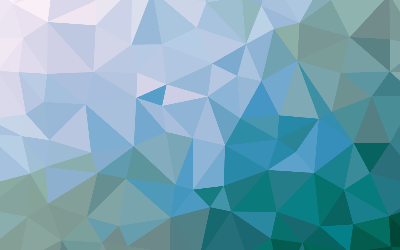
Python Operations
This article is about doing Python operations.
Let us get familiar with python operations and how they are used.
If you have not already, make a new folder at the base of your drive and call it python.
We will store our scripts here in case we need them to expand on a subject.
As in other programming languages, use parentheses to direct the order of operations.
Values in our expressions are called operands.
All the different python operations have an order in which they are performed.
Arithmetic
Define some variables:
a=5
b=10
print( a, '+', b, '=', a + b )
5 + 10 = 15
print( a, '-', b, '=', a - b )
5 - 10 = -5
print( a, '*', b, '=', a * b )
5 * 10 = 50
print( b, '/', a, '=', b / a )
10 / 5 = 2.0
print( a, '%', b, '=', a / b )
5 % 10 = 0.5
print( a, '^2 =', a*a, sep = '')
5^2 = 25
The sep character sequence specifies space in the output. We will use it more later.
Assigning Values
There are many ways to assign values in python. There are several ways to assign values because these are shortcuts and save time once you learn them.
= | a=b | a=b |
+= | a+=b | a=(a+b) |
-= | a-=b | a=(a-b) |
*= | a*=b | a=(a*b) |
/= | a/=b | a=(a/b) |
%= | a%=b | a=(a%b) |
//= | a//b | a=(a//b) |
**= | a**b | a=(a**b) |
These operations are efficient to use. It will save you time by learning these shortcuts slowly.
Also, the ‘=’ operator assigns a value to a variable. It does not test equality between two different variables or values.
Let us practice these shortcuts with some simple examples.
a=10
b=20
print(‘Initializing Variables:\t\t’, ‘a =’ a, ‘\tb =’, b)
a += b
print(‘Addition:\t\t’, ‘a =’, a, ‘(10+20)’)
a -= b
print(‘Subtraction:\t’, ‘a =’, a, ‘(20-10)’)
a *= b
print(‘Multiplication:\t’, ‘a =’, ‘(10*20)’)
a /= b
print(‘’Division:\t’, ‘a =’, a, ‘(20 / 10)’)
a %= b
print(‘Modulo:\t’, ‘a =’, a, ‘(20 % 10)’)
Comparing Values
As in math and other languages, there are several operators for comparing values in python. The most commonly used are:
== | Equality |
!= | Inequality |
> | Greater Than |
< | Less Than |
>= | Greater Than or Equal To |
<= | Less Than or Equal To |
This is logic math. For example, the ‘==’ will compare two operands and return true if both are equal in value. The ‘!=’ operator returns true if two operands are not equal. The ‘>’ operator returns true if the first operator is greater than the second. The ‘<’ operator returns true if the first operator is less than the second.
The ‘>=’ operator returns true if the operand is greater than or equal to the second. The ‘<=’ returns true if the operand is less than or equal to the second.
nothing=0
zero=0
one=1
upper= 'A'
lower= 'a'
print('Equality :\t', nothing, '==', zero, nothing == zero)
print('Equality :\t', upper, '==', lower, upper == lower)
print('Inequality :\t', nothing, '!=', one, nothing != one)
print('Greater :\t', nothing, '>', one, nothing > one)
print('Lesser :\t', nothing, '<', one, nothing < one)
print('Greater or Equal To :\t', nothing, '>=', zero, nothing >= zero)
print('Less Than or Equal To :\t', one, '<=', zero, one <= zero)
Logic
Logic operators are used a lot in python. They include:
and | Logical And |
or | Logical Or |
not | Logical Not |
Logical And will look at two operators and return true if both operands are true.
Logical Or will look at two operators and return true if one of the operands is true.
Logical Not is used with another operand and returns the inverse value of it.
This is called boolean logic.
t=True
f=False
print('And Logic:')
print('t and t =', t and t)
print('t and f =', t and f)
print('f and f =', f and f)
print('\nOr Logic:')
print('t or t =', t or t)
print('t or f =', t or f)
print('f or f =', f or f)
print('\nNot Logic:')
print('t =', t, '\tnot t =', not t)
print('f =', f, '\tnot f =', not f)
Conditions
Every programming language I have heard of has a way to test conditions to see if they are true or false. Python is no different. You have an expression and if it is true you do a certain action. However, if it is false , you do a different action. It is called the conditional expression. You can use several different operators with the conditional expression.
