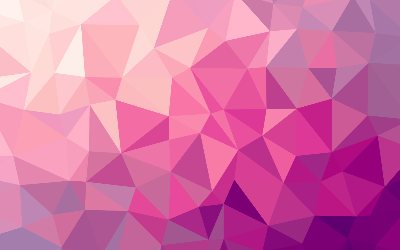
Using Strings in Python
These are my notes on using strings in python.
Manipulating Strings
Strings can be manipulated in a program using the various operators that are
available in python.
+ concatenate - join strings together
* repeat - multiply the string
[] slice - select a character at a specified index position
[:] range slice - select characters in a specified index range
in membership exclusive - return True if character exists in the string
not in membership exclusive - return True if character doesn't exist in string
r/R raw string - suppress meaning of escape characters
''' ''' docstring - describe a module, function, class, or method
The [] slice operator and [:] range slice operator recognize that a string is
simply a list containing an individual character within each element, which can
be referenced by their index number.
Similarly, the in and not in membership operators iterate through each element
seeking to match the specified character.
The raw string operator, r, must be placed immediately before the opening quote
mark to suppress escape characters in the string, and is useful when the string
contains the backslash character.
A docstring is a descriptive string literal that occurs as the first statement
in a module, a function, a class, or a method definition. This should be
enclosed within triple single quote marks. Uniquely, the docstring becomes the
_doc_ special attribute of that object, so can be referenced using its name and
dot-suffixing. All modules should normally have docstrings, and all functions
and classes exported by a module should also have docstrings.
The membership operators performa case-sensitive match, so A in abc will fail.
The range slice returns the string up to, but not including, the final specified
index position.def display(s):
'''Display an argument value.'''
print(s)
display(display.__doc__)
display(r'home\jason')
display('\nHello' + ' Andromeda')
display('C++ is cool, too!\n'[0:11])
display('C' in 'C++')
display('r' in 'Andromeda')
The doc keyword is preceded by 2 underscores and followed by 2 underscores.
Remember that strings must be enclosed within either single quote marks or
double quote marks. With range slice, if the start index number is omitted, zero
is assumed, and if the end index number is omitted, the string length is
assumed.
Formatting Strings
The python built-in dir() function can be useful to examine the names of
functions and variables defined in a module by specifying the module name within
its parentheses. Interactive mode can easily be used for this purpose by
importing the module name then calling the dir() function.
Any defined names that begin and end with a double underscore are python
objects, whereas the others are programmer-defined. The __builtins__ module can
also be examined using the dir() function, to examine the names of functions and
variables defined by default, such as the print() function and a str object.
The str object defines several useful methods for string formatting, including
an actual format() method that performs replacements. A string to be formatted
by the format() method can contain both text and replacement fields marking
places where text is to be inserted from an ordered comma-separated list of
values. Each replacement field is denoted by {} braces, which may, optionally,
contain the index number position of the replacement in the list.
Strings may also be formatted using the C-style %s substitution operator to mark
places in a string where text is to be inserted from a comma-separated ordered
list of values.book='{} and {}'.format('Chemistry', 'Electronics')
print('\nReplaced:', book)
book='{1} and {0}'.format('Chemistry', 'Electronics')
print('Replaced:', book)
book='%s and %s' %('Calculus', 'Proofs')
print('\nSubstituted:', book)
You cannot leave spaces around the index number in the replacement field. Other
data types can be substituted using %d for a decimal integer, and %f for a
floating-point number.