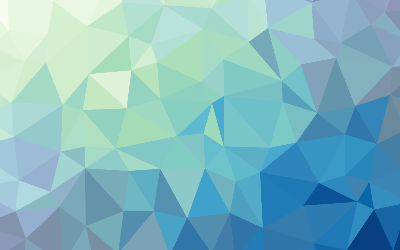
Launching a Window in Python
These are my notes on launching a window in python.
The standard python module that you can use to create graphical applications is
called "tkinter", a toolkit to interface with the system gui.
The tkinter module can be imported into a program like any other module to
provide attributes and methods for windowed apps. Every tkinter program must
begin by calling the tk() constructor to create a window object. The window's
size can be specified as a "widthxheight" string argument to the window object's
geometry() method. Similarly, the window's title can be specified as a title
string argument to the window object's title() method. If not specified, default
size and title values will be used.
Every tkinter program must also call the window object's mainloop() method to
capture events, such as when the user closes the window to quit the program.
This loop should appear at the end of the program as it also handles window
updates that may be implemented during execution.
With tkinter, all the graphical controls that can be included in the application
window, such as buttons or checkboxes, are referred to as widgets. Perhaps the
simplest widget is a non-interactive label object that merely displays text or an
image in the app interface. A label object can be created by specifying the
window object's name and text='string' as arguments to a Label() constructor.
Once created, each widget, such as a label, must then be added to the window
using one of these geometry manager methods.
pack()-places the widget against a specified side of the window using Top,
Bottom, left, or Right constant values specified to its side=argument.
place()-places the widget at XY coordinates in the window using numerical values
specified to its x= and y= arguments.
grid()-places the widget in a cell within the window using numerical values
specified to its row= and column= arguments.
Optionally, the pack() method may include a fill argument to expand the widget
in available space. For example, with fill='x'. Alternatively, the pacl() method
may include padx and pady arguments to expand the widget along an axis by a
specified amount.
There can be only one call to the TK() constructor and it must be at the start
of the program code.from tkinter import *
window=Tk()
window.title('Window Label')
label=Label(window, text='This is an introduction to interfaces in Python')
label.pack(padx=200, pady=50)
window.mainloop()
Widgets will not appear in the window when running the program unless they have
been added with a program manager.
Responding to Buttons
A button widget provides a graphical button in an application window that may
contain either text or an image to convey the button's purpose. A button object
is created by specifying the window name and options as arguments to a button()
constructor. Each option is specified as an option=value pair. The command
option must always specify the name of a function or method to call when the
user clicks that button. The most popular options are listed below, with a brief
description.
activebackground background color when the cursor is over
activeforeground foreground color when the cursor is over
bd border width in pixels(default is 2)
bg background color
font font for button label
height button height in text lines, or pixels for images
highlightcolor border color when in focus
image image to be displayed instead of text
justify multiple text lines as Left, center, or Right
padx horizontal padding
pady vertical padding
relief border style of Sunken, Ridge, Raised or Groove
state enabled status of normal or Disabled
underline index number in text of character to underline
width button width in letters, or pixels for images
wraplength length at which to wrap text
The values assigned to other options determine the widget's appearance. These
can be altered by specifying a new option=value pair as an argument to the
widget's configure() method. Additionally, a current option value can be
retrieved by specifying its name as a string argument to the widget's cget()
method.
You can also call a button's invoke() method to call the function nominated to
its command option. from tkinter import *
window=Tk()
window.title('Click this Button')
btn_end=Button(window, text='Close', command=exit)
def tog():
if window.cget('bg')=='yellow':
window.configure(bg='gray')
else:
window.configure(bg='yellow')
btn_tog=Button(window,text='Switch', command=tog)
btn_tog.pack(padx=150,pady=20)
btn_end.pack(padx=150,pady=20)
window.mainloop()
Only the function name is specified to the command option. Do not add trailing
parentheses in the assignment. The 'gray' color is the original default color of
the window.
Displaying Messages
A program can display messages to the user by calling methods provided in the
"tkinter.messagebox" module. This must be imported separately and its lengthy
name can be assigned a short alias by an "import as" statement.
A message box is created by supplying a box title and the message to be
displayed as the two arguments to one of these methods.
showinfo() ok
showwarning() ok
showerror() ok
askquestion() yes and no
askofcancel() ok returns 1 and cancel
askyesno() yes returns 1 and no
askretrycancel() retry returns 1 and cancel
Those methods that produce a message box containing a single OK button return no
value when the button gets clicked by the user. Those that do not return a value
can be used to perform conditional branching by testing that value.from tkinter import *
import tkinter.messagebox as box
window=Tk()
window.title('Message')
def dialog():
var=box.askyesno('Message', 'Proceed?')
if var==1:
box.showinfo('Yes Box', 'Proceeding...')
else:
box.showwarning('No box', 'Canceling...')
btn=Button(window, text='Click', command=dialog)
btn.pack(padx=150, pady=50)
window.mainloop()
Options can be added as a third argument to these method calls. For example, add
'type=abortretryignore' to get three buttons.
