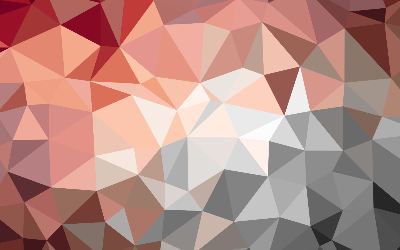
Scope of Functions in Python
These are my notes on the scope of functions in Python.
Most Python programs contain a number of functions that can be called when they are needed. These are custom functions. A custom function is created using the “def” keyword. It stands for definition. You follow it with a function name of your choice and parentheses. You can choose any name for your function except Python keywords. This line must end with a colon character. The statements to be executed whenever the function gets called must appear on lines below and be indented.
Def base_steals();
Statement1
Statement2
Function statements must be indented from the definition line by the same amount so the Python interpreter can recognize the block.
Once the function statements have been executed, program flow resumes at the point directly following the function call. This modularity is very useful in Python programming to isolate set routines so they can be called upon repeatedly.
To create custom functions it is necessary to understand the accessibility scope of variables in a program. Variables created outside functions can be referenced by statements inside functions, so they have global scope. Variables created inside functions cannot be referenced from outside the function in which they have been created, so these have local scope.
The limited accessibility of local variables means that variables of the same name can appear in different functions without conflict. If you want to coerce a local variable to make it accessible elsewhere, it must first be declared with the Python global keyword followed by its name only.
It may subsequently be assigned a value that can be referenced from anywhere in the program. Where a global variable and a local variable have the same name, the function will use the local version.
Avoid using global variables in order to prevent accidental conflict, use only local variables where possible.
global_var=1
def my_vars():
print('Global Variable:', global_var)
local_var=2
print('Local Variable:', local_var)
global inner_var
inner_var=3
my_vars()
print('Coerced Global:', inner_var)
Arguments To Your Functions
When defining a custom function in Python programming, you can specify an argument name between the function’s parentheses. A value can then be passed to that argument by specifying the value in the parentheses of the call to the function. The function can now use that passed in value during its execution by referencing it via the argument name. For example, defining a function to accept an argument to print out, like this…
Def echo(user):
print(‘User:’, user)
A call to this function must specify a value to be passed to the argument within its parentheses so it can be printed out:
echo(‘YourName’)
Multiple arguments can be specified in the function definition by including a comma-separated list of argument names within the function parentheses:
Def echo(user, lang, sys):
print(‘User:’, user, ‘Language:’, lang, ‘Platform:’, sys)
When calling a function whose definition specifies arguments, the call must include the same number of data values as arguments. For example, to call this example with multiple arguments:
echo(‘YourName’, ‘Python’, ‘Windows’)
The passed values must appear in the same order as the arguments list unless the caller also specifies the argument names, like this:
echo(lang=’Python’, user=’YourName’, sys=’Windows’)
Optionally, a default value may be specified in the argument list when defining a function. This will be overridden when the caller specifies a value for that argument, but will be used by the function when no value gets passed by the caller:
Def echo(user, lang, sys=’Linux’):
print((‘User:’, user, ‘Language:’, lang, ‘Platform:’, sys)
This means you may call the function passing fewer values than the number of arguments specified in the function definition, to use the default argument value, or pass the same number of values as specified arguments to override the default value.
Argument naming follows the same conventions as variables and functions. Name arguments the same as variables passed to them to make the data movement obvious.
def echo(user, lang, sys):
print('User:', user, 'Language:', lang, 'Platform:', sys)
echo('YourName', 'Python', 'Windows')
echo(lang='Python', sys='Mac OS', user='Anne')
def mirror(user='Carole', lang='Python'):
print('\nUser:', user, 'Language:', lang)
mirror()
mirror(lang='Java')
mirror(user='Tony')
mirror('Susan', 'C++')
Returning Values
Like Python’s built in str() function, which returns a string representation of the value specified as its argument by the caller, custom functions can also return a value to their caller by using the Python “return” keyword to specify a value to be returned. For example, to return to the caller the total of adding two specified argument values, like this:
Def sum(a,b):
Return a+b
The returned result may be assigned to a variable by the caller for subsequent use by the program, like this:
total=sum(8,4)
print(‘Eight plus four is:’, total)
Or the returned result may be used directly in line like this:
print(‘Eight plus four is:’, sum(8,4))
Typically, a return statement will appear at the very end of a function block to return the final result of executing all statements contained in that function.
A return statement may appear earlier in the function block to halt execution of all subsequent statements in that block. This immediately resumes execution of the program at the caller. The return statement may specify a value to be returned to the caller or the value may be omitted. Where no value is specified, a default value of “none” is assumed. Typically, this is used to halt execution of the function statements after a conditional test is found to be “false”. For example, where a passed argument value is below a specified number:
Def sum(a,b):
If a<b:
Return
Return a+b
In this case, the function will return the default value “none” when the first passed argument value is below five and the final statement will not be executed. Where the function is to perform arithmetic, user input can be validated for integer values with the built-in “isdigit()” function. You can specify a default value for an argument in the function definition.
num=input('Enter an integer:')
def square(num):
if not num.isdigit():
return 'invalid entry'
num=int(num)
return num*num
print(num, 'Squared is:', square(num))
Remember that user input is read as a “str” data type so ust be cast into an “int” or a “float” data type for arithmetic.