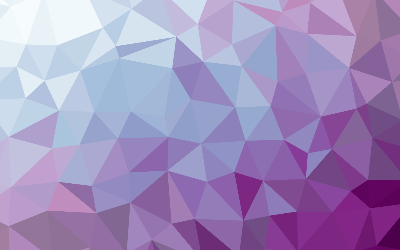
Branching in Python
These are my notes on branching in Python.
Branching with If
The Python if keyword performs the basic conditional test that evaluates a given expression for a boolean value of True or False. This allows a program to proceed in different directions according to the result of a test and it is known as conditional branching.
The tested expression must be followed by a :, then statements to be executed when the test succeeds should follow below on separate lines, and each line must be indented from the if test line. The size of the indentation is not important, but it must be the same for each line.
If expression
Do this
An if test can offer alternative statements to execute when the test fails by appending an else keyword after the statements to be executed when the test succeeds.
If expression
Do this
Else:
Alternative expression
An if test block can also be followed by an alternative test using the elif keyword (else if) that offers statements to be executed when the alternative test succeeds.
If expression
Do this
Elif another expression
Do this instead
Else:
Do this when the above are false
Conditional branching can also be performed using a match case block. A value to be evaluated is specified after the match keyword and this is compared to a pattern specified to one or more case statements. Only when the specified value matches a pattern will the statements inside that case block be executed, otherwise the program will simply proceed. A match case block has this syntax:
Match value:
Case 1:
Do this
Case 2:
Do this
Case 3:
Do this
Indentation of code is very important in Python as it identifies code blocks to the interpreter. The match case block in Python is similar to the switch case block found in other languages.
num = int(input(' Please enter a number: '))
if num > 5:
print(' Number is greater than 5\n ')
elif num < 5:
print(' Number is less than 5\n ')
else:
print(' Number is 5\n ')
cmd = input(' Enter STOP or GO: ').upper()
match cmd:
case 'GO':
print(' Started ')
case 'STOP':
print(' Stopped ')
The user input is read as a string value by default, so must be cast as an int data type with int() for arithmetical comparison. The match comparison is case-sensitive so the user input is forced to uppercase by the upper() string method before comparison.
Looping
A loop is a piece of code in a program that automatically repeats. One complete execution of all statements within a loop is called an iteration. The length of the loop is controlled by a conditional test made within the loop. While the tested expression is found to be true, the loop will continue. When the tested expression is found to be false the loop ends.
In Python programming, the while keyword created a loop. It is followed by the test expression then a : character. Statements to be executed when the test succeeds should follow below on separate lines, and each line must be indented the same space from the while test line. This statement block must include a statement that will at some point change the result of the expression evaluation, otherwise an infinite loop is created.
Indentation of code blocks must also be observed in Python’s interactive mode. Loops can be nested, one within another, to allow complete execution of all iterations of an inner nested loop on each iteration of the outer loop. A counter variable can be initialized with a starting value immediately before each loop definition, included in the test expression, and incremented on each iteration until the test fails, then the loop ends.
Unlike other Python keywords, the keywords True and False begin with uppercase letters. Hit return to move to the next line and see the interpreter automatically indent the new line as it expects further statements. Hit return again to execute the entered code.
i = 1
while i <4:
print( '\nOuter loop iteration: ', i)
i+=1
j = 1
while j <4:
print( '\nInner loop iteration: ',j)
j+=1
The output printed from the inner loop is indented from that of the outer loop by the \t tab character. The += assignment statement i +=1 is simply a shorthand way to say i=i+1.
Looping Over Items
In Python programming, the for keyword loops over all items in any list specified to the in keyword. This statement must end with a : colon character, and statements to be executed on each iteration of the loop must be indented.
For each item inlist
Statements to be executed on each iteration
Statements to be executed on each iteration
As a string is simply a list of characters, the for in statement can loop over each character. Similarly, a for in statement can loop over each element in a list, each item in a tuple, each member of a set, or each key in a dictionary.
A for in loop iterates over the items of any list or string in the order that they appear in the sequence, but you cannot directly specify the number of iterations to make, a halting condition, or the size of iteration step. You can, however, use the Python range() function to iterate over a sequence of numbers by specifying a numeric end value within its parameters. This will generate a sequence that starts at zero and continues up to, but not including, the specified end value. For example, range(5) generates 0,1,2,3,4.
Optionally, you can specify both a start and end value within the parentheses of the range() function, separated by a comma. For example, range(1,5) generates 1,2,3,4. Also, you can specify a start value, end value, and a step value to the range() function as a comma-separated list within its parentheses. For example, range(1,14,4) generates 1,5,9,13.
You can specify the list’s name within the parentheses of Python’s enumerate() function to display each element’s index number and its associated value.
When looping through multiple lists simultaneously, the element values of the same index number in each list can be displayed together by specifying the list names as a comma-separated list within the parentheses of Python’s zip() function. To ensure lists are of equal length, this can include a final strict=true argument.
When looping through a dictionary you can display each key and its associated value using the dictionary items() method and specify two comma-separated variable names to the for keyword, one for the key name and the other for its value.
The range() function can generate a sequence that decreases, counting down, as well as those that count upward. The for loop in Python is unlike that in other languages, as it does not allow step size and end value to be specified.
chars=[ 'A', 'B', 'C' ]
fruit=( 'Apple', 'Banana', 'Cherry' )
dict={ 'name': 'Mike', 'ref': 'Python', 'sys': 'Win' }
print( '\nElements:\t', end='')
for item in chars:
print( item, end='')
print( '\nEnumerated:\t', end='')
for item in enumerate( chars ):
print( item, end='' )
print( '\nZipped:\t', end='' )
for item in zip( chars, fruit, strict=True ):
print( item, end='' )
print( '\nPaired:' )
for key, value in dict.items():
print( key, '=', value )
In Python programming, anything that contains multiple items that can be looped over is called iterable.
Breaking Out of Loops
The Python break keyword can be used to prematurely terminate a loop when a specified condition is met. The break statement is situated inside the loop statement block and is preceded by a test expression. When the test returns True, the loop ends immediately and the program proceeds on to the next task. For example, in a nested inner loop it proceeds to the next iteration of the outer loop.
for i in range(1,4):
for j in range(1,4):
print( 'Running i=' , i , 'j=' , j )
Now, once you see how that runs, we will add a break statement to see how it will affect the previous code.
for i in range(1,4):
for j in range(1,4):
print( 'Running i=' , i , 'j=' , j )
if i == 2 and j == 1:
print( 'Breaks inner loop at i=2 j=1' )
break
The Python continue keyword can be used to skip a single iteration of a loop when a specified condition is met. The continue statement is situated inside the loop statement block and is preceded by a test expression. When the test returns true, that one iteration ends and the program proceeds to the next iteration.
for i in range(1,4):
for j in range(1,4):
print( 'Running i=' , i , 'j=' , j )
if i==1 and j==1:
print( 'Continue inner loop at i=1 and j=1' )
continue
if i == 2 and j == 1:
print( 'Breaks inner loop at i=2 j=1' )
break
Here, the break statement halts all three iterations of the inner loop when the outer loop tries to run it the second time. The continue statement just skips the first iteration of the inner loop when the outer loop tries to run it for the first time.