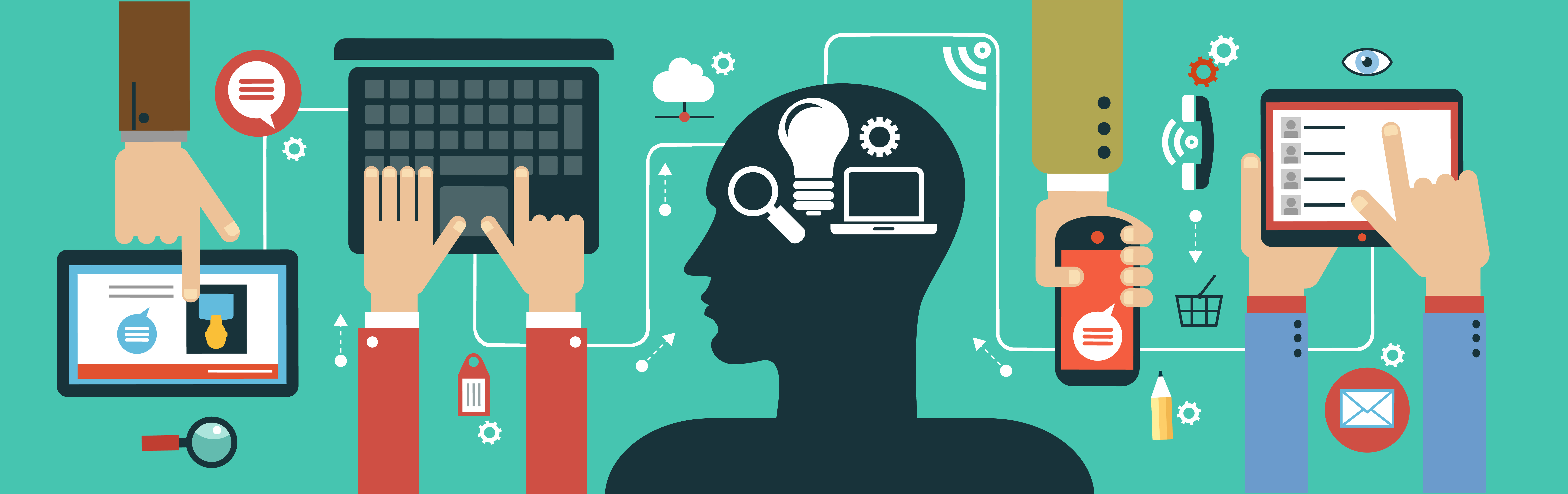
Sending Web Responses in Python
These are my notes on sending web responses in python.
Sending Web Responses
Whenever a user asks to view an online web page in their browser it requests the
page from the web server, and receives the page in response, via the http
protocol.
Where a requested web page address is an html document, with an .html file
extension, the web server response will return that file to the browser so its
contents can be displayed.
Where python is installed on the computer hosting the web server, the web server
can be configured to recognize python scripts and call upon the python
interpreter to process script code before sending an html response to the web
server, for return to the browser client.
A python script requested by a web server can generate a complete html document
response by describing the content type on the first line as
"content-type:text/html/r/n/r/n" so the web browser will parse the markup
content for display on the screen.
The content-type output description gets sent as an http header to the browser,
and must appear on the first line.
Enclose html attribute values within double quote marks so they do not get
confused with the single quote marks enclosing the strings.
Handling Values
Values can be passes to a python script on the web server when the browser makes
an http request. Those values can be used in the script and echoed in a response
returned to the browser.
Python's cgi module can be used to easily handle data passes from the web
browser by an http request. This provides a "fieldstorage()" constructor that
creates an object storing the passed data as a dictionary of key:value pairs.
Any individual value can then be retrieved by specifying its associated key
name within the parentheses of the "fieldstorage" object's "getvalue()" method.
The browser can submit data to the script using a "get" method that simply
appends key:value pairs to the script's url address. These follow a ? question
mark character after the file name and multiple pairs must be separated by an &
ampersand character.
The request string in the get method is lmited to 1024 characters so is
unsuitable for passing lots of key:value pairs. The values appended to the url
are visible in the browser address field of the response, so the get method
should not be used to send passwords or other sensitive data values to the web
server.