Gathering Entries
An entry widget provides a single-line input field in an application where the
program can gather entries from the user. An entry object is created by
specifying the name of its parent container, such as a window or frame name, and
options as arguments to an Entry() constructor. Each option is specified as an
option=value pair. Popular options are listed below, together with a brief
description.
bd border width in pixels(default is 2)
bg background color
fg foreground color used to render the text
font font for the text
highlightcolor border color when in focus
selectbackground background color of selected text
selectforeground foreground color of selected text
show hide password characters with show='*'
state enabled status of Normal or Disabled
width entry width in letters
Multiple widgets can be grouped in frames for better positioning. A frame object
is created by specifying the name of the window to a Frame() constructor. The
frame's name can then be specified as the first argument to the widget
constructors to identify it as that widget's container.
When actually adding widgets to the frame you can specify which side to pack
them to in the frame with Top, Bottom, left, or Right constants. For example,
'entry.pack(side=Left)'.
Typically, an entry widget will appear alongside a label describing the type of
input expected there from the user, or alongside a button widget that the user
can click to perform some action on the data they have entered, so positioning
in a frame is ideal.
Data currently entered into an entry widget can be retrieved by the program
using that widget's get() method.from tkinter import *
import tkinter.messagebox as box
window=Tk()
window.title('Entry Box')
frame=Frame(window)
entry=Entry(frame)
def dialog():
box.showinfo('Greetings', 'Welcome ' + entry.get())
btn=Button(frame, text='Enter Name', command=dialog)
btn.pack(side=RIGHT, padx=5)
entry.pack(side=LEFT)
frame.pack(padx=20, pady=20)
window.mainloop()
Use a label widget instead of an Entry widget if you want to display text that
the user cannot edit.
Listing Options
A Listbox widget provides a list of items in an application from which the user
can make a selection. A listbox object is created by specifying the name of its
parent container, such as a window or frame name, and options as arguments to a
Listbox() constructor. Popular options are listed below, together with a brief
description.
bd border width in pixels(default is 2)
bg background color
fg foreground color used to render the text
font font for the text
height number of lines in list(default is 10)
selectbackground background color of selected text
selectmode single(the default) or multiple selections
width Listbox width in letters(default is 20)
yscrollcommand attach to a vertical scrollbar
Items are added to the listbox by specifying a list index number and the item
string as arguments to its insert() method.
You can retrieve any item from a listbox by specifying its index number within
the parentheses of its get() method. Usefully, a listbox also has a
curselection() method that returns the index number of the currently selected
item, so this can be supplied as the argument to its get() method to retrieve
the current selection.from tkinter import *
import tkinter.messagebox as box
window=Tk()
window.title('Listbox')
frame=Frame(window)
listbox=Listbox(frame)
listbox.insert(1, 'C++')
listbox.insert(2, 'Python')
listbox.insert(3, 'R')
def dialog():
box.showinfo('Selection', 'Your Choice:')
listbox.get(listbox.curselection())
btn=Button(frame, text='Choose', command=dialog)
btn.pack(side=RIGHT, padx=5)
listbox.pack(side=LEFT)
frame.pack(padx=30, pady=30)
window.mainloop()
If the selectmode is set to MULTIPLE, the curselection() method returns a tuple
of the selected index numbers.
Polling Radio Buttons
A Radiobutton widget provides a single item in an application that the user may
select. Where a number of radio buttons are grouped together, they user may
only select any one item in the group. With tkinter, radio button objects are
grouped together when they nominate the same control variable object to assign a
value to upon selection. An empty string variable object can be created for this
purpose using the "stringvar()" constructor or an empty integer variable object
using the "intvar()" constructor.
A radio button object is created by specifying four arguments to a
"Radiobutton()" constructor.
1. name of the parent container, such as the frame name.
2. text for a display label, specified as a text=text pair.
3. control variable object, specified as a variable=variable pair.
4. value to be assigned, specified as a value=value pair.
Each radio button object has a select() method that can be used to specify a
default selection in a group of radio buttons when the program starts. A string
value assigned by selecting a radio button can be retrieved from a string
variable object by using its get() method.from tkinter import *
import tkinter.messagebox as box
window=Tk()
window.title('Radio Button')
frame=Frame(window)
book=StringVar()
radio_1=Radiobutton(frame, text='Javascript', variable=book, value='web')
radio_2=Radiobutton(frame, text='C++', variable=book, value='microprocessor')
radio_3=Radiobutton(frame, text='R', variable=book, value='statistics')
radio_1.select()
def dialog():
box.showinfo('Selection', 'Choice: ' + book.get())
btn=Button(frame, text='Choose', command=dialog)
btn.pack(side=RIGHT, padx=5)
radio_1.pack(side=LEFT)
radio_2.pack(side=LEFT)
radio_3.pack(side=LEFT)
frame.pack(padx=30, pady=30)
window.mainloop()
A Radiobutton object has a deselect() method that can be used to cancel a
selection programmatically.
