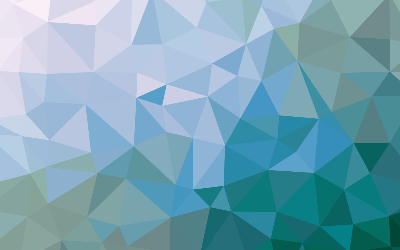
Accessing Files in Python
These are my notes on accessing files in python.
Accessing Files
The _builtins_ module can be examined using the "dir()" function to reveal that
it contains a file object that defines several methods for working with files,
including "open()", "read()", "write()", and "close()".
Before a file can be read or written, it must always be opened using the
"open()" method. This requires two string arguments to specify the name and
location of the file, and one of the following "mode" specifiers in which to
open the file:
r open an existing file to read
w open an existing file to write
a Append text and opens/creates a text file for writing at the end of the
file
r+ open a text file to read from or write to
w+ open a text file to write to or read from
a+ open or creates a text file to read from or write to at the end of the
file
Where the mode includes "b" after any of the file modes listed above, the
operation relates to a binary file rather than a text file: rb or w+b
Once a file is opened and you have a file object, you can get various details
related to that file from its properties.
name name of the opened file
mode mode in which the file was opened
closed status boolean value of True or False
writeable() write permission boolean value of True or False
File mode arguments are string values so must be surrounded by quotes. You can
also use a "readlines()" method that returns a list of all lines.file = open('document.txt', 'w')
print('File Name:', file.name)
print('File Open mode:', file.mode)
print('Readable:', file.readable())
print('Writable:', file.writable())
def get_status(f):
if (f.closed != False):
return 'Closed'
else:
return 'Open'
print('File Status:', get_status(file))
file.close()
print('\nfile Status:', get_status(file))
If your program tries to open a non-existant file in "r" mode, the interpreter
will report an error.
Reading and Writing Files
Once a file has been successfully opened it can be read or added to, or new text
can be written in the file, depending on the mode, specified in the call to the
open() method. Following this, the open file must then always be closed by
calling the close() method.
As you might expect, the read() method returns the entire content of the file,
and the write() method adds content to the file. You can quickly read the entire
contents in a loop, iterating line by line. Writing to an existing file will
automatically overwrite its contents. LaTeX = "LaTeX is used to add math to the web\n"
LaTeX += "Fractions are made using this notation: \\frac[4}{5}\n"
LaTeX += "Exponents are made by: a^{4n}\n"
LaTeX += "Subscripts are made by: a_{4n}\n"
file = open('LaTeX.txt', 'w')
file.write(LaTeX)
file.close()
file = open('LaTeX.txt', 'r')
for line in file:
print(line, end = '')
file.close()
Suppress the default newline provided by the print() function where the strings
themselves contain newlines. You can also use the obkect's readlines() method
that returns a list of all lines in a file - one line per element.
Updating File Strings
A file object's read() method will, by default, read the entire contents of the
file from the very beginning, at index position zero, to the very end - at the
index position of the final character. Optionally, the read() method can accept
an integer argument to specify how many characters it should read.
The position within the file, from which to read or at which to write, can be
finely controlled using the file object's seek() method. This accepts an integer
argument specifying how many characters to move position as an offset from the
start of the file.
The current position within the file can be discovered at any time by calling
the file object's tell() method to return an integer location.
When working with file objects it is good practice to use the Python "with"
keyword to group the file operational statements within a block. This technique
ensures that the file is properly closed after operations end, even if an
exception is raised on the way, and is much shorter than writing equivalent "try
except" blocks.
Storing Data
In Python, string data can easily be stored in text files using the
techniques demonstrated in the previous examples. Other data types, such as
numbers, lists, or dictionaries, could also be stored in text file but would
require conversion to strings first. Restoring that stored data to their
original data type on retrieval would require another conversion. An easier
way to achieve data persistence of any data object is provided by the pickle
method.
The process of pickling objects stores a string representation of an object that
can later be unpickled to its former state, and is a very common Python
programming procedure.
An object can be converted for storage in a file by specifying the object and
file as arguments to the pickle object's dump() method. It can later be restored
from that file by specifying the file name as the sole argument to the pickle
obkect's load() method.
Unless the storage file needs to be human readable for some reason, it is more
efficient to use a machine readable binary file.
Where the program needs to check for the existence of a storage file, the "os"
module provides a path object with an isfile() method that returns True if a
file is specified within its parentheses is found.
import pickle, os
if not os.path.isfile('pickle.dat'):
data = [0,1]
data[0] = input('Enter Topic:')
data[1] = input('Enter Series:')
file = open('pickle.dat', 'wb')
pickle.dump(data,file)
file.close()
else:
file = open('pickle.dat', 'rb')
data = pickle.load(file)
file.close()
print('\nWelcome Back To:', data[0], data[1])