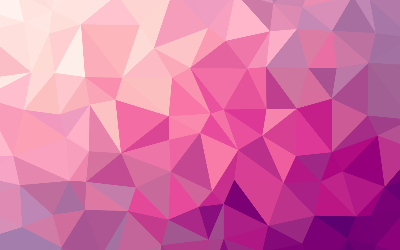
Using Modules in Python
This is my guide on using modules in python.
Storing Functions
Python function definitions can be stored in one or more separate files for
easier maintenance and to allow them to be used in several programs without
copying the definitions into each one. Each file storing function definitions is
called a module and the module name is the file name without the .py extension.
Functions stored in the module are made available to a program using the python
import keyword followed by the module name. Although not essential, it is
customary to put any import statements at the beginning of the program.
Imported functions can be called using their name dot-suffixed after the module
name. Where functions stored in a module include arguments, it is often useful
to assign a default value to the argument in the definition. This makes the
function more versatile, as it becomes optional for the call to specify an
argument value.
You can create an alias when importing a module using "import as" keywords. For
example, import pokemon as darkrai allows you to use darkrai as the function
prefix in function calls.
Function Names
Internally, each python module and program has its own symbol table that is used
by all functions defined in that context only. This avoids possible conflicts
with functions of the same name in another module if both modules were imported
into one program. Where you import individual function names, the module name
does not get imported, so it cannot be used as a prefix.
When you import a module with an import statement, that module's symbol table
does not get added to the program's table, only the module's name gets added.
That is why you need to call the module's functions using their module name
prefix.
Generally, it is prefarable to avoid conflicts by importing the module name and
calling its functions with the module name prefix, but you can import individual
function names instead with a from import statement. the module name is
specified after the from keyword, and functions to import are specified as a
comma-separated list after the import keyword. Alternatively, the * wildcard
character can be specified after import to import all function names into the
program's own symbol table. This means the functions can be called without a
module name prefix.
For larger programs, you can import modules into other modules to build a module
hierarchy.
System Queries
Python includes "sys" and "keyword" modules that are useful for querrying the
python system itself. The keyword module contains a list of all python leywords
in its "kwlist" attribute, and provides an "iskeyword()" mothod if you want to
test a word.
You can explore the many features of the "sys" module, and indeed any feature of
python, using the interactive mode help system. Just type "help()" at the ">>>"
prompt to start the help system, then type "sys" at the "help>" prompt that
appears.
Perhaps most usefully, the "sys" module has attributes that contain the python
version number, interpreter location on your system, and a list of all
directories where the interpreter seeks module files, so if you save module
files in any of these directories you can be sure the interpreter will find
them.
import sys, keyword
print("Python Version: ", sys.version)
print("Python interpreter location:", sys.executable)
print("Python module Search path:")
for dir in sys.path:
print(dir)
print("Python Keywords:")
for word in keyword.kwlist:
print(word)
The first item on the python search path is your current directory, so any file
within there, or within any subdirectories you make there, will be found by the
python interpreter.
Mathematical Operations
Python includes a math module that provides lots of methods you can use to
perform mathematical procedures once imported.
The "math.ceil()" and "math.floor()" methods enable a program to perform
rounding of a floating point value specified between their parentheses to the
closest integer. "Math.ceil()" rounds up and "math.floor()" rounds down but the
value returned, although an integer, is a float data type rather than an int data
type.
The "math.pow()" method requires two arguments to raise a specified value by a
specified power. The "math.sqrt()" method simply requires a single argument and
returns a square root of that specified value. Both method results are returned
as a numeric value of the float data type.
Typical trigonometry can be performed using methods from the math module too,
such as "math.sin()", "math.cosin()", and "math.tan()". Python also includes a
random module that can be used to produce pseudo random numbers once imported
into a program.
The random.random() method produces a single floating point number between zero
and 1.0. The random.sample() method produces a list of elements selected at
random from a sequence. This method requires two arguments to specify the
sequence to select from, and the length of the list to be produced. As the
range() function returns a sequence of numbers, this can be used to specify a
sequence as the first argument to the random.sample() method, so it will
randomly select numbers from that sequence to produce a list in which no numbers
repeat.
Integers can be cast from the int data type to the float data type using the
float() function and to the string data type using the str() function.
import math, random
print("Rounding up 6.6:", math.ceil(6.6))
print("Rounding down 3.3:", math.floor(3.3))
num=9
print(num, "Squared:", math.pow(num,2))
print(num, "Square Root:", math.sqrt(num))
numbers=random.sample(range(1,69),6)
print("The random numbers selected are:", numbers)
All the math methods here return floating point numbers of the float data type.
The list produced by random.sample() does not actually replace elements of the
sequence but merely copies a sample, as its name says.
Calculating Decimals
Python programs that attempt floating-point arithmetic can produce unexpected
and inaccurate results because the floating-point numbers cannot accurately
represent all decimal numbers.
book=14.99
rate=1.35
tax=book*rate
total=book+tax
print("item:\t", "%.2f" % book)
print("Tax:\t", "%.2f" % tax)
print("Total:\t", "%.2f" % total)
Here, the variable values are formatted using a string substitution technique to
show two decimal places.
To help understand this problem, edit all three print statements to display the
variable values expanded to 20 decimal places, then run the program again.
book=14.99
rate=1.35
tax=book*rate
total=book+tax
print("item:\t", "%.20f" % book)
print("Tax:\t", "%.20f" % tax)
print("Total:\t", "%.20f" % total)
It is now clear that the tax value is represented numerically slightly below
0.735, so gets rounded down to 0.73. Conversely, the total value is represented
numerically slightly above 1.435, so gets rounded up to 1.44, creating the
apparent addition error.
Erros in floating-point arithmetic can be avoided by using python's decimal
module. This provides a decimal() object with which floating-point numbers can
be more accurately represented.
Add a statement at the beginning of the program to import the decimal module to
make all features available from decimal import *. Then, edit the first two
variable assignments to objects:
book=Decimal(14.99)
rate=Decimal(1.35)
Now run the program again to see the difference.
from decimal import *
book=Decimal(14.99)
rate=Decimal(1.35)
tax=book*rate
total=book+tax
print("item:\t", "%.2f" % book)
print("Tax:\t", "%.2f" % tax)
print("Total:\t", "%.2f" % total)
Always use the decimal() object to calculate monetary values or anywhere that
accuracy is essential.