Checking Boxes
A Checkbutton widget provides a single item in an application that the user may
select. Where a number of check buttons appear together the user may select one
or more items. Check button objects nominate an individual control variable
object to assign a value to whether checked or unchecked. An empty string
variable object can be created for this using the stringvar() constructor or an
empty integer variable object using the intVar() constructor.
A check button object is created by specifying five arguments to a
Checkbutton() constructor.
1. name of the parent container, such as the frame name
2. text for a display label, as a text=text pair
3. control variable object, as a variable=variable pair
4. value to assign if checked, as an onvalue=value pair
5. value to assign if unchecked, as an offvalue=value pair
An integer value assigned by a check button can be retrieved from an integer
variable object by its get() method.from tkinter import *
import tkinter.messagebox as box
window=Tk()
window.title('Check Button')
frame=Frame(window)
var_1=IntVar()
var_2=IntVar()
var_3=IntVar()
book_1=Checkbutton(frame, text='C++', variable=var_1, onvalue=1, offvalue=0)
book_2=Checkbutton(frame, text='R', variable=var_2, onvalue=1, offvalue=0)
book_3=Checkbutton(frame, text='Javascript', variable=var_3, onvalue=1, offvalue=0)
def dialog():
str='Your Choice:'
if var_1.get()==1:str+='For Microprocessors'
if var_2.get()==1:str+='For Statistics'
if var_3.get()==1:str+='For Web Sites'
box.showinfo('Selection', str)
btn=Button(frame, text='Choose', command=dialog)
btn.pack(side=RIGHT, padx=5)
book_1.pack(side=LEFT)
book_2.pack(side=LEFT)
book_3.pack(side=LEFT)
frame.pack(padx=30, pady=30)
window.mainloop()
A Checkbutton object has select() and deselect() methods that can be used to
turn the state on or off. For example, check_1, select(). The state of any
Checkbutton object can be reversed by calling its toggle() method.
Adding Images
With the tkinter module, images in gif or pgm/ppm file formats can be displayed
on label, button, text, and canvas widgets using the PhotoImage() constructor to
create image objects. This simply requires a single file= argument to specify
the image file. It also has a subsample() method that can scale down a specified
image by stating a sample value to x= and y= arguments. For example, values of
x=2, y=2 samples every second pixel so the image object is half the size of the
original.
Once an image object has been created, it can be added to a label or button
constructor statement by an image= option. Text objects have an image_create()
method with which to embed an image into the text field. This requires two
arguments to specify location and image=. For example, '1.0' specifies the
first line and first character.
Canvas objects have a create_image() method that requires two arguments to
specify location and image=. Here, the location sets the x,y coordinates on the
canvas at which to paint the image.from tkinter import *
window=Tk()
window.title('Image')
img=PhotoImage(file='galaxy.gif')
label=Label(window, image=img, bg='yellow')
small_img=PhotoImage.subsample(img, x=2, y=2)
btn=Button(window, image=small_img)
txt=Text(window, width=25, height=7)
txt.image_create('1.0', image=small_img)
txt.insert('1.0', 'Galaxies Are Cool')
can=Canvas(window, width=100, height=100, bg='cyan')
can.create_image((50,50), image=small_img)
can.create_line(0,0,100,100, width=25, fill='yellow')
label.pack(side=TOP)
btn.pack(side=LEFT, padx=10)
txt.pack(side=LEFT)
can.pack(side=LEFT, padx=10)
window.mainloop()
Notice that the text method is image_create() but the canvas method is
create_image(). Text and canvas widgets are both powerful and flexible.
Summary
The tkinter module can be imported into a python program to provide attributes
and methods for windowed applications. Every tkinter program must begin by
calling Tk() to create a window and call its mainloop() method to capture
events.
The window object's title is specified by its title() method. A label widget is
created by specifying the name of its parent container and its text as arguments
to the Label() constructor.
Widgets can be added to an application using the pack(), grid(), or place()
geometry managers. A button widget is created by specifying the name of its
parent container, its text, and the name of a function to call when the user
pushes it, as arguments to the Button() constructor.
The tkinter.messagebox module can be imported into a python program to provide
attributes and methods for message boxes. Message boxes that ask the user to
make a choice return a value to the program for conditional branching.
The Frame() constructor creates a container in which multiple widgets can be
grouped for better positioning. The Entry() constructor creates a single line
text field whose current contents can be retrieved by its get() method.
Items are added to the Listbox object by its insert() method, and retrieved by
specifying their index numbers to its get() method. Radiobutton and Checkbutton
objects store values in the StringVar or IntVar object nominated by their
variable attribute.
The PhotoImage() constructor creates an image object that has a subsample()
method that can scale down an image. Images can be added to Button and Label
objects, embedded in Text objects, and painted on Canvas objects.