Submitting Forms
Passing data from a web page to a web server using the get method to append
key:value pairs to a url is simple, but has some limitations - the request
string length cannot exceed 1024 characters, and the values appear in the
browser address field.
As a more reliable alternative, the browser can submit data to the data script
using a post method that sends the information to the web server as a separate
message not appended to the url.
Python's cgi module can be used to handle form data sent from the browser with
the post method in exactly the same way as data passed from the browser with the
get method. This module's fieldstorage() constructor can create an object to
store the posted data as a dictionary of key:value pairs for each form field.
Any individual value can be retrieved by specifying its associated key name to
the object's getvalue() method.
Providing Text Areas
Large amounts of user-input text data can be passed from a web page to a web
server using the html <textarea> tags and the form post method. This tag has no
value attribute so a default value may not be provided. It is useful to have the
python script test whether the text area has been left blank and provide a
default value when the user has entered no text.
The average character width may vary between browsers - so the physical size of
the text area field may vary too. You can use the F12 developer tools in your
web browser to examine the http request and response components.
Checking Boxes
An html form can provide a visual checkbox on/off switch that the user can
toggle to include its associated data for submission to the web server. The
python script nominated to handle the form data can test whether each checkbox
has been checked, simply by testing if a value has been received from the
checkbox of that name.
The "checked" keyword can be added in any checkbox <input> element to make it
checked by default.
Choosing Radio Buttons
An html form can provide a 'radio button" group from which the user can select
just one button to submit its associated data to the web server. Unlike
checkboxes, radio buttons that share a common name are mutually exclusive, so
when one button in the group is selected, all other buttons in that group are
switched off. The python script nominated to handle the form data can test the
value submitted for the radio button group name and supply an appropriate
response.
Always include a "checked" attribute to automatically select one button in each
radio button group - to include a default choice. Radio button elements resemble
the buttons on old radios where each button selected a particular radio station.
Selecting Options
An html form can provide a drop-down list of possible options from which the
user can select a single option to include its associated data for submission to
the web server. The submitted value can then be retrieved by specifying its
associated list key name within the parentheses of the "fieldstorage" object's
"getvalue()" method.
Typically, the first list option will be selected for submission by default,
unless you click open the drop-down list and select an alternative. You can
include the selected attribute in an <option> tag to automatically select one
option in each list - to include a default choice.
Updating Files
An html form can provide a file selection facility, which calls opon the
operating system's "choose file" dialog, to allow the user to browse their local
file system and select a file. To enable this facility the html <form> tag must
include an 'enctype" attribute specifying the encoding type as
multiple/form-data.
The full path address of the file selected for upload is a value stored in the
fieldstorage object list that can be accessed using its associated key name.
Usefully, the file name can be stripped from the path address by the "os"
module's "path.basename()" method.
Notice that binary file mode is used here to copy the uploaded file.
Summary
Python can be installed on a web server host to process script code before
sending a response to a web browser client. A server-side python script can
generate an html document by describing the content type as
"content-type:text/html/r/n/r/n".
The cgi module provides a fieldstorage() constructor to create an object for
storing submitted data as key:value pairs. Any value stored in a fieldstorage
object can be retrieved by specifying its key name to the object's getvalue()
method.
The browser can send data to a script using the get method that appends
key=value pairs to its url address after a ? question mark. Multiple key=value
pairs of data can be submitted using the get method if each pair is separated by
an & ampersand character.
The get method request string length cannot exceed 1024 characters and will be
visible in the browser address field. The browser can send data to a script
using the post method that submits key:value pairs as a separate message.
Data submitted from an html form can be stroed in a fieldstorage object as
key:value pairs for each form field. A server-side python script can provide
default values for submitted html form fields that the user has left blank.
Checkbox fields of an html form that are unchecked do not get submitted to the
web server. A selected radio button in a group provides the value to be
associated with the group name when the form gets submitted.
A selected item in a drop-down list provides the value to be associated with the
list name when the form gets submitted. An html form can allow file uploads only
if its "enctype" attribute specifies its encoding type as "multiple/form-data".