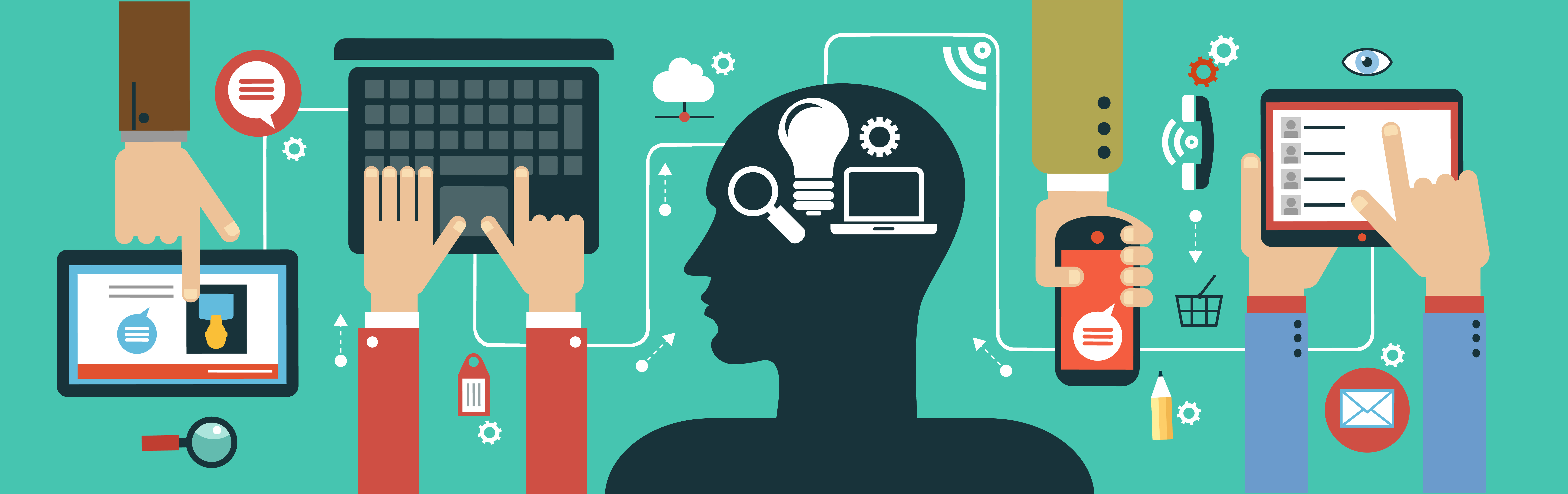
Learning Powershell Scripting.
This is a guide for learning Powershell scripting.
Table of Contents
Powershell For Beginners
It has been around a long time and has been through several versions already. Many information technology people have waited to become familiar with this tool. They did so because many were not sure it would continue to be supported in the future.
Well we now know that not only has it continued to be supported but it is the preferred way by many to administer a Windows environment. So continue with me and we will discuss the basics so that you can go on and make this a long learning endeavor.
Powershell is a command oriented scripting environment. This means that you can open up a console and run a series of commands to accomplish various tasks. It also means that you can run a series of commands as a script over and over.
Scripting is very important for your environment as it lets you automate certain tasks over and over.
If you work in information technology then you need to start learning this as soon as possible. There is much you can do with it and there will be even more you can do with it in the future. It will help you earn more money in your career too. Who can really argue with that?
Using The Help System
So you have convinced yourself to start learning Powershell for beginners. Now what? The single best piece piece of advice I can give is to learn the help system. Let me give you a short example. I was asked the other day to do something.
I had no idea at the time how to do it. So I went to my desk and opened up the console and started the help system. From a console it is [get-help] and looks like this.
I had a slight idea of what command I would need and looked at the help options for it. I was actually wrong the first time so then tried another option which would work eventually.
The important thing to learn here is that using the help system allows you to see the options and arguments with helpful descriptions to see if a command might work for you.
I wanted to import a third party module and the help showed me how to do it.
To start lets make sure you have the current version of help so you have all of its benefits. Type the command in yellow at your prompt.
The help system will even give examples. This is especially handy like when your totally unfamiliar with a command. Below is an example.
As you can see it shows two different examples of how to use this command. There were actually lots of examples but the following ones get more and more niche.
Powershell Terminology
There are several terms you should know in this Powershell for beginners guide. They are not difficult at all but will help so you know what the help system is trying to tell you.
- Cmdlet
- Parameter
- Alias
- Function
- Variable
- Module
A Cmdlet is the command you will be typing. It has a nice naming convention that will be of the verb-noun format. The verb parts are actions that do something. Example of verbs are import, set, get, and stop.
The noun parts are what the verbs act upon. This could be module, process, help, or service.
Parameters customize what a Cmdlet will do. You will see the parameters of a Cmdlet when using the help for it. To use a parameter you will use a dash, a space, the parameter name, another space, and a value.
An Alias is another form of a Cmdlet that you use a lot. It is a shortcut basically. You can make an Alias by the below syntax.
