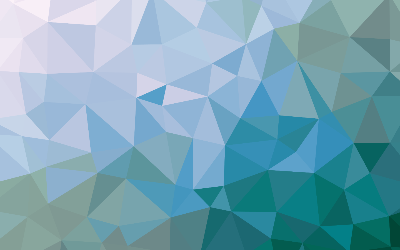
Processes in Linux
These are my notes on processes in linux.
Intro
Prcoesses are how Linux organizes the different programs waiting for their turn
at the cpu.
How processes Work
When a system starts up, the kernel initiates a few of its own activities as
processes and lauches a program called init. It runs a series of shell scripts
(located in /etc) called init scripts, which start all the system services. Many
of these services are implemented as daemon programs, programs that just sit in
the background and do their thing without having any user interface. So, even if
we are not logged in, the system is at least a little busy performing routine
stuff.
The fact that a program can launch other programs is expressed in the process
scheme as a parent process producing a child process. The kernel maintains
information about each process to help keep things organized. For example, each
process is assigned a number called a process ID. PID's are assigned in
descending order, with init always getting PID 1. The kernel also keeps track of
the memory assigned to each process, as well as the processes' readiness to
resume execution. Like files, processes also have owners and user IDs, effective
user IDs, and so on.
Viewing Processes
The most commonly used command to view processes is 'ps'. The 'ps' program has a
lot of options but it is used like this:ps
The result in this example lists two processes, which are bash and ps. As we can
see, ps does not show us very much, just the processes associated with the
current terminal session. To see more, we need to add some options.
If we add an option, we can get a bigger picture of what the system is doing.ps x
Adding the x option tells ps to show all of our processes regardless of what
terminal they are controlled by. The presence of a ? in the TTY column indicates
no controlling terminal. Using this option, we see a list of every process that
we own.
Because the system is running a lot of processes, ps produces a long list. It is
often helpful to pipe the output from ps to less for easier viewing. Some option
combinations also produce long lines of output, so maximizing the terminal
emulator window might be a good idea too.
Processor States
R Running
S Sleeping
D Uninterruptible sleep
T Stopped
Z Defunct or zombie process
< High priority process
N Low priority process
The process state may be followed by other characters. These indicate various
exotic process characteristics. Another popular set of options is aux. This
gives us even more information. ps aux
This set of options displays the processes belonging to every user. Using the
options without the leading dash invokes the command with BSD style behavior.
The linux version of ps can emulate the behavior of the ps program found in
different unix implementations.
Viewing Processes Dynamically
While the ps command can reveal a lot about what the machine is doing, it
provides only a snapshot of the machine's state at the moment the ps command is
executed. To see a more dynamic view of the machine's activity, we use the top
command.top
The top program displays a continuously updating display of the system processes
listed in order of process activity. The name top comes from the fact that the
top program is used to see the top processes of the system. The top display
consists of two parts, a system summary at the top of the display, followed by a
table of processes sorted by cpu activity.
The top program accepts a number of keyboard commands. The two most interesting are h, which displays the program's help screen, and q, which quits top.
Both major desktop environments provide graphical applications that display
information similar to top, but top is better than the graphical versions
because it is faster and it consumes far fewer system resources. After all, our
system monitor program should not be the source of the system slowdown that we
are trying to track.
Controlling Processes
Now that we can see and monitor processes, let us gain some control over them.
Type gedit to open that program. Now type control-c to interrupt the program. It
should close. Most command line programs can be closed in this way.
If we want the shell program back on top but still want another program to run,
we can put it into the background. To launch a program so that it is immediately
placed in the background, we follow the command with a & character.gedit &
After entering the command, the window appeared and the shell prompt returned. A message will appear which is a shell feature called job control. With this
message, the shell is telling us we have started a job number and what its PID
is. If we then run ps, we can see this process.
The shell's job control facility also gives us a way to list the jobs that have
been launched from our terminal. Using the jobs command, we can see this list.jobs
This shows us a list of the current jobs running.