An expression will return a value of some kind. In Python, this is the test expression and up to two actions afterwards.
a=1
b=2
print('\nVariable a is :', 'One' if(a==1) else 'Not One')
print('Variable a is : :', 'Even' if(a%2==0) else 'Odd')
print('\nVariable b is :', 'One' if (b==1) else 'Not One')
print('Variable b is :', 'Even' if (b%2==0) else 'Odd')
max = a if (a>b) else b
print('\nGreater Value Is :', max)
Precedence
Operator precedence determines the order in which the interpreter evaluates expressions. You can dictate precedence with certain operators.
a=2
b=4
c=8
print('\nDefault Order :\t', a, '*', c,'+', b, '=', a*c+b)
print('Forced Order :\t', a, '* (',c,'+',b,') =', a*(c+b))
print('\nDefault Order :\t', c, '//', b, '-', a, '=', c//b-a)
print('Forced Order :\t', c,'// (',b,'-', a,')=', c//(b-a))
print('\nDefault Order :\t', c, '%', a, '+', b, '=', c%a+b)
print('Forced Order :\t', c, '% (',a,'+',b,')=', c%(a+b))
print('\nDefault Order :\t', c, '**', a, '+', b, '=', c**a+b)
print('Forced Order :\t', c, '** (',a, '+', b,')=', c**(a+b))
Casting Data Types
The most popular data types are string, integer, and float. Data type recognition is especially important when assigning numeric data to variables from user input as it is stored by default as a string data type. The data type of stored values can be easily converted into a different data type using built-in functions. The built-in data type conversion functions return a new object representing the converted value.
- int(x) - converts x to an integer whole number
- float(x) - converts x to a floating-point number
- str(x) - converts x to a string representation
- chr(x) - converts x to a character
- unichr(x) - converts x to a unicode character
- ord(x) - converts x to its integer value
- hex(x) - converts x to its hexadecimal value
- oct(x) - converts integer x to an octal string
The Python built-in type() function can be used to determine which data type class the value contained in a variable.
a=input('Enter A Number:')
b=input('Now Enter Another Number:')
sum=a+b
print('\nData type sum:', sum, type(sum))
sum=int(a)+int(b)
print('Data type sum:', sum, type(sum))
sum=float(sum)
print('Data type sum:', sum, type(sum))
sum=chr(int(sum))
print('Data type sum:', sum, type(sum))
Manipulating Bits
In computer terms, each byte comprises 8 bits that can each contain a 1 or a 0 to store a binary number, representing decimal values from 0 to 255. It is possible to manipulate individual parts of a byte using the Python bitwise operators listed below:
- | Or
- & And
- ~ Not
- ^ Xor
- << Shift left
- >> Shift right
Unless programming for a device with limited resources, there is seldom a need to utilize bitwise operators, but they can be useful.
a=10
b=5
print('a=', a, '\tb=', b)
# 1010 ^ 0101 = 1111 (decimal 15)
a=a^b
# 1111 ^ 0101 = 1010 (decimal10)
b=a^b
# 1111 ^ 1010 = 0101 (decimal 5)
a=a^b
print('a=', a, '\tb=', b)
Arithmetic operators can form expressions with two operands for addition, subtraction, multiplication, division, floor division, modulo, and exponent.
The assignment operator (=) can be combined with an arithmetic operator to perform an arithmetic calculation then assign its result.
Comparison operators can form expressions comparing two operands for equality, inequality, greater, lesser, greater or equal, and lesser or equal values.
Logical (and) and (or) operators form expressions evaluating two operands to return a boolean value of true or false.
The logical not operator returns the inverse boolean value of a single operand.
A conditional if-else expression evaluates a given expression for a boolean True or False value, then returns one of two operands depending on the result.
Expressions containing multiple operators will execute their operations in accordance with the default precedence rules unless explicitly determined by the addition of parentheses.
The data type of a variable value can be converted to a different data type by the built-in Python functions int(), float(), and str() to return a new converted object.
Python’s built-in type() function determines to which data type class a specified variable belongs.
Bitwise operators OR, And, Not, and Xor each return a value after comparison of the values within two bits whereas the Shift left and Shift right operators move the bit values a specified number of bits in their direction.