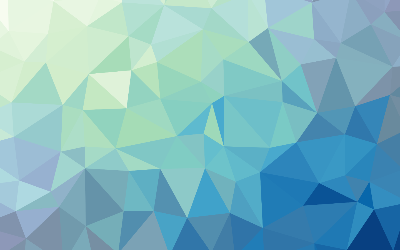
Doing Math in R
This is my guide on doing math in R.
Doing Math in R
Working in R means doing a lot of calculations. This is what R is for and why it is called a statistical programming language. You can do simple math and work with vectors. In fact, there are a few different categories. They are:
- Arithmetic
- Functions
- Vectors
- Matrixes
The arithmetic operators should be familiar to everyone. These are the basic math operators everyone learned when they were kids.
- \[x + y\] y added to x
- \[x - y\] y subtracted from x
- \[x * y\] x multiplied by y
- \[\frac{x}{y}\] x divided by y
- \[x ^ y\] x raised to the power of y
- \[x %% y\] remainder of x divided by y
- \[x% / %y\] x divided by y but rounded down
Let’s move to the next section, mathematical functions. These are the traditional algebraic functions and they work the same way.
- abs(x) takes the absolute value of x
- log x takes the logarithm of x with base y
- exp(x) returns the exponential of x
- sqrt(x) returns the square root of x
- factorial (x) returns the factorial of x!
- choose(x,y) returns the number of possible combinations when drawing y elements from x possibilities
You can take the log of a number like this:
Log 1.5
You can take the log of a series of numbers:
log(2:4)
That function takes the natural log of the numbers 2,3,4
You can specify a base:
log(2:4, base=4)
The other functions work similarly, I will get into them more when we need to.
You can round numbers easily in R. You just use the ‘round()’ function.
round(454567.2333445, digits=3)
Significant digits can be done just as easily.
signif(333.334455, digits=3)
Trig functions are also available. By default, R gives results in radians. So, if you need a result in degrees, you will have to convert it. I will show you how, though.
cos(120)
Gives results in radians
cos(95 * pi / 180)
Gives results in degrees
Working With Vectors
A vector is a one-dimensional set of values. It looks like this:
x=c(1,2,3,4,5,6,7,8)
They have to be the same type, such as integers.
There is a function, ‘str()’, that lets you look at any particular vector and see its properties. Use it like this:
str(x)
To see the length of a vector:
length(x)
Vectors can be several different types:
- Numeric
- Integer
- Logical
- Character
- Datetime
- Factors
You can test a vector to see what kind it is:
is.numeric(x)
is.character(x)
is.logical(x)
Those are all separate tests to determine what kind of vector you have. You will get the output of ‘true’ when you have a match.
To create vectors you can enter in numbers or use a sequence of numbers. I will show you how to do both. It is common to assign a variable to your data so it is easy to work on it. The variable is ‘x’.
x=c(22,33,44,55,66,77,88,99)
The ‘c’ is a function itself and combines the numbers in the parentheses to make a vector.
You can also use the colon operator to create a sequence of numbers.
x=c(2:7)
This creates a vector with the number 2,3,4,5,6,7
You can include negative numbers too.
x=c(7:-2)
R lets you combine vectors when you need to. If we have:
x=c(1:9)
y=c(11:16)
We can combine them like this:
total=c(x,y)
We can repeat vectors too. We do this with the ‘rep()’ function.
If we want to repeat a vector a set number of times, we do this:
rep(c(1:9), times=4)
When we want to repeat every value:
rep(c(1:9), each=3)
We can also tell R how often to repeat each value:
rep(c(1,9), times=c(3,4)
Looking At Vector Values
Once we have a vector, R lets us look at and work with individual values. The square brackets let us extract a value from the vector. We just indicate the position we want inside of the square brackets.
X[3]
This gives us the 3rd number from the start of the vector.
We can get more than one position value at once:
x[c(1,2,3)]
This will give us the first 3 positions of the vector.
You can change the value of a vector.
x=c(1,2,3,4,5)
Let us change the last value from 5 to 3
X[5] = 3
Now, our vector has been changed to what we want to reflect it as.
Making Copies of Vectors
Before working with an important vector set of data, make a copy of it. You do not want to accidentally change it without knowing it. Do it like this:
X.copy = x
Now you can do your work with a little less worry.
Comparing Values
To compare values in a vector:
X > 5
This gives us logical values. Any time there is a value greater than 5, the output is true.
We can also check positions that are greater than 5.
which(x > 5)
This shows us which positions in the vector are greater than 5.
These are the logical operators in R:
- X == y
- X != y
- X > y
- X >= y
- X < y
- X <= y
- X & y
- X | y
- !x
- xor(x,y)
More Arithmetic Operations
Once we have a vector set up and kind of know how it works, we can start doing more with it. I recently took a statistics class and I used many of these functions to great effect. It really speeds things up. The idea of a vector is to look at each value in a vector and do something with it. That is what functions do once we have a vector set up. Here are the arithmetic functions that are pretty useful:
- sum(x) calculates the sum of values in the vector x
- prod(x) calculates the product of all the values in the vector x
- min(x) gives the minimum of all values in x
- max(x) gives the max of all the values in the vector x
- cumsum(x) gives the cumulative sum of all the values in the vector x
- cumprod(x) gives the cumulative product of all the values in the vector x
- cumin(x) gives the minimum for all values in x from the start of the vector to the position indicated
- cummax(x) gives the maximum for all values in x from the start of the vector until the position indicated
- diff(x) gives for every value the difference between that value and the next value in the vector