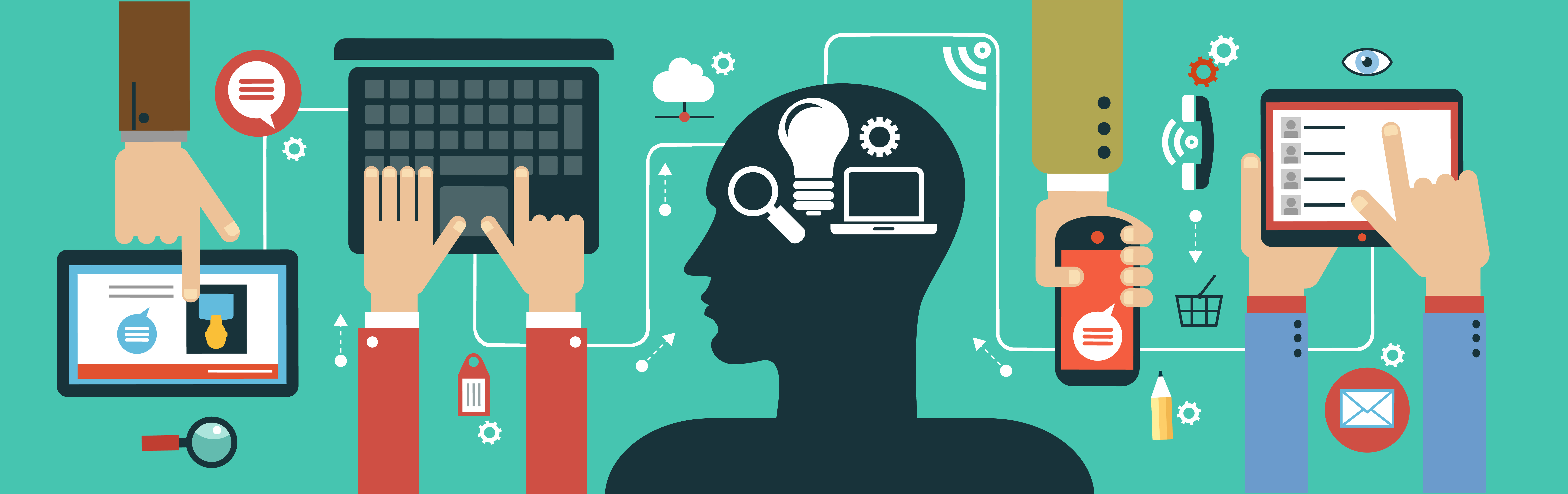
Creating Random Numbers in Python
This article is about creating random numbers in python.
This is my Networking book on Amazon, if you would take a look at it I would appreciate it.
Creating random numbers are one of the first tasks you learn to do. Well, they
are pseudo-random anyway. Base python includes a random function for us to get
started. You can create a text file to do this or use the python shell.random()
The pseudo-random generator is usually based off our system time because it is
easy to start like this. Let's start writing some code to see how it works.
# import required functions from the random module so we can use themfrom random import random, sample
# assign a random value to a variable and then print to consolevalue=random()
print(value)
# multiply the value by 10 and then cast it so it to an integervalue=int(value*10)
print(value)
# add a loopvalues=[]; i=0
while i < 20:
values.append(int(random()*10)+1)
i=i+1
print(values)
# assign random integersvalues=sample(range(1,100),20)
print(values)
The next step after you create software that does something is often packaging
it for distribution. You may have created a recipe app for your mother. You want
her to be able to use it, so you have to package it for the system she is
running. Most often this is Windows but we can do it for Mac and Linux as well.
There is a tool built for this which is "PyInstaller". It is not cross platform
so you built software for the platform you want.
You will need the "pip" tool installed also. This is very commonly used.
Use your package manager to install it.sudo dnf install pip -y
Once that is done we are ready to install "pyinstaller". Go to the directory
where you work in python and install from there.python -m pip install pyinstaller
Now type:pyinstaller -h
This lets you see all of the options., As you can see, you can fine tune your
packaging a lot. For Windows, I would suggest using these options: -scriptname,
--onefile, --add-binary, and --noconsole. There is a lot more we could go over
but is probably not applicable right now. For now, these options should get you
started.