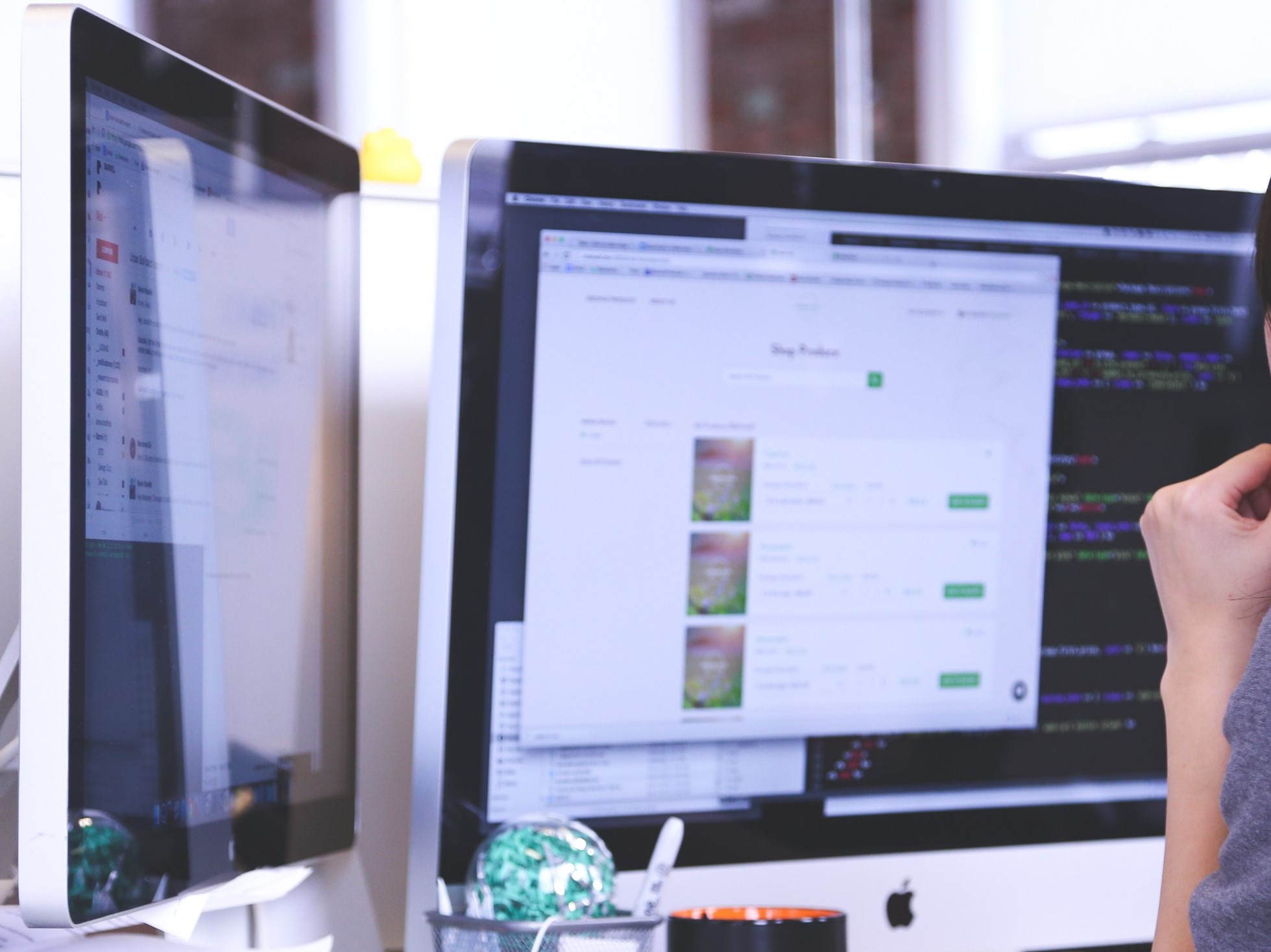
How To Use Pointers In C++
Pointers may not be the most intuitive of concepts. However, in C++, they can
still be useful in the right situation.
Introduction to Pointers
What is a pointer? A pointer is an operator in C++ that gives the address of a
variable. When variables are declared, they are given a section of memory large
enough to hold a value according to their data type. Common data types include
int, short, double, and float.
Each section of memory has a unique address. The address of a variable is the
starting address of that variable. A pointer allows you to request the address
of that variable. This is accomplished by using the <&> symbol in front of any
variable. The variable must already exist. Let us look at an example.#include <iostream>
using namespace std;
int main()
{
// creates an integer named number
int number = 100;
// shows current value of number
cout << "Number equals " << number << endl;
// shows current size of number
cout << "The size of number is " << sizeof(number) << endl;
// shows the address of the number variable
cout << "The address of number is at " << &number << endl;
return 0;
}
Copy and paste this into your editor or IDE and run it. It outputs 3 lines.
You will notice the address line is in hex format. This is common in C and C++
because they are low level languages.
Pointer Variables
Now that you have been introduced to pointers, we can be a bit more formal.
Pointer variables are the definition of pointers. The terms are used
interchangeably.
Pointers hold addresses of variables. Why does that matter? It matters because
we can then change variables using the address if we want to. Individual
pointers <point> to a piece of data held in a variable. These concepts are c++ essentials that you will need to understand.
Pointer variables are another way to use memory addresses. These addresses allow you to work with your data. Pointers may seem awkward to use. However, they are great for things like memory allocation.
Creating Pointers
Declaring pointer variables is easy for you to do.
double &number;
Note the <&> sign in front of the variable name. This indicates that it is a pointer. This says that it is a pointer to a <double> value. It could be any other data type too.#include <iostream>
using namespace std;
int main()
{
// creates an integer named number
int number = 100;
// create a pointer variable
int *pntr;
// stores the address into the pointer variable
pntr = &number;
// shows current value of number
cout << "Number equals " << number << endl;
// shows the size of the number variable
cout << "The size of number is " << sizeof(number) << endl;
// shows the address
cout << "The address of number is at " << &number << endl;
return 0;
}
Here I created integer and pointer variables. The pointer is then assigned the address of my integer. You will notice the <*> symbol and it is called the indirection operator. It is used to create a pointer and to work with the item it points to.
Initializing Pointer Variables
A cool attribute of pointers is they can be initialized with the address of an existing variable. The only restriction is that it has to point to a legal data type. A double has to point to a double and not to an integer.int number;
int *pntr = &number;
As seen in this small snippet, a variable has to exist first before a pointer can point to it.
Pointer Variable Comparisons
It is possible to compare different pointers with each other. However, it is a simple relationship. The pointer variables are in memory at certain addresses. Addresses are linear. If one pointer variable comes before a second pointer variable then it is considered less than the second variable. In this way they are related to arrays.
The addresses are in hexadecimal format so the computer can compare them. If you are competent in number systems then you can too! However, I certainly am not. I forgot all that years ago because it was never necessary before. Not a big deal though. To make comparisons you can use any C++ relational operator.
Functions that use Pointers
Pointer variables can be used as function parameters. This concept lets the function modify the original variable.