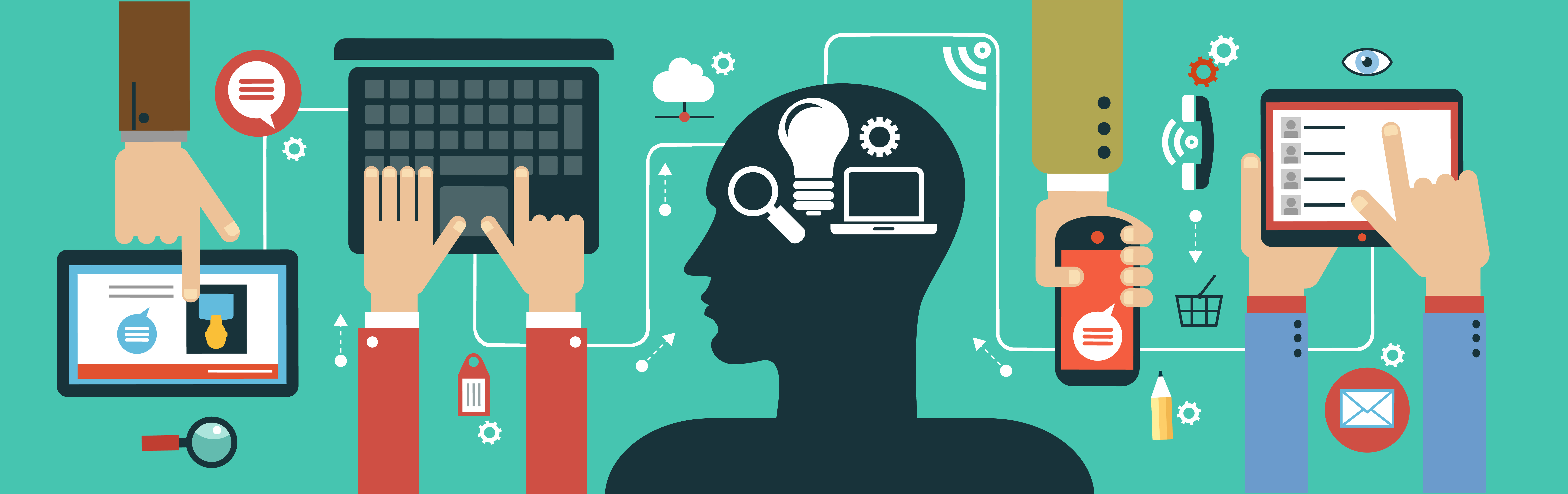
Local Scope In C++
Understanding the scope of your variables is key to avoiding undefined behavior. In this article, I will explain what it means to be in or out of scope.
Table of Contents
Introduction
Variables and parameters of a function are called local variables. The function can be “main” or or one that you created. If you define a variable in “main”, that variable can only be used in “main”. A variable created and defined in a function you create cannot be used in “main”.
Local Variables
Look at the following piece of code. The variable is local to the “main” function because it was created and defined there.
#include <iostream>
int main()
{
int num{}; // the variable “num” can only be used in the “main” function
return 0;
}
If we create a function and define a variable inside, that variable will be local and in scope to that function. It will not be visible to the “main” function.
#include <iostream>
int multiply( int a, int b)
{
return a * b;
}
int main()
{
std::cout << multiply(5,3);
return 0;
}
This program will output 15 as expected. The variables “a” and “b” are local to the “multiply” function. This means they are in scope to “multiply”. However, they are not accessible to the “main” function and are out of scope to “main”.
Variable Lifetime
The reason variables are out of scope between functions is they do not exist after their function runs. When a variable is defined in a function, it is destroyed at the end of that function. It can be the “main” function or “multiply”, it does not matter. To be exact, variables are destroyed at run time, not at compile time. It happens at the ending curly brace of the function it was defined in.
int multiply( int a, int b)
{
return a * b;
} // both variables “a” and “b” are destroyed here when the program gets to this ending curly brace
Local Scope
The scope of a variable determines whether it can be accessed. If it is in scope then it can be accessed. However, if it is out of scope, then it cannot be accessed. Scope is determined at compile time. A variable’s life begins at the point of creation and ends at the end of the function in which it is created.
Conclusion
In this section, we talked about local scope in C++. Local variables are local and in scope to the function they were created in. Variable lifetimes start when they are created and end at the last curly brace of the function they were created in. We then talked about the differences between being in scope and being out of scope.