You can see from the above command that I made a shortcut to calc.exe and named it just c. So then when I typed c at the prompt my calculator opened. You can do this for any Cmdlet to save you time so make use of it when the need arises.
A Function is a piece of code that is re-usable. If you have any programming experience at all then you are already familiar with this terminology.
Variables are just the same as they are in programming languages. They hold values. It is like an empty box until it has a value.
Modules are a series of commands that are related to other products usually.
Piping Commands
Powershell, just like other tools, can send the output of one command into another command. This is called piping. In the syntax you do it with a vertical bar just like with Unix. Look at the following example.
I have a simple text file on my desktop with some content inside. I am going to run two different commands which are [Get-Content] and [Measure-Object].
However, I am going to do it in one line and pipe the output of [Get-Content] into the [Measure-Object] command so that it can do its magic.
I used the [Get-Content] Cmdlet or command followed by the path to my text file that I wanted to analyze with [Measure-Object]. I then used a vertical bar to pipe its output into [Measure-Object].
You should also notice that I used the parameters character, line, and word for [Measure-Object]. So pretty interesting for sure. This is just one example of piping Powershell for beginners.
Running Scripts
What is scripting? Scripting is the automation of tasks. You usually perform a task every time you type out a full working command in Powershell. To get scripting working you will need to set things up first.
By default Powershell is set to only run signed scripts. So to run your own scripts you will need to change this behavior. Run the following command below.
You will need to run this as an administrator. There are several other execution policies available but this is the one I recommend. You should not use the -unrestricted policy. It is too insecure.
Commenting Code
You should always comment whatever code or commands you type so that you or others will know exactly what is meant. Systems can break with the wrong commands so comment so that everyone knows exactly what is happening or will happen.
To comment you add a # on the line of code that you do not want to execute.
That's easy enough. Lets move on then.
Variables
As mentioned above somewhere, a variable is an empty container. It is waiting to hold a value so that it can be used. The data type of a variable can be a string, integer, decimal, or an array. Variables start with the $ symbol. Here is a basic example.
Yes this is very simple but it shows how the variables work. You can do this from the console , like I just did, or within a script. It works the same way. You could even pipe that into a text file and email it to your boss so he would know how much money to allocate for new equipment.
The [Write] command just writes the output to the screen so you all could see the results.
This is a string variable and I include it because it is done slightly different than a numerical variable. See below.
Now we are ready to use that variable any time we want.
If you are working in the console and you want to remove a variable then there are ways to do that.
You can see that when I sent the output to the screen at first the variable $Switches had a value of 2. After I removed the variable there was nothing to write to the screen.
Arrays
An Array is a container, like a variable, that holds a definable number of values that are of a single type. Every value inside an Array is called an element. They are indexed starting at 0. An Array can hold numerical or string values.
Look to my example below to see how to do it.
That should be easy enough to follow. I made two different arrays with data on the types of phones I have and the respective quantities.
Sorting And Filtering
Sorting and filtering the data that you have will be very important in Powershell for beginners. For example, you may want to do a certain action on only a select few items. You could run a command to find the few that you are looking for and do some action to them.
Now you may be thinking this is not a big deal if you have 10 total items in your list and you would be right then. If, however, you have 15,000 items in your list or spreadsheet then you will appreciate being able to do this in five minutes instead of weeks.
I will look again at my phone type array and look for anything that starts with "p".
Ok that is helpful. My phone list does not have too many in it but if it did this would take the same amount of time.
Lets sort my list of phone brands now. That is easily done and will be quite helpful at some point.
That is quick and very useful. As you can see I once again piped the output of my array into the sort command. If you want it to sort in descending order then just add the -descending parameter to sort-object.
You can also sort by length. I am sure this would be useful to someone.
There we go. It is pretty cool to be able to manipulate data like this. My phone brands are sorted by the length of their name.
Incorporating Logic
Conditional logic and decisions can be very important in Powershell for beginners to run scripts correctly. You might have certain conditions you need to be met before you want certain things to run. It could be your whole script or just portions.
There are many ways to do this but the most basic is through [if] and [if-else] loops. Here is a short [if] statement.
You can see I made a variable and called it [$magnets]. The next line is a statement where I test a basic condition. Since the condition passes (magnets = 5), the next part writes "You have enough magnets" to the screen.
Now that is okay but we can do better. Another version of an [if] loop is an [if-else] loop. This will let us deal with the problem if the condition is not met.
At first you can see that I had [$magnets] equal to 7 and when I ran the condition loop it passed and my success message appeared on the screen. Then, however, I went back and changed my variable [$magnets] to equal 4 and ran my conditional loop again.
Of course it failed and told me to go back and get more magnets. While this is basic it does show you some of the capabilities of basic programming that Powershell is capable of.
Looping
Looping your commands and part of your scripts is the next topic I want to talk about in this Powershell for beginners guide. If you have been exposed to any programming before then you knew I was going to get here eventually.