Returning a Process to the Foreground
A process in the background is immune from terminal keyboard input, including
any attempt to interrupt it with control-c. To return a process to the
foreground, use the fg command:// run jobs command to get the job number of process you want
jobs
// then use the job number with the fg command
fg %1 // 1 is the job number we want to bring to foreground
The fg command followed by a percent sign and then job number is how you use it. If we have only one background, the job number is optional.
Stopping A Process
Sometimes we will want to stop a process without terminating it. This is the
same as pausing it. This is often done to allow a foreground process to be moved
to the background. To stop a foreground process and place it in the background,
press control-z.
After stopping the process, we can verify that the program has stopped by trying
to use it. It will not work. We can either continue the program's execution in
the background, using the fg command, or resume the program's execution in the
background with the bg command. bg %1
As with the fg command, the job number is optional if there is only one job.
Moving a process from the foreground to the background is handy if we launch a
graphical program from the command line but forget to place it in the background
by appending the trailing &.
There are two reasons why we would want to launch a graphical program from the
command line. The program you want to run might not be listed on the window
manager's menu. By launching a program from the command line, you might be able
to see error messages that would otherwise be invisible if the program were
launched graphically.
Sometimes, a program will fail to start up when launched from the graphical
menu. By launching it from the command line instead, we may see an error message
that will reveal the problem. Also, some graphical programs have interesting and
useful command line options.
Signals
The kill command is used to kill processes. This allows us to terminate programs
that need killing.kill 16606
We get the process ID from one of the ways mentioned before, then use that ID
with the kill command. We could have also used a job number if we wanted to go
that route.
While this is straightforward, there is more to it than that. The kill command
does not exactly kill processes, it sends them signals. Signals are one of
several ways that the operating system communicates with programs. We have
already seen signals in action with the use of control-c and control-z.
When the terminal receives one of these keystrokes, it sends a signal to the
program in the foreground. In the case of control-c, a signal called INT
(interrupt) is sent. Programs, in turn, listen for signals and may act upon them
as they are received. The fact that a program can listen and act upon signals
allows a program to do things such as save work in progress when it is sent a
terminating signal.
Sending Signals to Processes
The kill command is used to send signals to programs. Its most common syntax is
this:kill -signal (PID)
If no signal is specified on the command line, then the terminate signal is sent
by default.
1 hup hang up
2 int interrupt
9 kill kill
15 term terminate
18 cont continue
19 stop stop
20 tstp terminal stopkill -1 16606
In this example, sent the process a hup singal with the kill command. It
terminates and the shell indicates that the background process has received a
hang up signal. We may need to press enter a couple of times before the message
appears. Note that the signal may be specified either by number or by name.
Sending Signals to Multiple Processes
It is also possible to send signals to multiple processes matching a specified
program or username by using the killall command.killall -u user -signal name
To demonstrate, we will start a couple of instances of programs and terminate
them.killall gedit
As with the kill command, you must have superuser privileges to send kill
signals to processes that do not belong to you.
Shutting Down the System
The process of shutting down the system involves the orderly termination of all
the processes on the system, as well as performing some vital housekeeping
chores before the system powers off. Four different commands can perform this
function.
halt
poweroff
reboot
shutdown
The first three are self explanatory and are generally used without any command
line options. The shutdown command is more interesting. With it, we can specify
which of the actions to perform and provide a time delay to the shutdown event.
Most often it is used like this to halt the system:shutdown -h now
We can also use it like this:shutdown -r now
The delay can be specified in a variety of ways. Once the shutdown command is
executed, a message is broadcast to all logged in users warning them of the
impending event. Because monitoring processes is an important system
administration task, there are a lot of commands for it.
pstree outputs a process list arranged in a tree like pattern
vmstat outputs a snapshot of system resource usage
xload graphical program that draws a graph showing system load over time
tload same as xload but draws the graph in the terminal
Most modern systems feature a mechanism for managing multiple processes. Linux provides a rich set of tools for this purpose. Unlike other systems, Linux
relies primarily on command line tools for process management. Though there are
graphical process tools for Linux, the command line tools are greatly preferred
because of their speed and light footprint.