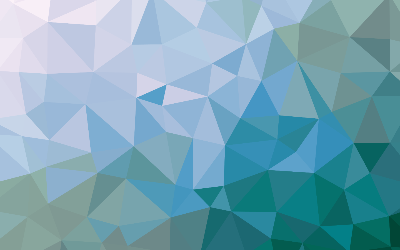
Encapsulating Data in Python
These are my notes on encapsulating data in python.
A class is a specified prototype describing a set of properties that
characterize an object. Each class has a data structure that can contain both
functions and variables to characterize the object.
The properties of a class are referred to as its data members. Class function
members are known as its methods, and class variable members are known as its
attributes.
Class members can be referenced throughout a program using dot notation,
suffixing the member name after the class name, with syntax of
class-name.method-() or class-name.attribute-name.
A class declaration begins with the class keyword, followed by a
programmer-specified name then a colon. Next, indented statements optionally
specifying a class document string, class variable attribute declarations, and
class method definitions, so the class block syntax looks like this:
class ClassName:
'''class-documentation-string'''
class-variable-declarations
class-method-definitions
The class declaration, which specifies its attributes and methods, is a
blueprint from which working copies can be made.
All variables declared within method definitions are known as instance variables
and are only available locally within the method in which they are declared.
They cannot be directly referenced outside the class structure.
Typically, instance variables contain data passed by the caller when an instance
copy of the class is created. As this data is only available locally for
internal use, it is effectively hidden from the rest of the program. This
technique of data encapsulation ensures that data is securely stored within the
class structure and is the first principle of object oriented programming.
All properties of a class are referenced internally by the dot notation prefix
self-so an attribute named sound is self.sound. Additionally, all method
definitions in a class must have self as their first argument, so a method named
talk is talk(self).
When a class instance is created, a special _init_(self) method is automatically
called. Subsequent arguments can be added in its parentheses if values are to be
passed to initialize its attributes.
It is conventional to begin class names with an uppercase character, and object
names with lowercase. The class documentation string can be accessed via the
special__doc__ docstring attribute with Classname__doc__. While a program class
cannot perfectly emulate a real-world object, the aim is to encapsulate all
relevant attributes and actions.
Objects
An instance of a class object is simply a copy of the prototype created by
calling that class name's constructor and specifying the required number of
arguments within its parentheses. The call's arguments must match those
specified by the __init__() method definition, other than a value for the
internal self argument.
The class instance object returned by the constructor is assigned to a variable
using the syntax instance-name=ClassName(args).
Dot notation can be used to reference the methods and class variable attributes
of an instance object by suffixing their name as instance-name.method-name() or
instance-name.attribute.name.
Typically, a base class can be defined as a python module file so it can be
imported into other scripts where instance objects can be easily created from
the master class prototype.
A constructor creates an instance of a class and is simply the class name
followed by parentheses containing any required argument values. You must not
pass an argument value for the self argument, as this is automatically
incorporated by python.
Class Attributes
An attribute of a class can be added, modified, or removed at any time using dot
notation to address the attribute. Making a statement that assigns a value to an
attribute will update the value contained within an existing attribute or create
a new attribute of the specified name containing the assigned value:
instance-name.attribute-name=value
del instance-name.attribute-name
Alternatively, you can use the following built in function to add, modify, or
remove an instance variable:
getattr(instance-name, 'attribute-name') - return the attribute value of the
class instance.
- hasattr(instance-name, 'attribute-name') - return True if the attribute value
- exists in the instance, otherwise return False.
- setattr(instance-name, 'attribute-name', value) - update the existing attribute
- value or create a new attribute in the instance.
- delattr(instance-name, 'attribute-name') - remove the attribute from the
- instance.
The name of attributes autmatically supplied by python always begin with an
underscore character to notationally indicate privacy so these should not be
modified or removed. You can add your own attributes named in this way to
indicate privacy if you wish, but in reality these can be modified like any
other attribute.
The attribute name specified to these built in functions must be enclosed within
quotes.
Built-In Attributes
Each python class is automatically created with a number of built-in private
attributes whose values can be referenced using dot notation. For example, with
class-name.__doc__ to see the document string value of a specified class name.
The built-in dir() function can be used to display a list of all the built-in
attributes in a class specified within its parentheses by testing whether each
attribute name begins with an underscore.
The built-in __dict__ attribute contains a namespace dictionary of class
component keys and their associated values. The dictionary of a base class
includes its default __init__() method, and all class methods and attributes.
The dictionary of a class instance includes its instance attributes.
The function values stored in the dictionary are the machine addresses where the
functions are stored.
A class instance is first created so the __init__() method has been called to
increment the count value before the dictionary gets listed. The __weakref()__
attribute is simply used internally for automatic garbage collection of weak
references in the program for efficiency.