So what is a loop? Loops are commands or tasks that are repeated until they reach a condition that tells them to stop. So lets do some looping shall we?
Alright I admit it, I enjoy playing the Pokemon card game. My son and I play together now after he introduced me to it. These are some of my favorites that I play with and I needed something to put into an array.
We can also do a [for] loop. These are handy when you want to work on certain elements of an array or just run a loop a certain number of times. Here is an example where 0-13 are output to the screen.
The [for] loop looks a bit crazy I know if you have not seen them before but they work like a charm. The first part after [for] initializes the $i variable to 0. In the middle section the ending value of the $i variable is given.
The last part increments $i step by step. The rest of the line outputs the value of the $i variable one value and step at a time.
Another type of loop is the [while] loop. It can do a lot too. It just depends on what you need of course. Here is an example of counting down while the variable $n does not equal 0.
The last major type of loop is a [Do] loop. This is a handy loop when you need to run through your commands at least once. It allows you to loop while the condition is true or false.
So here is a small [Do] loop where I start my variable $n at 0, then increment for each time the loop runs, prints the value of the variable $n at each loop iteration, until finally the value of the variable $n reaches 10 and the loop exits.
Conclusion
This Powershell for beginners guide is really a small sampling of the basics. There is still a lot to cover and I hope you will go through all of these examples with me. Hopefully once you do that you will be able to come up with your own testing and experiments.
That is truly the fun of scripting and programming. Create your own scripts that do something you need to automate. It will be very fun for you and make you more efficient at whatever tasks you need to complete.
How to Run Powershell Commands
Running commands is the most basic way to use Powershell for beginners. It is how most people get started. We often have a quick task or need some information quickly on a remote machine.
These kind of uses are ideal for shells. They are ideal because they are quick and reliable. I was a Linux user long before Powershell existed. So when I needed to work on a Windows machine, the first thing I did was learn its shell environment.
Others may look for a tool with a gui and that is perfectly acceptable if that is what you want. However, when you get used to working with shells and their advantages, you never want to use anything else.
Running A Command
A Powershell command always has a verb-noun structure. Something like:get-content
It is done like this so it is relatively easy to know what the command does.
You can use the help system to learn how to use a particular command. This will probably be a very often used technique. There have been many times when I have forgot a command and just had to search for it.
So I do appreciate having this ability built in to the system. It has helped me greatly, as I am fairly forgetful at times.get-help get-content -examples
This will give you several details and a few good examples of the command. It does not go into the full depth of the command. That is an option though. The help system will also tell you of any required parameters.
If it is required, then you must supply that information in order for the command to run. If any parameter includes spaces then you will need to enclose it in quotation marks. Commands are also not case sensitive.
Using Aliases
Aliases can be a convenient way to remember long commands. It is one of the powershell essentials that will always be useful. Some of these long commands are just a pain. In these instances, using an alias makes a lot of sense. It is what they are for.
There is a nice way to find out if a command has an alias. So, if it is a pain to type or you type it a lot, then try this.get-alias -definition get-content
This does indeed tell us there is an alias for the "get-content" command.
It is "gc".
If you need to know what an alias is, then there is a way for that also. I know I can never remember anything but the most basic ones. I end up having to use this a lot. People like to use aliases when they write code.help gc
This will give you the full command and all of its details.
Useful Commands
For the rest of this chapter I want to go over some of the more useful commands. I want to do this so you will start to become familiar as soon as possible with them.get-childitem
This command will list files in a certain location. Use it like this.get-childitem c:/Documents
This is useful when you want to check if there is anything in a directory. You can use the "-recurse" option to list subfolders and files.copy-item
Thanks to Powershell's naming scheme, it is pretty easy to figure out what most commands do. This is a good example. Copying an item to another location is easy with this command.get-childitem c:\users\username -recurse | copy-item -destination c:\folder
This reads the information in with the previous command and copies those items to another folder. That's pretty useful!export-csv
When you need reports of things, this is the command to use. It will take the output of another command and make a "csv" file of it. Let's try a few.get-process | export-csv -path c:\export\process_report.csv
Put that in your shell and you will get a lot of information about the processes running on your system right now. It will be a file in the folder you just specified. We can make it look a little nicer so lets do that.get-process | export-csv -notypeinformation -path c:\export\process_report.csv
That extra flag will hide some of the useless stuff that comes with the file. Now let's customize the information. We can use the "select-object" command to select the columns we want. Let's just see the handles processname.get-process | select-object handles, processname |export-csv -path c:\export\process_report.csv
If we needed just those two columns for some reason, then we would have them. This is a useful command and I have also shown how to link different commands together.
Conclusion
In this chapter we started with how to run a basic command. These commands were basic forms with no parameters added. We then took a look at aliases and how they can be useful. Afterwards, we even went over how to create your own alias.
Then I showed some of these basic commands in action. They are all nice to know and can be very helpful in the right situations. I gave examples and even showed how to create some files with your reporting.