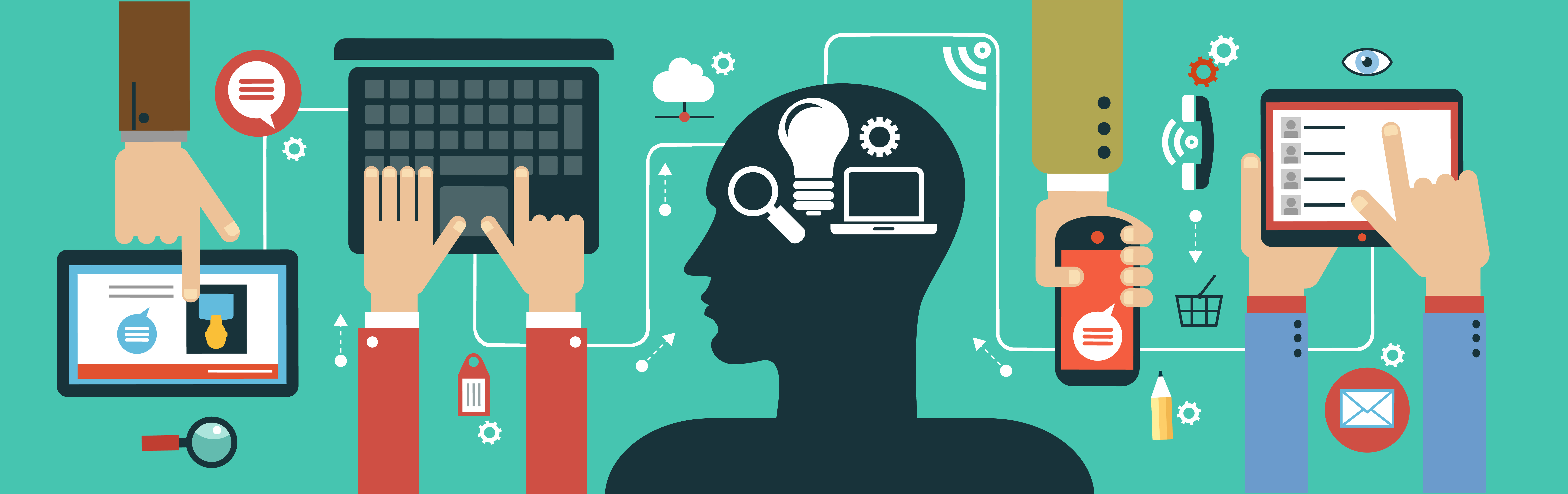
Naming Collisions in C++
These are my notes and thoughts about naming collisions in C++.
A naming collision happens when there is an identifier that is not unique. Why might this happen? You could use the same name in two different parts of your program. There could be multiple files that need compiling which have the same name. The linker would give an error in this case. Also, future versions of C++ might have a new name for an identifier that breaks an existing program.
If this happens, the compiler will give an error. If your project contains thousands of lines, good luck figuring out where everything is.
This problem happens with variables and functions. With variables, you have global and local scopes. You can prevent a lot of issues by using local scope variables. However, we do not get this luxury with functions. Either of these can cause a naming collision.
This leads us to namespaces. Namespaces were created so that we can use the same name identifier, or at least so they would not break a project. There is the standard namespace and we can also create our own namespaces. The advantage to a namespace is that anything inside one will not cause errors with one outside of it. It is a good idea to create your own namespace and include your functions in it. When you then refer to that code inside that particular namespace, it will not cause any naming collisions or conflicts.
The global namespace contains anything that is not already part of another function, class, or another namespace. A global variable would be an example of this. This is an important reason to avoid global variables if you can. For example, any variable outside of the main function is a global variable.
#include <iostream>
int num{};
double x{};
int main()
{
return 0;
}
The variables declared above the main function are global variables. If you have another file that has the same name that is in the global namespace, you will have an error and your project will not compile.
The standard namespace was created to address this issue. All the original functions and identifiers were in the global namespace. However, this caused issues as it was easy to have naming collisions from other files or libraries. So, they were all moved to the standard namespace. So, when we want to use a function from that namespace, we have to designate it.
std::cin
That is using the cin function inside the standard namespace. This is the proper way to do it.
#include <iostream>
int main()
{
std::cout << “I live in the standard namespace”;
return 0;
}
Try not to use using-directives as there is no good purpose for most people.